Question
This lab is designed as a basic recursion overview. By the end of it, you should have enough recursive programming experience to have an elementary
This lab is designed as a basic recursion overview. By the end of it, you should have enough recursive programming experience to have an elementary understanding of the recursive problem-solving process. This process will be built upon in future assignments, as well as in future classes.
Using eclipse!
Problem:
For this lab, you will write a class called RecursionToolkit. In it are a number of recursive methods. You will then use a main method to test your code with the provided test information. If you are particularly savvy, you could write these as JUnit Test Cases (and will get a bit of extra credit as thanks for making my job easier). The methods, and their test cases, follow: (1) Harmonic Series
Write a method to calculate the nth term of the Harmonic Series. The series is defined as:
As an argument, your method should take n, corresponding to which nth term of the series the user wants. The return value is the result of the series up to that n. If the number is zero or negative, the result should be the integer 1 as a way of error handling. Examples:
harmSer(5) should return 2.283333333333333
harmSer(2) should return 1.5
harmSer(20) should return 3.597739657143682
harmSer(-5) should return 1
(2) Sum Digits
Write a method to sum all the digits in an integer. Return 0 if the input is negative.
sumDigits(1234) should return 10
sumDigits(9) should return 9
sumDigits(5032134) should return 18
sumDigits(-50) should return 0
(3) Recursive Sequential Search
Write a method to recursively search an array of Strings for a given target, using sequential search. Each call is only concerned with searching the current element otherwise, a recursive call (or return) should be made. Return a -1 if the element is not found, and the index of the element if the element is found
Searching the array {Apple, Orange, Grapefruit, Pear} for Orange, should return the integer 1. Searching for Pineapple, should return -1
(4) String Cleaning
Write a method for which, given a String, will clean redundant characters. That, characters that are the same and are neighbors should be reduced to one character.
For example, using the String yyzzza as an argument should return yza and Hello should return Helo.
co n= 1 + 1 + 1 + 1 n=1 14 1-3 1-2 1-n 8>Step by Step Solution
There are 3 Steps involved in it
Step: 1
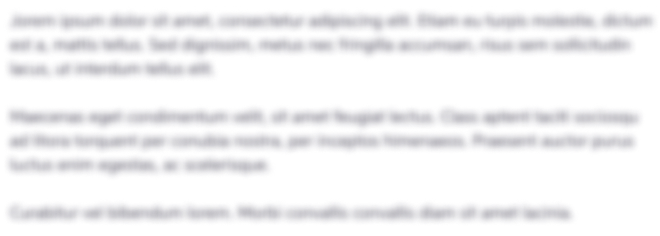
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started