Question
Tic Tac Toe Game: Help, please. Design and implement a console based Tic Tac Toe game. The objective of this project is to demonstrate your
Tic Tac Toe Game: Help, please.
Design and implement a console based Tic Tac Toe game. The objective of this project is to demonstrate your understanding of various programming concepts including Object Oriented Programming (OOP) and design.
Tic Tac Toe is a two player game. In your implementation one opponent will be a human player and the other a computer player.
? The game is played on a 3 x 3 game board.
? The first player is known as X and the second is O.
? X always goes first.
? Players alternate placing Xs and Os on the game board.
? Winning: ? The opponent that gets 3 of their assigned symbol in a row (horizontal, vertical or diagonal) wins!
? Draw: ?If no one has 3 in a row and all nine squares are filled no one wins, the game is a draw
You will need to design your program using OOP design, meaning you must use classes, interfaces etc as needed to implement your program. This section describes some required functionality to get you started. Some code is given to get you started, note you can change this code to fit your needs. You can create more than the listed classes etc below if needed. Implement A Game Board
? Must represent a 3 x 3 game board. ? Hint: ?Use two dimensional array. ? The data type used is up to you (examples: String, Char or a custom class that represents a cell).
? Must include methods? that implement the following functionality: ? Build the initial game board, this will create a game board with empty cells.
Example:
| |
-----------
| X |
-----------
| |
? Prints the current board that represents current board / game state. ? Checks board and returns a list of current, valid moves.
? A valid move is an empty space on the board. Players can only place their symbol in an empty cell.
? Hint:? A move is simply represented by a two values, row and column. These are the indices for the space on the game board (two dimensional array). ? Applies a players move to the board. ? Getter and setter methods for any instance variables you need to expose to other classes to use.
GameState (Enum)
? Enumeration that represents game state. This code is given. IllegalMoveException
? Create this exception class, it should extend the Exception class. The constructor should take a String message. The message given will describe the move that was illegal. Implement A Rules Engine
? Must include methods? that implement the following functionality: ? Method that checks for draw game state. ? Method that checks for winning game state (diagonals, rows and columns). ? Method that checks if a proposed move (i.e. row,column pair) by a player is a valid move.
? Hint:? Get list of valid moves from board class, then check the move against the list.
? If the move is invalid, raise an IllegalMoveException. The caller should catch and handle this exception, returning appropriate message to the user
ComputerPlayer
? File is given, but you must fill in the parts where it says Your Code Goes Here
? Some code such as the method to implement generating a valid move for the computer player is implemented for you.
? When the computer wins, it should display a taunting catch phrase. ? Create a method that randomly selects from a list of custom catch phrases (Strings), and returns the selected phrase. Implement A Game Class
? This is the driver class for your game.
****************************************************************
public class ComputerPlayer
{
private int[][] preferredMoves = {
{1, 1}, {0, 0}, {0, 2}, {2, 0}, {2, 2},
{0, 1}, {1, 0}, {1, 2}, {2, 1}};
private Board board;
//----- YOUR CODE GOES HERE:
// ADDED INSTANCE VARIABLE THAT STORES THE GAME SYMBOL ASSIGNED TO THE COMPUTER PLAYER
/** Constructor with reference to game board */
public ComputerPlayer(Board board)
{
this.board = board;
}
//----- YOUR CODE GOES HERE:
// ADD A SETTTER METHOD FOR THE INSTANCE VARIABLE THAT
// STORES THE GAME SYMBOL ASSIGNED TO THE COMPUTER PLAYER
/** Search for the first empty cell, according to the preferences
* @return int array of two values [row, col]
*/
public int[] generateMove()
{
for (int[] move : preferredMoves)
{
// checks for empty space on board
// (i.e. checks if this "move" is available, if the space is empty its available)
if (this.board[move[0]][move[1]] == " ")
{
return move;
}
}
return null;
}
}
****************************************************
public enum GameState { PLAYING, DRAW, X_WON, O_WON }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
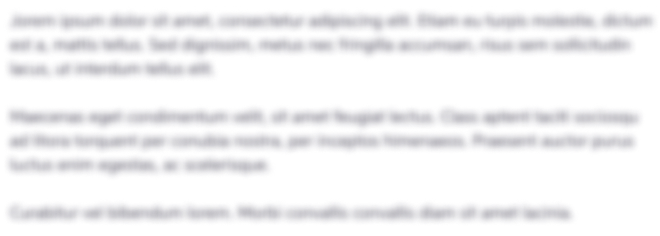
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started