Question
This program is running the functions too many times. When you run a function save the result into a variable, then pass that variable into
This program is running the functions too many times. When you run a function save the result into a variable, then pass that variable into the other functions as input parameters.
Can you fix it like the comment above?
"use strict";
// Use document.getElementById to read the prices of the 3 elements from the DOM: back-price, calc-price, and text-price.
function showItems() {
const backPrice = document.getElementById("back-price");
const calcPrice = document.getElementById("calc-price");
const textPrice = document.getElementById("text-price");
// Use the textContent property of the elements to read the text contents from the 3 DOM elements.
let backpackPrice = backPrice.textContent;
let calculatorPrice = calcPrice.textContent;
let textbookPrice = textPrice.textContent;
let pricestax = [backpackPrice, calculatorPrice, textbookPrice,];
return pricestax;
}
// Cal total items price
function calcSum() {
let subTotal = 0;
subTotal = Number(showItems()[0]) + Number(showItems()[1]) + Number(showItems()[2]);
return subTotal;
}
// Declare tax rate
function taxRate() {
const taxRate = 0.13; // The bookstore charges 13% sales tax.
return taxRate;
}
// Cal sale tax
function calcTax() {
let taxAmount = 0;
taxAmount = calcSum() * taxRate();
return taxAmount;
}
// Cal final cost
function calcfinalCost() {
let finalcostAmount = 0;
let sumitemsAmout = calcSum();
let taxrateAmount = calcTax();
finalcostAmount = calcSum() + calcTax();
//Output the results
document.getElementById("sub-total").textContent = sumitemsAmout.toFixed(2);
document.getElementById("tax-amount").textContent = taxrateAmount.toFixed(2);
document.getElementById("total").textContent = finalcostAmount.toFixed(2);
}
calcfinalCost();
Step by Step Solution
There are 3 Steps involved in it
Step: 1
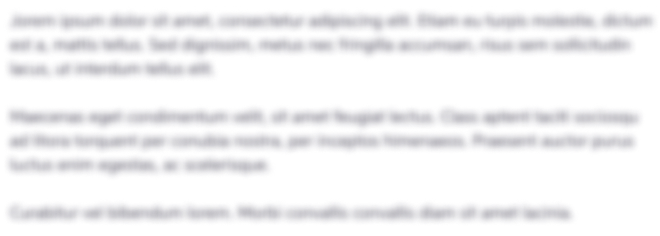
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started