Question
This lab practices defining and implementing class, and working on an array of objects of the class type. Requirement Modify the rational class from lab6
This lab practices defining and implementing class, and working on an array of objects of the class type.
Requirement
Modify the rational class from lab6 as follows (a sample solution is here SEE Below FOR RATIONAL CLASS THAT MUST BE USED)):
Merge the three constructors into one, by using default values for parameters (recall the example we have seen, Box class below.).
class Box { public: // Constructor definition Box(double l=2.0, double b=2.0, double h=2.0) { length = l; breadth = b; height = h; } // more functions omitted private: int length; int breadth; int height; }; int main() { Box box1; // this will call the above constructor with all parameters set to // default values, i.e., 2.0, 2.0, 2.0 Box box2(4.5); // this will call with 4.5, 2.0, 2.0 Box box3 (3.4, 2.2); // this will use 3.4 for l, 2.2 for b, and 2.0 for h Box box4 (1.0, 1.0, 10.0); // this will give us a tall box ! l=1.0, b=1.0, h=10.0 }
add a set function that sets both numerator and denominator of the invoking object to the values provided in parameter:
rational a; a.set (1,100); // this set a to be 1/100
For all parameters that are of rational type, use pass-by-reference (to avoid copying the whole object when passing parameters). If the function is not supposed to modify the paramter, use const before the parameter type.
Follow the example of Sum function to declare and implement a Product function which sets the invoking object to be the product of two rational numbers.
Extra credit: declare and implement a private function called simplify that simplfies the invoking object, for example, if the invoking object is stored as 4/12, the function will simplify it to be 1/3.
Hint: you will need to find the gcd(greatest common divisor) of the numerator and denominator, and then update them by dividing them by the gcd.
//EZ: return the gcd of numbers a and b int gcd (int a, int b) { while (a!=0 && b!=0) { a = a % b; if (a!=0) b = b % a; } if (a==0) return b; if (b==0) return a; }
And then, in all the functions that modify the invoking object's value, call simplify function in the very end to simplfiy its value before returning from the function.
Modify your main function, so that in addition to the original test code, your program should also:
Prompt the user to enter the number of terms to add and multiply,
Dynamically allocate an array rational objects with the above entered size
rational * fractions = new rational[seq_length]; // suppose you use int seq_length to store the input from step 1
call set function to set each of these objects to be 1/1, 1/2, 1/3, 1/4, ... . You can access the above array the usual way.
fractions[4].set (1,4); // This will set the 4th object to 1/4.
calculate the sum (as a rational number) of all these 10 rational numbers, display the sum, i.e.,
// report the value of 1/1 + 1/2 + 1/3 + 1/4 +... as a ratioanl number //You are required to call the Sum member function multiple times to find out the sum
calculate the product (as a retioanl number) of all these rational numbers, display the product
// report the value of 1/1 * 1/2 * 1/3 *... as a ratioanl number // Note: You are required to call the Product member function multiple times to find out the product
Rational Class:
/* Todo: Please remove this comment after you have completed the job of * separating the files. Please open at least three terminal windowss: one for viewing lab6_sol.cpp, one for editing the new files, and one for later usage. Before you go on, learn how to copy and paste lines from a file to another file. We will practice separating lab6_sol.cpp into three files: 1. rational7.h: header file that define the class rational cut and paste the definition of the class rational into this file 2. lab7.cpp: driver program that tests the class rational, this file contains your main function... In addition to the usual header files you include, add the following: #include "rational7.h" This allows the compiler to knows about rational class: 3. rational7.cpp: implementation file for class rational cut and paste the implementation of ALL member functions of class rational into this file It also needs the following line: #include "rational7.h" Compile your code with all sources: 1. You can use a single command to compile all .cpp files, and link them together into a.out, as follows: g++ rational7.cpp lab7.cpp 2. Or you can compile each .cpp file separately, and then link them together: g++ -c rational7.cpp ## the above command just compile rational7.cpp to object code, ## rational7.o g++ -c lab7.cpp ## the above command just compile main.o to object code, ## main.o g++ rational7.o main.o ## link *.o into one executable a.out Finally: Add a new member function to your rational class, that tests whether the invoking object has the same value as another object (passed by reference, as a parameter). Modify both rational7.cpp and rational7.h And then call this function from lab7.cpp... */ /* This rational class practice basics of defining and using class. */ #include#include using namespace std; class rational { public: /* default constructor set the rational number to 0, (i.e., numerator is 0, denominator is 1) */ rational (int p=0, int q=1); /* Set the invoking object's value from user input */ void input(); /* Display invoking object's value in the standard output, in the form of numerator/denominator */ void output(); //return the invoking object's numerator int get_numerator(); //return the invoking object's denominator int get_denominator(); // Set invoking object to be the sum of op1 and op2 void Sum (rational op1, rational op2); static int counter; private: int numerator; int denominator; }; int rational::counter=0; int main () { char answer; // Test the three constructors ... rational a(1,2); // a is 1/2 cout <<"rational object initialized with parameter 1,2:"; a.output (); cout < > answer; } while (answer=='y' || answer=='Y'); //test getters cout <<" C's numerator is: " << c.get_numerator() << endl; cout <<" C's denominator is: " << c.get_denominator() << endl; } //Definitions of all member functions rational::rational (int p, int q) { if (q==0) exit(1); numerator = p; denominator = q; counter++; cout <<"So far " < > top >> c >> bottom; if (c!='/' || bottom==0) { cout <<"wrong input. Enter a rational (p/q):"; valid = false; } else valid = true; } while (!valid); // set the invoking object's numerator, denominator numerator = top; denominator = bottom; } /* Display invoking object's value in the standard output, in the form of numerator/denominator */ void rational::output() { cout << numerator << "/" << denominator; } //return the invoking object's numerator int rational::get_numerator() { return numerator; } //return the invoking object's denominator int rational::get_denominator() { return denominator; } // Set invoking object to be the sum of op1 and op2 void rational::Sum (rational op1, rational op2) { numerator = (op1.numerator * op2.denominator + op2.numerator*op1.denominator); denominator = op1.denominator*op2.denominator; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
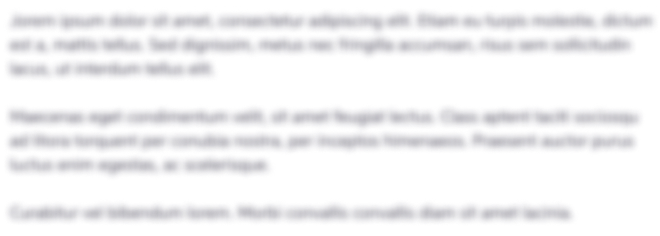
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started