Question
This program takes inputs for adding to a bag, removing from a bag, displaying the bag, and exits the program. Whenever the user inputs to
This program takes inputs for adding to a bag, removing from a bag, displaying the bag, and exits the program. Whenever the user inputs to add something into the bag, the bag will return an integer, which is the index that the item has been stored in.
Sample output:
Removing an item from the bag takes the integer (index) as the argument, and removes it from the bag.
Sample output:
However, when the user wants to remove something from the bag that has more than one object, and another object has a higher index than the item that's removed, the items that have a higher index than the item that got removed will shift down one index to fill-in the spot. How would I be able to fix this so that those items keep the index that they were stored in, without shifting everything down?
Example:
Code:
// main.cpp:
#include
void insert(ArrayBag vector void remove(ArrayBag void display(ArrayBag int numberOfEntries = (int)bagItems.size(); for (int i = 0; i void bagFunctions(ArrayBag int const bagCapacity = 6; // Assuming that the bag will be 6 throughout the project. string items[bagCapacity]; bool inUse[bagCapacity]; cout cin >> userInputNumerical; if (userInputNumerical == 1) { if (bag.getCurrentSize() == 6) { cout > addToBag; insert(bag, addToBag, items, inUse); } } if (userInputNumerical == 2) { if (bag.isEmpty()) { cout > removeFromBag; remove(bag, removeFromBag); display(bag); } } if (userInputNumerical == 3) { display(bag); } if (userInputNumerical == 4) { exit = true; } if (exit) { // Program is complete. } else { bagFunctions(bag); } } int main() { ArrayBag --------------- // ArrayBag.cpp: template template template return itemCount; } template return itemCount == 0; } template int emptyIndex = getNextEmptyIndex(); if (emptyIndex >= 0 && (itemCount template ItemType removedObject; if (inUse[receipt]) { removedObject = items[receipt]; items[receipt] = items[itemCount - 1]; items[itemCount - 1] = ""; inUse[itemCount - 1] = false; --itemCount; } return removedObject; } template itemCount = 0; } template bool found = false; int curIndex = 0; while (!found && curIndex return found; } template int frequency = 0; int curIndex = 0; while (curIndex return frequency; } template vector for (int i = 0; i return bagContents; } template bool found = false; int result = -1; int searchIndex = 0; while (!found && searchIndex return result; } template template ------------- // ArrayBag.h: template /** Number of items in this bag. */ int itemCount; /** Maximum capacity of this bag. */ int maxItems; /** Data storage. */ ItemType items[DEFAULT_CAPACITY]; //ItemType* items = new ItemType[DEFAULT_CAPACITY]; bool inUse[DEFAULT_CAPACITY]; int getIndexOf(const ItemType& target) const; public: ArrayBag(); ~ArrayBag(); int getCurrentSize() const; bool isEmpty() const; int add(const ItemType& newEntry); ItemType remove(const int receipt); void clear(); int getFrequencyOf(const ItemType& anEntry) const; bool contains(const ItemType& anEntry) const; vector int getNextEmptyIndex(); void printInUse(); }; #include "ArrayBag.cpp" #endif -------------- // BagInterface.h: #ifndef _BAG_INTERFACE #define _BAG_INTERFACE #include using namespace std; template virtual ~BagInterface() {} virtual int getCurrentSize() const = 0; virtual bool isEmpty() const = 0; virtual int add(const ItemType& newEntry) = 0; virtual ItemType remove(const int receipt) = 0; virtual void clear() = 0; virtual int getFrequencyOf(const ItemType& anEntry) const = 0; virtual bool contains(const ItemType& anEntry) const = 0; virtual vector virtual int getNextEmptyIndex() = 0; virtual void printInUse() = 0; }; #endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
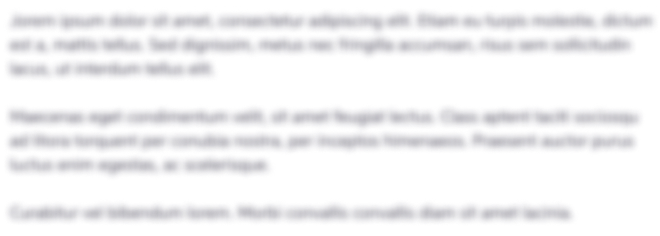
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started