Question
Time Driver #include #include Time.h using namespace std; void ShowNowTime(Time& timept) { cout < < Time is now ; timept.Display(); cout < < endl; }
Time Driver
#include
#include "Time.h"
using namespace std;
void ShowNowTime(Time& timept) {
cout << "Time is now ";
timept.Display();
cout << endl;
}
int main()
{
Time nowTime;
nowTime.Set(11, 30, 'P');
ShowNowTime(nowTime);
cout << "Forward 20 Minutes." << endl;
//nowTime.Forward(20);
nowTime += 20;
ShowNowTime(nowTime);
cout << "Forward 20 Minutes." << endl;
//nowTime.Forward(20);
nowTime += 20;
ShowNowTime(nowTime);
cout << "Forward 500 Minutes." << endl;
//nowTime.Forward(500);
nowTime += 500;
ShowNowTime(nowTime);
cout << "Forward 330 Minutes." << endl;
//nowTime.Forward(330);
nowTime += 330;
ShowNowTime(nowTime);
cout << "Forward 30 Minutes. (Testing the + operator.)" << endl;
Time testTime = nowTime + 30;
ShowNowTime(testTime);
cout << endl;
cout << "Testing Assignment Operator." << endl;
Time testTime(9, 30, 'P');
testTime.Display();
testTime = 10;
testTime.Display();
testTime = 7;
testTime.Display();
testTime = 13;
testTime.Display();
}
time.h
#pragma once
#include
using namespace std;
class Time {
public:
// Constructors
Time();
Time(unsigned hours, unsigned minutes, char am_pm);
// Functions to get the values of member
unsigned getHours() const; // Return the hour number
unsigned getMinutes() const; // Return the minute number
char getAMorPM() const; // Return the AM PM value
// Set, Display as discussed in class
void Set(unsigned hours, unsigned minutes, char am_pm);//Set the time
void Display() const; //Display the time
// A convenient forward function
void Forward(int minutes);
Time operator += (int minutes);
Time operator + (int);
private:
unsigned myHours, myMinutes;
char myAMorPM;
};
Time .cpp
#include "Time.h"
#include
#include
using namespace std;
Time :: Time ()
{
}
Time :: Time (unsigned hours, unsigned minutes, char am_pm)
{
Set(hours, minutes, am_pm);
}
void Time::Set(unsigned hours, unsigned minutes, char am_pm)
{
if (hours >= 1 && hours <= 12 && minutes >= 0 && minutes <= 59 &&
(am_pm == 'A' || am_pm == 'P'))
{
myHours = hours;
myMinutes = minutes;
myAMorPM = am_pm;
}
else
cerr << "*** Can't set these values *** ";
}
void Time::Display() const
{
string minStr = to_string(myMinutes);
if (myMinutes == 0) {
minStr = "00";
}
cout << myHours << ':' << minStr << ' ' << myAMorPM << ".M." <<
endl;
}
unsigned Time::getHours() const {
return myHours;
}
unsigned Time::getMinutes() const {
return myMinutes;
}
char Time::getAMorPM() const {
return myAMorPM;
}
// Forward the time by a given number of minutes
void Time::Forward(int minutesToForward)
{
int totalMinutes = myMinutes + minutesToForward;
myMinutes = totalMinutes % 60;
int hoursAdded = totalMinutes / 60;
int nowHours = myHours + hoursAdded;
int flipAmPm = ((nowHours / 12) - (myHours / 12)) % 2;
if (flipAmPm == 1) {
if (myAMorPM == 'A') {
myAMorPM = 'P';
}
else {
myAMorPM = 'A';
}
}
myHours = nowHours % 12;
if (myHours == 0) {
myHours = 12;
}
}
Time Time :: operator += (int minutesToForward)
{
this -> Forward(minutesToForward);
return * this;
}
Time Time :: operator + (int minutesToForward)
{
Time newTime = * this;
newTime.Forward(minutesToForward);
return newTime;
}
Do not mess with the driver. my functiosn fir some reason will not overlaod
Time Driver
#include
#include "Time.h"
using namespace std;
void ShowNowTime(Time& timept) {
cout << "Time is now ";
timept.Display();
cout << endl;
}
int main()
{
Time nowTime;
nowTime.Set(11, 30, 'P');
ShowNowTime(nowTime);
cout << "Forward 20 Minutes." << endl;
//nowTime.Forward(20);
nowTime += 20;
ShowNowTime(nowTime);
cout << "Forward 20 Minutes." << endl;
//nowTime.Forward(20);
nowTime += 20;
ShowNowTime(nowTime);
cout << "Forward 500 Minutes." << endl;
//nowTime.Forward(500);
nowTime += 500;
ShowNowTime(nowTime);
cout << "Forward 330 Minutes." << endl;
//nowTime.Forward(330);
nowTime += 330;
ShowNowTime(nowTime);
cout << "Forward 30 Minutes. (Testing the + operator.)" << endl;
Time testTime = nowTime + 30;
ShowNowTime(testTime);
cout << endl;
cout << "Testing Assignment Operator." << endl;
Time testTime(9, 30, 'P');
testTime.Display();
testTime = 10;
testTime.Display();
testTime = 7;
testTime.Display();
testTime = 13;
testTime.Display();
}
time.h
#pragma once
#include
using namespace std;
class Time {
public:
// Constructors
Time();
Time(unsigned hours, unsigned minutes, char am_pm);
// Functions to get the values of member
unsigned getHours() const; // Return the hour number
unsigned getMinutes() const; // Return the minute number
char getAMorPM() const; // Return the AM PM value
// Set, Display as discussed in class
void Set(unsigned hours, unsigned minutes, char am_pm);//Set the time
void Display() const; //Display the time
// A convenient forward function
void Forward(int minutes);
Time operator += (int minutes);
Time operator + (int);
private:
unsigned myHours, myMinutes;
char myAMorPM;
};
Time .cpp
#include "Time.h"
#include
#include
using namespace std;
Time :: Time ()
{
}
Time :: Time (unsigned hours, unsigned minutes, char am_pm)
{
Set(hours, minutes, am_pm);
}
void Time::Set(unsigned hours, unsigned minutes, char am_pm)
{
if (hours >= 1 && hours <= 12 && minutes >= 0 && minutes <= 59 &&
(am_pm == 'A' || am_pm == 'P'))
{
myHours = hours;
myMinutes = minutes;
myAMorPM = am_pm;
}
else
cerr << "*** Can't set these values *** ";
}
void Time::Display() const
{
string minStr = to_string(myMinutes);
if (myMinutes == 0) {
minStr = "00";
}
cout << myHours << ':' << minStr << ' ' << myAMorPM << ".M." <<
endl;
}
unsigned Time::getHours() const {
return myHours;
}
unsigned Time::getMinutes() const {
return myMinutes;
}
char Time::getAMorPM() const {
return myAMorPM;
}
// Forward the time by a given number of minutes
void Time::Forward(int minutesToForward)
{
int totalMinutes = myMinutes + minutesToForward;
myMinutes = totalMinutes % 60;
int hoursAdded = totalMinutes / 60;
int nowHours = myHours + hoursAdded;
int flipAmPm = ((nowHours / 12) - (myHours / 12)) % 2;
if (flipAmPm == 1) {
if (myAMorPM == 'A') {
myAMorPM = 'P';
}
else {
myAMorPM = 'A';
}
}
myHours = nowHours % 12;
if (myHours == 0) {
myHours = 12;
}
}
Time Time :: operator += (int minutesToForward)
{
this -> Forward(minutesToForward);
return * this;
}
Time Time :: operator + (int minutesToForward)
{
Time newTime = * this;
newTime.Forward(minutesToForward);
return newTime;
}
Do not mess with the driver. my functiosn fir some reason will not overlaod
Step by Step Solution
There are 3 Steps involved in it
Step: 1
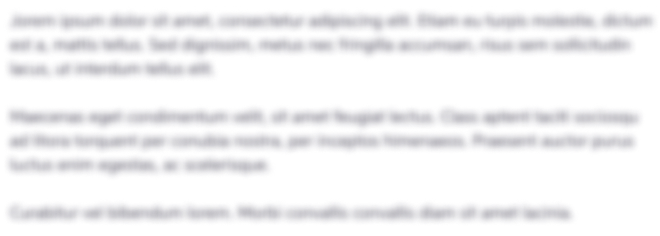
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started