Question
Time ADT Java programming: A value of Time represents a point in historical time, anywhere from the time of the dinosaurs to the distant future.
Time ADT Java programming:
A value of Time represents a point in historical time, anywhere from the time of the dinosaurs to the distant future. (Strictly speaking, the dinosaurs aren't historical, which is just was well since the numbers used internally cannot represent time back to the Big Bang). Values of Time should only be used for historical dates and dates in the "near future" future: AD 2235 is OK, AD 10000000 is not). Misuse of the range of time is the fault of the user not the ADT; your code doesn't need to detect such misuse.
The Time ADT provides the standard operations described above where toString() returns a UTC ("coordinated universal time" in its French acronym) time string such as "UTC AD 2018/09/04 14:00:00" which is when the class starts. (14:00 is Greenwich Mean time for 9:00am CDT). You will need to use the library class SimpleDateFormat.
The Time ADT provides the following additional operations:
Time() Return the current time (now!)
Time(Calendar) Return the time corresponding to the calendar instance given.
difference(Time) Return the time between this and the argument as a Duration. The result is always a non-negative duration whehter the argument is before or after this time.
add(Duraction) Return the new time after adding the given extent of time to this time.
subtract(Duraction) Return the new time after subtracting the given extent of time.
We require that you implement Time using a long field that represents milliseconds after the Java Epoch (UTC AD 1970/01/01 00:00:00). If the number is negative, the time falls before the epoch. As with Duration, the Time class should not reveal the representation, or even the epoch through public methods. The Calendar library class has a method getTimeInMillis which will be useful. Also see the function System.currentTimeMillis() for how to get the current time in terms of milliseconds since the Epoch
package homework2;
import java.text.DateFormat; import java.text.SimpleDateFormat; import java.util.Calendar; import java.util.Date; import java.util.Objects; import java.util.TimeZone;
/** * A point in time. */ public class Time { // TODO: declare a final long field., // representing milliseconds since the Java epoch. // The solution also has a private constructor, // which is very useful -- we recommend you declare it here. /** * Create a time for now. */ public Time() { // TODO (see homework) one line of code } /** * Create a time according to the time parameter. * @param c calendar object representing a time, must not be null */ public Time(Calendar c) { // TODO: one line of code } // Override/implement four methods standard for immutable classes. /** * Return the difference between two time points. * The order doesn't matter --- the difference is always a * (positive) Duration. * @param t time point to compute difference with * @return duration between two time points. */ public Duration difference(Time t) { return null; // TODO (one line of code) // Duration has an easy way to create 'n' times some constant duration }
/** * Return the time point after a particular duration. * If the point advances too far into the future, * more than a hundred million years from now, this * method may malfunction. * @param d duration to advance, must not be null * @return new time after given duration */ public Time add(Duration d) { return null; // TODO (one line of code) // Duration has an easy way to ask how many of some constant Duration it is. } /** * Return the time point before a particular duration. * If a point regresses too far into the past, * more than a hundred million years from now, * this method may malfunction. * @param d duration to regress, must not be null * @return new time before this one by the given duration. */ public Time subtract(Duration d) { return null; // TODO (one line of code) // Very similar to add } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
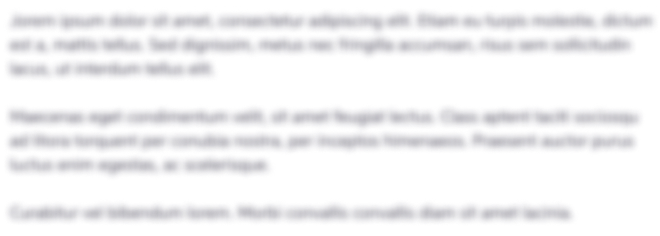
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started