Answered step by step
Verified Expert Solution
Question
1 Approved Answer
This lab will take all of the work you have previous done and connect it together to make a calculator. You will be using the
This lab will take all of the work you have previous done and connect it together to make a calculator. You will be using the expresssionStream from Lab 1 to parse an input expression and calculate that expressoin. You will be implementing the Shunting yard algorithm to perform your calculation. This algorithm uses both Last In/First out (Stack) and First In/First Out (queue) to accomplish the calculation. This means that in order to get your calculator working, you need to have lab 2 and lab 3 working properly. ### Lab Instructions Implement each of the functions to perform the necessary actions outlined in the `.h` files. As you are writing your functions, read the instructions and think of how you would test that functions while you are writing it. Write your Test first and then implement your functions. Try running your test and then fixing your issues. You only need to support integer calculations. If you feel like supporting floating point calculations, you might need to change the way expressionStream works. You will also need to write a new calculate function that returns a float rather than an integer. You may need to create auxiliary functions to complete tasks, or to avoid copy and pasting repetitive code. Do not make these class functions. These should only appear in the .cpp file ### Hints ### - Some of these functions are basically the same. Don't rewrite or paste code, just call the function with the altered inputs. - Keep track of your edge cases: empty, 1 item, 2 items
#ifndef CMPE126S18_LABS_CALCULATOR_H #define CMPE126S18_LABS_CALCULATOR_H #include "lifo.h" #include "fifo.h" #include "expressionstream.h" namespace lab4{ class calculator{ lab3::fifo infix_expression; lab3::fifo postfix_expression; void parse_to_infix(std::string &input_expression); //PRIVATE function used for converting input string into void convert_to_postfix(lab3::fifo infix_expression); //PRIVATE function used for converting infix FIFO to postfix public: calculator(); //Default constructor calculator(std::string &input_expression); // Constructor that converts input_expression to infix and postfix upon creation friend std::istream& operator>>(std::istream& stream, calculator& RHS); //Store the infix and postfix expression in calculator int calculate(); //Return the calculation of the postfix expression friend std::ostream& operator<<(std::ostream& stream, calculator& RHS); //Stream out overload. Should return in the format "Infix: #,#,#,# Postfix: #,#,#,#" }; }
#include "lifo.h" namespace lab3 { lifo::lifo() { lifo_storage.reserve(100); index = -1;//Reserve 100 spaces in lifo_storage } lifo::lifo(std::string input_string) { lifo_storage.reserve(100); lifo_storage.append(input_string); index = 0; } lifo::lifo(const lifo &original) { lifo_storage.reserve(100); this->index = original.index; } lifo::~lifo() { index = -1; } lifo &lifo::operator=(const lifo &right) { //return <#initializer#>; lifo_storage.reserve(right.lifo_storage.capacity()); index = right.index; for (int i = 0; i <= index; i++) { lifo_storage[i] = right.lifo_storage[i]; } return *this; } bool lifo::is_empty() const { if (index == -1 ||) { return true; } else { return false; } //return false; } unsigned lifo::size() const { int temp; if (index > -1) { for (int i = 0; i <= index; i++) { temp++; } } else { temp = 0; } return temp; } std::string lifo::top() const { return lifo_storage[index]; //return std::__cxx11::string(); } void lifo::push(std::string input) { if (index == lifo_storage.capacity() - 1) { lifo_storage.reserve(lifo_storage.capacity() + 20); } lifo_storage[++index] = input; } void lifo::pop() { if (index > -1) index--; } }
#include "fifo.h" namespace lab3{ fifo::fifo() { lab2::stringVector fifo_storage; fifo_storage.reserve(100); front_index=0; back_index=0; //Reserve 100 spaces in fifo_storage } fifo::fifo(std::string input_string) { fifo_storage[0]=input_string; fifo_storage.reserve(100); front_index=0; back_index=0; } fifo::fifo(const fifo &original) { fifo_storage.reserve(100); this->fifo_storage=original.fifo_storage; this->front_index=original.front_index; this->back_index=original.back_index; } fifo::~fifo() { front_index=-1; back_index=-1; } fifo &fifo::operator=(const fifo &right) { if (&right == this){ return (*this); } fifo_storage.reserve(100); this->front_index = right.front_index; this->back_index = right.back_index; } bool fifo::is_empty() const { //return false; if(front_index == -1 || front_index==back_index){ return true; } return false; //return false; } unsigned fifo::size() const { unsigned int temp; if(front_indexelse{ //If back index < front index then the list has wrapped around temp = (100-front_index)+back_index+1; //fifo_storave.size() - front index = how many until end. //Adding the back index +1 should be how many items are in that section } return (temp); } std::string fifo::top() const { //return std::__cxx11::string(); return fifo_storage[front_index]; } void fifo::enqueue(std::string input) { back_index++; fifo_storage[back_index]=input; if(fifo_storage.capacity()<=back_index){ fifo_storage.reserve(fifo_storage.capacity()+20); } } void fifo::dequeue() { if(!is_empty()) {// In queue you would always take from the front, so you need to make sure that the fifo storage isnt empty ++front_index; } else{ throw"ERROR, out of bounds"; } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
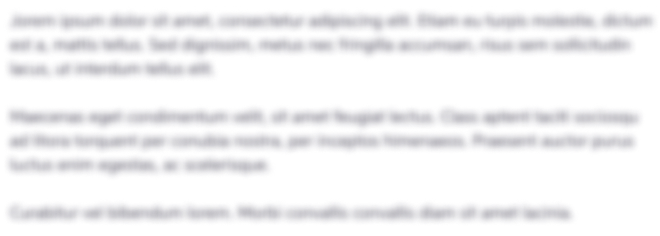
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started