Question
Time complexity and Big-O notation in Java !! 1. The sum_getImple Method /** * Get the total of the values in an integer list *
Time complexity and Big-O notation in Java !!
1. The sum_getImple Method
/** * Get the total of the values in an integer list * @param nums The list of integers * @return The sum of the values */ public int sum_getImple(java.util.List
I'm really confused how to define which one is the right one for each method. Please help
When nums is an ArrayList, the sum_getImple method is what: O(N),linear time. O(1) constant time, or O(N^2) quadratic time ?
When nums is a LinkedList, the sum_getImple method is what: O(N), linear time. O(1) constant time, or O(N^2) quadratic time?
=================================================
2. The sum_iteratorImple Method
/** * Get the total of the values in an integer list * @param nums The list of integers * @return The sum of the values */ public int sum_iteratorImple(java.util.List
When nums is an ArrayList, the sum_iteratorImple method is what ? O(N), linear time. O(1) constant time, or O(N^2) quadratic time? When nums is an LinkedList, the sum_iteratorImple method is what ?
==============================================
3. The removeEvens_getImple Method
/** * Remove the even values from a list of integers. * The method modifies the parameter list. * @param nums The list of integers */ public void removeEvens_getImple(java.util.List
When nums is an ArrayList, the removeEvens_getImple method is O(? When nums is an LinkedList, the removeEvens_getImple method is O(?
============================================
4. The removeEvens_iteratorImple Method
/** * Remove the even values from a list of integers. * The method modifies the parameter list. * @param nums The list of integers */ public void removeEvens_iteratorImple(java.util.List
When nums is an ArrayList, the removeEvens_iteratorImple method is O(? When nums is an LinkedList, the removeEvens_iteratorImple method is O(?
=================================================
5. The double_getImple Method
/** * Double the values in a list of integers. * This method modifies the parameter list. * @param nums The list of integers */ public void double_getImple(java.util.List
When nums is an ArrayList, The double_getImple Method is O(? When nums is an LinkedList, The double_getImple Method is O(?
===================================================
6. The double_iteratorImple Method
/** * Double the values in a list of integers. * This method modifies the parameter list. * @param nums The list of integers */ public void double_iteratorImple(java.util.List
When nums is an ArrayList, The double_iteratorImple Method is O(? When nums is an LinkedList, The double_iteratorImple Method is O(?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
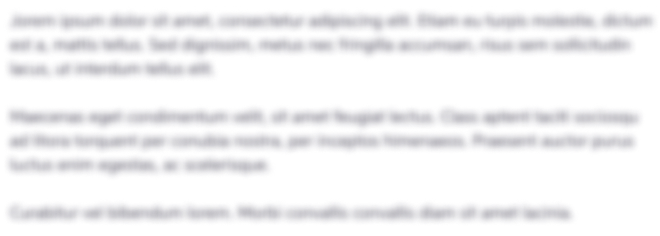
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started