Question
This program must be able to compile in Command prompt Note: In this assignment, only, you may use magic numbers (literal constants in the body
This program must be able to compile in Command prompt
Note:
In this assignment, only, you may use "magic numbers"
(literal constants in the body of a method) in the main program, only.
Do not use magic numbers anywhere in class Car.
In this program there is no need to use a debug print switch.
Remember to follow all programming guide lines as detailed
in Coding Standard Handout.
Write a main class CarLot, stored in a file named CarLot.java, and
a subsidiary class Car, stored in a file named Car.java .
The instance variables of a Car object are
private String make;
private int year;
private double price;
These instance variables are, as shown in Chapter 8 and in lecture,
stored "at class level." This means they are stored after the statement
public class Car
{
and before the beginning of the constructor.
Also at class level in class Car there should be the statement
public static DecimalFormat twoDecPl = new DecimalFormat("$0.00");
The purpose of making this DecimalFormat object "static"
is so that only one copy of it will be stored.
If it were not static, one copy of it would be stored in each object of
class Car. The purpose of making this DecimalFormat object public is so
that the main program will also be able to access the object.
At the top of the Car class you will need
import java.text.DecimalFormat;
The Car class shall contain
a constructor
an accessor method getMake
an accessor method getYear
an accessor method getPrice
a mutator method setPrice
an equals method
a toString method
These methods are of the forms that have been shown in
lecture and that are shown in Chapter 8.
The toString method must return a String that will print out as
Description of vehicle:
Make : Ford
Year : 1997
Price: $7300.00
This String should start with " " so that "Description..."
will automatically start on a new line without the user having
to worry about that.
This is only an example, of course; your toString must return a String
appropriate to the values of the three instance variables, whatever
those values may be.
Use the DecimalFormat object twoDecPl to format the price in toString.
Main program
------------
1) Declare four objects of type Car: car1, car2, car3, and car4.
2) Instantiate the object car1, with a make of Ford, a year of 2006,
and a price of $23000.
Print out this object, invoking the toString method.
Print a label "car1..." immediately before the description of car1,
as shown in the Sample Output below.
This can be done using the statement
System.out.println(" car1..." + car1);
in main.
3) Use the three accessor methods to retrieve and print the make, year,
and price of car1, as shown in the Sample Output below.
You must actually use the accessor methods, not just print out the
values of these three attributes as constants or local variables!
When printing the price in the main program, use the DecimalFormat object
from class Car:
Car.twoDecPl.format(...)
where ... is the price of the car.
Because twoDecPl is a public static object,
this will work if done correctly.
4) Instantiate car2 as a new object of class Car, with the same attributes
(the same values of the instance variables) as car1.
Print out this object, preceded by a label "car2..." as shown
in the Sample Output below.
5) Compare car1 to car2 using the == operator, and print the result.
Comparing two objects is, as you know, not a good idea and can give
incorrect results. Explain the results you got, in comments
at that point in your program.
5) Compare car1 to car2 using the .equals method of class Car,
and print the result.
6) Instantiate the object car3, having make equal to Honda,
year equal to 2003, and a price of $9000.
Print the description of car3 using the toString method.
7) Set car4 = car3;
Print the descriptions of car3 and car4.
8) Use the mutator method setPrice to reset the price of car4 to
$8695.
9) Print out car3 and car4.
Note that the price of car3 has been changed. Why is this?
Explain the answer in comments in your program at that point.
In this assignment, you do not need a debug print switch.
Sample Input: None
--------------------
Sample Output:
--------------
car1...
Description of car:
Make : Ford
Year : 2006
Price: $23000.00
Make of car1 using getMake = Ford
Year of car1 using getYear = 2006
Price of car1 using getPrice = $23000.00
car2...
Description of car:
Make : Ford
Year : 2006
Price: $23000.00
car1 and car2, printed above, are not equal when compared using == .
car1 and car2, printed above, are equal when compared using .equals().
car3...
Description of car:
Make : Honda
Year : 2003
Price: $9000.00
Set car4 = car3;
car3...
Description of car:
Make : Honda
Year : 2003
Price: $9000.00
car4...
Description of car:
Make : Honda
Year : 2003
Price: $9000.00
Reset car4.setPrice(8695.0);
car3...
Description of car:
Make : Honda
Year : 2003
Price: $8695.00
car4...
Description of car:
Make : Honda
Year : 2003
Price: $8695.00
Submit your Java source file, CarLot.java and Car.java
Step by Step Solution
There are 3 Steps involved in it
Step: 1
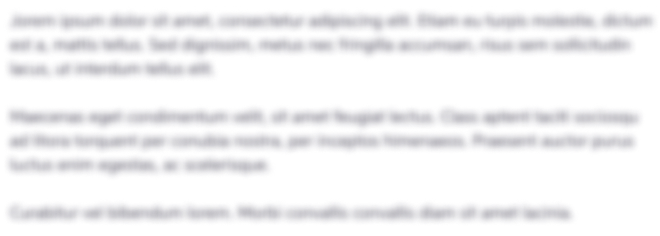
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started