Answered step by step
Verified Expert Solution
Question
1 Approved Answer
#This program was created by Huai Liu in 2021 #It is for Assignment 2 of Software Quality and Testing (COS80022) #It is used by Customers
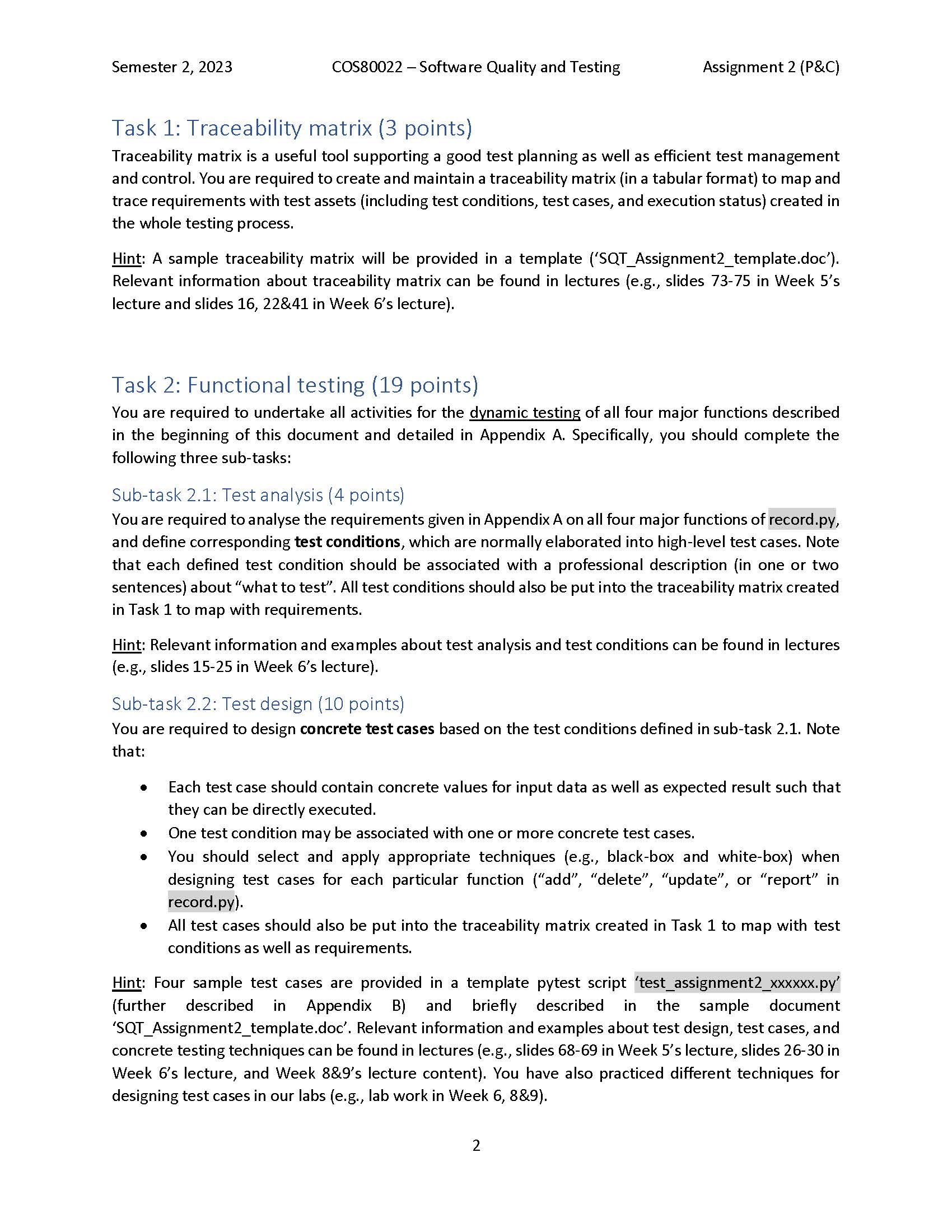
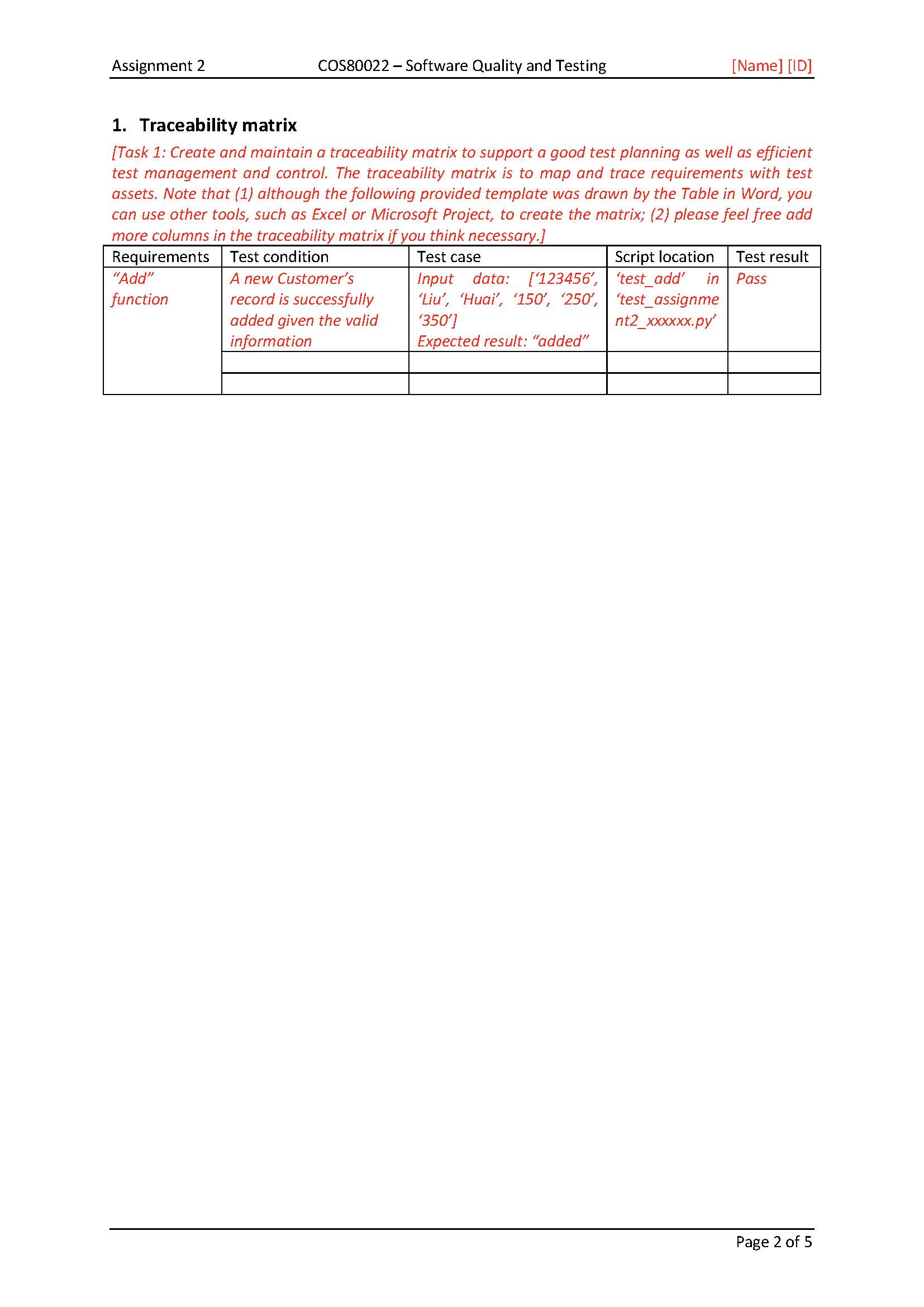
#It is for Assignment 2 of Software Quality and Testing (COS80022)
#It is used by Customers in the above unit at Swinburne University
import csv
import sys
#This module checks whether a row provides valid inputs
#It will be used by the "add" and "update" functions
def checkValidity(sRecord):
if not (sRecord[0].isdecimal() and len(sRecord[0]) == 6):
print("You should provide six digits as Customer ID")
return "invalid ID"
if not (sRecord[1].isalpha() and len(sRecord[1]) > 1 and len(sRecord[1]) < 36):
print("The surname should only contain 2 to 35 letters")
return "invalid surname"
if not (sRecord[2].isalpha() and len(sRecord[2]) > 1 and len(sRecord[2]) < 36):
print("The given name should only contain 2 to 35 letters")
return "invalid given name"
if not (sRecord[3].isdecimal() and int(sRecord[3]) >= 0 and int(sRecord[3]) <= 300):
print("The expense for the mouse should be an integer between 0 and 300")
return "invalid expense for the mouse"
if not (sRecord[4].isdecimal() and int(sRecord[4]) >= 0 and int(sRecord[4]) <= 300):
print("TThe expense for the bag should be an integer between 0 and 300")
return "invalid expense for the bag"
if not (sRecord[5].isdecimal() and int(sRecord[5]) >= 0 and int(sRecord[5]) <= 400):
print("The expense for the lamp should be an integer between 0 and 400")
return "invalid expense for the lamp"
return "valid"
#This module checks whether the input ID exists in the list
#It will be used by all four major functions
def checkExist(filename, ID):
with open(filename, newline='') as file:
rows = csv.reader(file)
for row in rows:
if(row[0] == ID):
return True
return False
#This module implements the "add" function
def addCustomer(filename, CustomerRecord):
#If the provided input is invalid or there already exists the Customer ID, no addition will be done
validity = checkValidity(CustomerRecord)
if validity != "valid":
return "not added"
if checkExist(filename, CustomerRecord[0]):
return "not added"
#The following adds a Customer's information into the file
with open(filename, 'a', newline='') as file:
writeOp = csv.writer(file)
writeOp.writerow(CustomerRecord)
return "added"
#This module implements the "delete" function
def deleteCustomer(filename, deletedID):
#If the given Customer ID does not exist, no deletion will be done
if not checkExist(filename, deletedID):
return "not exist"
#The followings delete the record of the Customer with the ID
lines = list()
with open(filename, newline='') as file:
rows = csv.reader(file)
for row in rows:
if row[0] == deletedID:
continue
else:
lines.append(row)
with open(filename, 'w', newline='') as file:
writeOp = csv.writer(file)
writeOp.writerows(lines)
return "deleted"
#This module implements the "update" function
def updateCustomer(filename, updatedLine):
#If the provided input is invalid or the given ID does not exist, no updating will be done
validity = checkValidity(updatedLine)
if validity != "valid":
return "not updated"
if not checkExist(filename, updatedLine[0]):
return "not updated"
#The following updates the record of the Customer with the ID
lines = list()
with open(filename, newline='') as file:
rows = csv.reader(file)
for row in rows:
if row[0] == updatedLine[0]:
lines.append(updatedLine)
else:
lines.append(row)
with open(filename, 'w', newline='') as file:
writeOp = csv.writer(file)
writeOp.writerows(lines)
return("updated")
#This module implements the "report" function
def reportCustomer(filename, reportedID):
#If the given Customer ID does not exist, no reporting can be given
if not checkExist(filename, reportedID):
return "not exist"
#The following reports the final expense and the profit evaluation of the Customer with the ID
with open(filename, newline='') as file:
rows = csv.reader(file)
for row in rows:
if row[0] == reportedID:
reportedLine = row
break
#Get the individual costs
costMouse = int(reportedLine[3])
costBag = int(reportedLine[4])
costLamp = int(reportedLine[5])
#Calculate the final mark
total = costMouse + costBag + costLamp
#Decide the evaluation
if total >= 800:
evaluation = 'Loss'
else:
if total > 500:
evaluation = 'Low Profit'
else:
evaluation = 'High Profit'
return [total, evaluation]
#We must use the file "list.csv"
filename = 'list.csv'
#The program requires some parameters
if len(sys.argv) == 1:
print("You should indicate what function to implement")
exit(0)
#The "add" function requires 6 parameters: ID, surname, given name, and costs for the mouse, the bag and the lamp
if sys.argv[1] == 'add':
if(len(sys.argv) < 8):
print("You should provide comprehensive Customer information to be added")
exit(0)
else:
newCustomer = [sys.argv[2], sys.argv[3], sys.argv[4], sys.argv[5], sys.argv[6], sys.argv[7]]
result = addCustomer(filename, newCustomer)
print(result)
exit(1)
#The "delete" function requires 1 parameter: ID
if sys.argv[1] == "delete":
if(len(sys.argv) < 3):
print("You should provide Customer ID to be deleted")
exit(0)
else:
deletedID = sys.argv[2]
result = deleteCustomer(filename, deletedID)
print(result)
exit(1)
#The "update" function requires 6 parameters: ID, surname, given name, and costs for the mouse, the bag and the lamp
if sys.argv[1] == "update":
if(len(sys.argv) < 8):
print("You should provide comprehensive Customer information to be updated")
exit(0)
else:
updatedLine = [sys.argv[2], sys.argv[3], sys.argv[4], sys.argv[5], sys.argv[6], sys.argv[7]]
result = updateCustomer(filename, updatedLine)
print(result)
exit(1)
#The "report" function requires 1 parameter: ID
if sys.argv[1] == "report":
if(len(sys.argv) < 3):
print("You should provide Customer ID to be reported")
exit(0)
else:
reportedID = sys.argv[2]
result = reportCustomer(filename, reportedID)
if result == "not exist":
print(result)
else:
print("The final expenditure for Customer " + reportedID + " is " + str(result[0]) + ", and the evaluation is " + result[1])
exit(1)
#If the program reaches this point, it means that the function is not accurately indicated in the command line
print("You should indicate one of the following four functions: add, delete, update, report")
import pytest
import campaign
from campaign import record
#Following is a sample test case to test the "add" function
def test_add():
#The following defines the input data
filename = "list.csv"
inputData = ['123456', 'Liu', 'Huai', '150', '250', '350']
#The following executes the function 'add' with the input data "123456 Liu Huai 150 250 350"
result = record.addCustomer(filename, inputData)
#The following verifies if the execution result is consistent with expectation
assert result == "added"
#Following is a sample test case to test the "update" function
def test_update():
#The following defines the input data
filename = "list.csv"
inputData = ['123456', 'Liu', 'Huai', '250', '250', '350']
#The following executes the function 'add' with the input data "123456 Liu Huai 250 250 350"
result = record.updateCustomer(filename, inputData)
#The following verifies if the execution result is consistent with expectation
assert result == "updated"
#Following is a sample test case to test the "report" function
def test_report():
#The following defines the input data
filename = "list.csv"
inputData = '123456'
#The following executes the function 'report' with the input data "123456"
result = record.reportCustomer(filename, inputData)
#The following verifies if the execution result is consistent with expectation
assert result[0] == 850 and result[1] == 'Loss'
#Following is a sample test case to test the "delete" function
def test_delete():
#The following defines the input data
filename = "list.csv"
inputData = '123456'
#The following executes the function 'delete' with the input data "123456"
result = record.deleteCustomer(filename, inputData)
#The following verifies if the execution result is consistent with expectation
assert result == "deleted"
ID,Surname,Given Name,Mouse,Bag,Lamp
611822,Bagnoli,Ansel,270,280,330
430024,Heimisson,Cian,240,170,0
318489,Farmer,Izem,130,150,290
111041,Hudson,Theano,150,130,140
269090,Dunn,Iob,200,180,290
839678,Beaufort,Shivani,250,200,280
123456,Liu,Huai,150,250,350
Semester 2, 2023 COS80022 - Software Quality and Testing Task 1: Traceability matrix (3 points) Traceability matrix is a useful tool supporting a good test planning as well as efficient test management and control. You are required to create and maintain a traceability matrix (in a tabular format) to map and trace requirements with test assets (including test conditions, test cases, and execution status) created in the whole testing process. Assignment 2 (P&C) Hint: A sample traceability matrix will be provided in a template ('SQT_Assignment2_template.doc'). Relevant information about traceability matrix can be found in lectures (e.g., slides 73-75 in Week 5's lecture and slides 16, 22&41 in Week 6's lecture). Task 2: Functional testing (19 points) You are required to undertake all activities for the dynamic testing of all four major functions described in the beginning of this document and detailed in Appendix A. Specifically, you should complete the following three sub-tasks: Sub-task 2.1: Test analysis (4 points) You are required to analyse the requirements given in Appendix A on all four major functions of record.py, and define corresponding test conditions, which are normally elaborated into high-level test cases. Note that each defined test condition should be associated with a professional description (in one or two sentences) about "what to test". All test conditions should also be put into the traceability matrix created in Task 1 to map with requirements. Hint: Relevant information and examples about test analysis and test conditions can be found in lectures (e.g., slides 15-25 in Week 6's lecture). Sub-task 2.2: Test design (10 points) You are required to design concrete test cases based on the test conditions defined in sub-task 2.1. Note that: Each test case should contain concrete values for input data as well as expected result such that they can be directly executed. One test condition may be associated with one or more concrete test cases. You should select and apply appropriate techniques (e.g., black-box and white-box) when designing test cases for each particular function ("add", "delete", "update", or "report" in record.py). All test cases should also be put into the traceability matrix created in Task 1 to map with test conditions as well as requirements. Hint: Four sample test cases are provided in a template pytest script 'test_assignment2_xxxxxx.py' (further described in Appendix B) and briefly described in the sample document 'SQT_Assignment2_template.doc'. Relevant information and examples about test design, test cases, and concrete testing techniques can be found in lectures (e.g., slides 68-69 in Week 5's lecture, slides 26-30 in Week 6's lecture, and Week 8&9's lecture content). You have also practiced different techniques for designing test cases in our labs (e.g., lab work in Week 6, 8&9). 2
Step by Step Solution
There are 3 Steps involved in it
Step: 1
Task 1 Traceability Matrix A traceability matrix maps requirements to test conditions test cases and their execution status You can use a table format in Word Excel or any other suitable tool Here is ...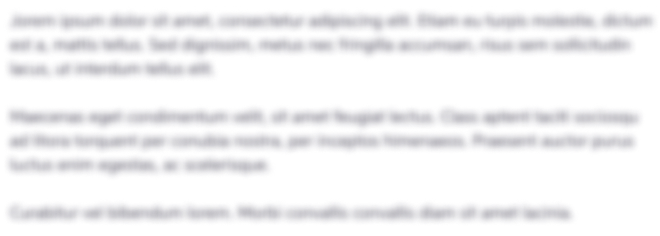
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started