Question
This problem asks you to create a custom data type to handle large integer arithmetic, by creating a custom C++ class called Hugeint. C++ has
This problem asks you to create a custom data type to handle large integer arithmetic, by creating a custom C++ class called Hugeint. C++ has a number of types to handle integer data, including ints, short ints and long ints, and their signed and unsigned variants. However, the maximum positive number that one can store in the largest of these, which is an unsigned long int, is 18,446,744,073,709,551,615. This number, as you can see, has 20 digits. In many scientific and engineering applications, this does not suffice to hold values required for complex computations. Anything about 1020 would be too big to hold in the unsigned long int type. So your task in this assignment is to create a C++ class called Hugeint which will allow basic arithmetic operations on positive integer values.
Can't use vectors, or maps, and limited to only using string, cmath, iostream, sstream, and iomanip headers. You do not need to worry about the user inputting negative or incorrect values, you can assume the user will input only valid data.
Here is the header file for the Hugeint class, the CPP file must match this:
class Hugeint
{
public:
Hugeint();
Hugeint(std::string);
Hugeint(const Hugeint &);
Hugeint(Hugeint &&);
Hugeint & operator = (const Hugeint &);
Hugeint & operator = (Hugeint &&);
~Hugeint();
friend std::ostream & operator << (std::ostream &, const Hugeint &);
friend std::istream & operator >> (std::istream &, Hugeint &);
friend bool operator < (const Hugeint &, const Hugeint &);
friend bool operator == (const Hugeint &, const Hugeint &);
friend bool operator != (const Hugeint & lhs, const Hugeint & rhs) { return !(lhs == rhs); }
Hugeint & operator ++ ();
Hugeint operator ++ (int);
Hugeint operator + (Hugeint) const;
Hugeint operator * (const Hugeint & lhs, const Hugeint & rhs);
Hugeint operator / (const Hugeint & lhs, const Hugeint & rhs);
private:
std::string value;
std::string add(std::string, std::string) const;
};
Step by Step Solution
There are 3 Steps involved in it
Step: 1
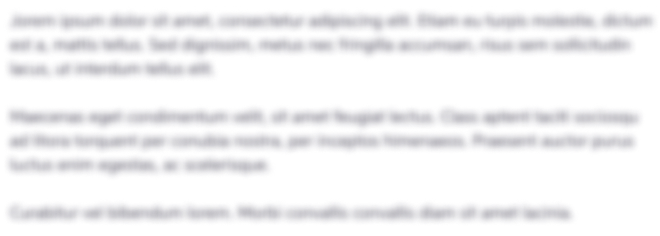
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started