Question
Tic Tac Toe Java Hello and good afternoon I am having some problems trying to follow the instructions for this assignment. Fo far this is
Tic Tac Toe Java
Hello and good afternoon I am having some problems trying to follow the instructions for this assignment.
Fo far this is my code with the instruccions
import java.util.Random; import java.util.Scanner; public class TicTacToe { public static void main ( String[] args ) { Scanner in = new Scanner ( System.in ); char[][] board = new char[ 3 ][ 3 ];
// Initialize the board to spaces for (int row=0; row // Keep playing while the game isn't finished // Get the location from the user and validate it // Mark the position in the board according to the user's specified location // Print the board after the user plays // Check to see if the game is finished. If it is, break out of the loop. // Have the AI make a move // Print the board after the AI plays // Check to see who the winner is // If the winner is 'X' or 'O', print that, otherwise, it is a tie } /** * Makes a move for the AI, and marks the board with an 'O'. * * @param board The game board */ public static void moveAI ( char[][] board ) { // Validate that the random location generated is valid. // Keep recalculating the location if the one generated is not // an empty space. // Be sure to mark the position in the board with an 'O' } /** * Prints out the tic-tac-toe board * * @param board The game board */ public static void printBoard ( char[][] board ) { // Box drawing unicode characters: char a = '\u250c'; // U+250C : top-left char b = '\u2510'; // U+2510 : top-right char c = '\u2514'; // U+2514 : bottom-left char d = '\u2518'; // U+2518 : bottom-right char e = '\u252c'; // U+252C : top-vertical-connector char f = '\u2534'; // U+2534 : bottom-vertical-connector char g = '\u251c'; // U+251C : left-horizontal-connector char h = '\u2524'; // U+2524 : right-horizontal-connector char i = '\u253c'; // U+253C : center plus sign connector char j = '\u2500'; // U+2500 : horizontal char k = '\u2502'; // U+2502 : vertical String l = j + "" + j + "" + j; // Three horizontals // Print out the game board System.out.printf ( " 0 1 2 " + " %c%s%c%s%c%s%c " + "0 %c %c %c %c %c %c %c " + " %c%s%c%s%c%s%c " + "1 %c %c %c %c %c %c %c " + " %c%s%c%s%c%s%c " + "2 %c %c %c %c %c %c %c " + " %c%s%c%s%c%s%c ", a, l, e, l, e, l, b, k, board[0][0], k, board[0][1], k, board[0][2], k, g, l, i, l, i, l, h, k, board[1][0], k, board[1][1], k, board[1][2], k, g, l, i, l, i, l, h, k, board[2][0], k, board[2][1], k, board[2][2], k, c, l, f, l, f, l, d ); } /** * Checks the result of the game * * @param board The game board * @return 'X' if 'X' is the winner * 'O' if 'O' is the winner * 'T' if the game is a tie * 'N' if the game isn't finished */ public static char checkWinner( char[][] board ) { if (board[0][0] == 'X' && board[0][1] == 'X' && board[0][2] == 'X' || // Check row 0 board[1][0] == 'X' && board[1][1] == 'X' && board[1][2] == 'X' || // Check row 1 board[2][0] == 'X' && board[2][1] == 'X' && board[2][2] == 'X' || // Check row 2 board[0][0] == 'X' && board[1][0] == 'X' && board[2][0] == 'X' || // Check col 0 board[0][1] == 'X' && board[1][1] == 'X' && board[2][1] == 'X' || // Check col 1 board[0][2] == 'X' && board[1][2] == 'X' && board[2][2] == 'X' || // Check col 2 board[0][0] == 'X' && board[1][1] == 'X' && board[2][2] == 'X' || // Check diag \ board[0][2] == 'X' && board[1][1] == 'X' && board[2][0] == 'X' || // Check diag / board[0][0] == 'x' && board[0][1] == 'x' && board[0][2] == 'x' || // Check row 0 board[1][0] == 'x' && board[1][1] == 'x' && board[1][2] == 'x' || // Check row 1 board[2][0] == 'x' && board[2][1] == 'x' && board[2][2] == 'x' || // Check row 2 board[0][0] == 'x' && board[1][0] == 'x' && board[2][0] == 'x' || // Check col 0 board[0][1] == 'x' && board[1][1] == 'x' && board[2][1] == 'x' || // Check col 1 board[0][2] == 'x' && board[1][2] == 'x' && board[2][2] == 'x' || // Check col 2 board[0][0] == 'x' && board[1][1] == 'x' && board[2][2] == 'x' || // Check diag \ board[0][2] == 'x' && board[1][1] == 'x' && board[2][0] == 'x') // Check diag / { return 'X'; } else if (board[0][0] == 'O' && board[0][1] == 'O' && board[0][2] == 'O' || // Check row 0 board[1][0] == 'O' && board[1][1] == 'O' && board[1][2] == 'O' || // Check row 1 board[2][0] == 'O' && board[2][1] == 'O' && board[2][2] == 'O' || // Check row 2 board[0][0] == 'O' && board[1][0] == 'O' && board[2][0] == 'O' || // Check col 0 board[0][1] == 'O' && board[1][1] == 'O' && board[2][1] == 'O' || // Check col 1 board[0][2] == 'O' && board[1][2] == 'O' && board[2][2] == 'O' || // Check col 2 board[0][0] == 'O' && board[1][1] == 'O' && board[2][2] == 'O' || // Check diag \ board[0][2] == 'O' && board[1][1] == 'O' && board[2][0] == 'O' || // Check diag / board[0][0] == 'o' && board[0][1] == 'o' && board[0][2] == 'o' || // Check row 0 board[1][0] == 'o' && board[1][1] == 'o' && board[1][2] == 'o' || // Check row 1 board[2][0] == 'o' && board[2][1] == 'o' && board[2][2] == 'o' || // Check row 2 board[0][0] == 'o' && board[1][0] == 'o' && board[2][0] == 'o' || // Check col 0 board[0][1] == 'o' && board[1][1] == 'o' && board[2][1] == 'o' || // Check col 1 board[0][2] == 'o' && board[1][2] == 'o' && board[2][2] == 'o' || // Check col 2 board[0][0] == 'o' && board[1][1] == 'o' && board[2][2] == 'o' || // Check diag \ board[0][2] == 'o' && board[1][1] == 'o' && board[2][0] == 'o') // Check diag / { return 'O'; } boolean finished = true; // If there is a blank space in the board, the game isn't finished yet for (int i = 0; i < board.length; i++) for (int j = 0; j < board[ i ].length; j++) if (board[ i ][ j ] == ' ') finished = false; // If the board is finished and 'X' or 'O' wasn't returned, then it is a tie // Otherwise, the game is not finished yet if ( finished ) return 'T'; else return 'N'; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
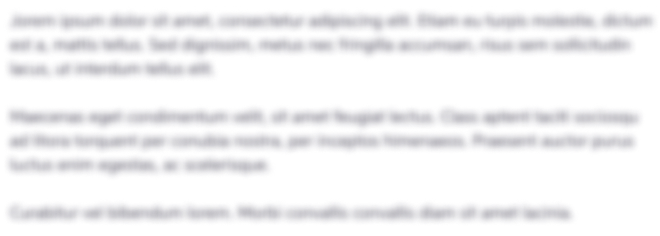
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started