Question
This week we will again be writing classes. We will be using Inheritance and Polymorphism as a way to make our code more efficient. We
This week we will again be writing classes. We will be using Inheritance and Polymorphism as a way to make our code more efficient. We will also be implementing Interfaces and Abstract classes to aid our efforts.
Shape3D
PART I:
Write an interface Shape3D that will be used by other classes
Write instance method declarations:
public double getVolume()
public double getSurfaceArea()
NOTE: Remember interfaces only have method headers - no bodies.
PART II:
Write an abstract class CircularShape that implements the Shape3D interace.
Declare one instance variable (field) for radius of type double.
Write a constructor that takes the radius as a parameter and initializes it to 0.
Write instance methods:
public double getDiameter() that returns the diameter of a circular object
public double getRadius() that returns the radius intance variable (accessor)
public double getCrossSectionArea()- that returns the cross section area of a circular object (hint: look up this formula if needed)
public double getCrossSectionPerimeter()- that returns the cross section perimeter of a circular object (**make sure to use the getDiameter method you already have in your formula for cross section perimeter) (look up formula if needed)
PART III:
Write an abstract class CircularShapeWithHeight that extends CircularShape
Declare one instance variable (field) for height of type double.
Write a constructor that takes the radius and height as parameters and initializes them.
Hint: You need to use super to call the CircularShape constructor, passing radius as a parameter
Write accessor method:
public double getHeight() that returns the height
PART IV:
Write a class CircularCone that extends CircularShapeWithHeight
Write a 2-parameter constructor that takes the radius and height as parameters and initializes them.
Hint: You want to use super to call the CircularShapeWithHeight constructor, passing radius and height as parameters
Write instance methods:public double getSurfaceArea() - that returns the surface area of a Cone
Hint: To access radius and height, you can call the getRadius() and getHeight() accessor methods contained in CircularShape and CircularShapeWithHeight, respectively. (b/c of inheritance)
public double getVolume() - that returns the volume of a Cone
You now have a getCrossSectionArea() method in CircularShape that can substitute in for part of the formula. Again, use the getHeight() method to access the height.
PART V:
Write a class Cylinder that extends CircularShapeWithHeight
Write a 2-parameter constructor that takes the radius and height as parameters and initializes them.
Hint: You want to use super to call the CircularShapeWithHeight constructor, passing radius and height as parameters
Write instance methods:public double getSurfaceArea() - that returns the surface area of a Cone
Hint: To access radius and height, you can call the getRadius() and getHeight() accessor methods contained in CircularShape and CircularShapeWithHeight, respectively.
You must also use getCrossSectionPerimeter() and getCrossSectionArea()methods from the CircularShape class in your calculations
public double getVolume() - that returns the volume of a Cone
You now have a getCrossSectionArea() method in CircularShape that can substitute in for part of the formula. Again, use the getHeight() method to access the height.
PART VI:
Once you have completed all of your classes, download and add the ShapesTUI class and the ShapesDriver class to the same folder or project as your other class files in order to test your program. You can run and test your program from the ShapesDriver class. (*Note: Don't worry if you don't understand all that is happening in these)
// this is the ShapesTUI program
import java.util.Scanner;
/* This is the test class for the Shapes3D project * @author CS 1302 * @version 9/26/17 */
public class ShapesTUI
{
private Scanner keyboardInput;
private double radius;
private double height;
public ShapesTUI()
{
this.keyboardInput = new Scanner(System.in);
this.radius = 0;
this.height = 0;
}
/*
*Calls methods necessary for getting input and displaying results
*/
public void run()
{
getRadiusAndHeight();
displayCircularCone();
displayCylinder();
}
/*
*Gets input from the user
*/
public void getRadiusAndHeight()
{
System.out.print("Please Enter the Radius of the Shape: ");
this.radius = keyboardInput.nextDouble();
System.out.print("Please Enter the Height of the Shape: ");
this.height = keyboardInput.nextDouble();
}
/*
*Displays the Cone Volume and Surface
*/
public void displayCircularCone()
{
CircularCone cone = new CircularCone(this.radius, this.height);
System.out.println();
System.out.printf("The Volume of a Circular Cone with those Dimensions is: %.2f", cone.getVolume());
System.out.printf(" The Surface of a Cirular Cone with those Dimensions is: %.2f", cone.getSurfaceArea());
}
/*
*Dislays the Cylinder Volume and Surface
*/
public void displayCylinder()
{
Cylinder tube = new Cylinder(this.radius, this.height);
System.out.println();
System.out.printf(" The Volume of a Cylinder with those Dimensions is: %.2f", tube.getVolume());
System.out.printf(" The Surface of a Cylinder with those Dimensions is: %.2f", tube.getSurfaceArea());
}
}
// This is the ShapeDriver program
public class ShapeDriver {
/**
* @param args
*/
public static void main(String[] args) {
ShapesTUI demo = new ShapesTUI();
demo.run();
}
}
Model Classes > Shape3D +getVolume() +getSurfaceArea CircularShape - ra dius: double +CircularShape() +CircularShape(radius: double) getDiameter(): double getRadius(): double + getCrossSectionArea(): double + getCrossSectionPerimeter(): double CircularShapeWithHeight - height: double + CircularShapeWithHeight() +CircularShapeWithHeight(radius: double, height: double) + getHeight(): double CircularCone Cylinder + CircularCone() + CircularCone(radius: double, height: double) + getVolume(): double (override) + getSurfaceArea(): double (override) + Cylinder( Cylinder(radius: double, height: double) + getVolume(): double (override) + getSurfaceArea(): double (override)Step by Step Solution
There are 3 Steps involved in it
Step: 1
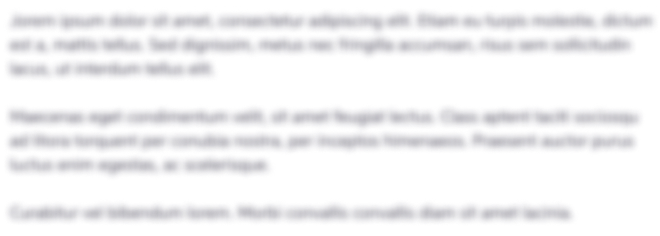
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started