Question
Time Event Waiting Time 1 Arrival 1 Departure 0 2 Arrival 3 Arrival 4 Departure 0 4 Departure 1 4 Arrival 5 Departure 0 5
Time | Event | Waiting Time |
1 | Arrival |
|
1 | Departure | 0 |
2 | Arrival |
|
3 | Arrival |
|
4 | Departure | 0 |
4 | Departure | 1 |
4 | Arrival |
|
5 | Departure | 0 |
5 | Arrival |
|
6 | Departure | 0 |
6 | Arrival |
|
8 | Arrival |
|
8 | Arrival |
|
9 | Arrival |
|
10 | Arrival |
|
11 | Departure | 0 |
11 | Arrival |
|
11 | Arrival |
|
11 | Arrival (Overflow) |
|
12 | Arrival (Overflow) |
|
12 | Arrival (Overflow) |
|
13 | Departure | 3 |
13 | Arrival |
|
14 | Departure | 5 |
14 | Departure | 5 |
14 | Departure | 4 |
14 | Arrival |
|
15 | Departure | 3 |
15 | Departure | 4 |
15 | Arrival |
|
16 | Departure | 2 |
16 | Departure | 2 |
18 | Arrival |
|
19 | Departure | 1 |
20 | Departure | 1 |
22 | Arrival |
|
22 | Departure | 0 |
22 | Arrival |
|
23 | Arrival |
|
23 | Arrival |
|
24 | Departure | 0 |
24 | Departure | 1 |
24 | Departure | 1 |
Arrival Time | Time Dequeued | Waiting Time |
5 |
| 0 |
5 | 15 | 10 |
7 | 25 | 18 |
12 | 35 | 23 |
12 | 45 | 33 |
13 | 55 | 42 |
14 | - | Overflow |
18 | 65 | 47 |
19 | - | Overflow |
25 | 75 | 50 |
Time | Event | Waiting Time |
5 | Arrival |
|
5 | Arrival |
|
7 | Arrival |
|
12 | Arrival |
|
12 | Arrival |
|
13 | Arrival |
|
14 | Arrival (Overflow) |
|
15 | Departure | 0 |
18 | Arrival |
|
19 | Arrival (Overflow) |
|
25 | Departure | 10 |
25 | Arrival |
|
35 | Departure | 18 |
45 | Departure | 23 |
55 | Departure | 33 |
65 | Departure | 42 |
75 | Departure | 47 |
85 | Departure | 50 |
=====================================================================================
Textbook Solution to Original Problem which needs expanding:
CarWash Class
import java.util.LinkedList; import java.util.Queue;
public class CarWash {
public final static String OVERFLOW = " (Overflow) "; public final static String HEADING = " Time\tEvent\tWaiting Time "; public final static int MAX_SIZE = 5; public final static int WASH_TIME = 10; public final static int INFINITY = 10000; private Queue
--------------------------------------------------------------------------------------------------------------
CarWashTest Class
import static org.junit.Assert.*;
public class CarWashTest extends CarWash{ @Test public void twoArrivalTest() { carWash.processArrival (5); results = carWash.processArrival (7); assertTrue (results.indexOf ("5\tArrival") != -1); assertTrue (results.indexOf ("7\tArrival") > results.indexOf ("5\tArrival")); } //method twoArrivalTest @Test public void overflowTest() { carWash.processArrival (5); carWash.processArrival (7); carWash.processArrival (8); carWash.processArrival (12); carWash.processArrival (12); assertTrue (carWash.processArrival (13).toString().indexOf (CarWash.OVERFLOW) == -1); /o overflow for arrival at 13 assertTrue (carWash.processArrival (14).toString().indexOf (CarWash.OVERFLOW) > 0); //overflow for arrival at 14 } //method overflowTest
}
----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
Car Class
public class Car { private int arrivalTime; public Car() { } // just in case public Car(int a){ arrivalTime = a; } // constructor public void setArrivalTime(int a) { arrivalTime = a; } //setArrivalTime public int getArrivalTime(){ return arrivalTime; } //getArrivalTime
} // Car
--------------------------------------------------------------------------------------------------------------
CarWashUser Class
import java.util.LinkedList; import java.util.Scanner;
public class CarWashUser {
public static void main(String[] args) { CarWash carWash = new CarWash(); Scanner in = new Scanner(System.in); int nextArrivalTime; while (true){ System.out.println("Please enter the next arrival time" + " or 999 to quit"); nextArrivalTime = in.nextInt(); if (nextArrivalTime == 999) break; carWash.process(nextArrivalTime); } carWash.finishUp(); System.out.println(" Here are the results of the simulation: "); LinkedList
----------------------------------------------------------------------------------------------------------------
Thank You!
*Original Car Wash Simulation Question and textbook solution posted below question Making the Speed o's Car Wash Simulation More Realistic Problem Expand the Car Wash Simulation Question with random arrival and service times Use unit testing JUnit Testing) of new methods after you have specified those methods Analysis The arrival times-with a Poisson distribution- should be generatedrandomly from the mean arrival time. Speedo has added a new feature: The service time is not necessarily 10 minutes, but depends on what the customer wants done, such as wash only, wash and wax, wash and vacuum, and so on. The service time for a car should be calculated just before the car enters the wash station- that's when the customer knows how much time will be taken until the customer leaves the car wash. The service times, also with a Poisson distribution, should be generator used for arrival times The input consists of three positive integers: the mean arrival time, the mean service time and the maximum arrival time. Repeatedly re prompt until each value is a positive integer Calculate the average waiting time and the average queue line) length, both to one fractional digits. The average waiting time is the sum of the waiting times divided by the number of customers The average queue length is the sum of the queue lengths for each minute of the simulation divided by the number of minutes until the last customer departs. To calculate the sum of the queue lengths, we add, for each minute of the simulation, the total number of customers on the queue during that minute. We can calculate this sum another way: we add, for each customer, the total number of minutes that customer was on the queue. But this is the sum of the waiting times! So we can calculate the average queue length as the sum of the waiting times divided by the number of minutes of the simulation until the last cu stomer departs. And we already calculated the sum of the waiting times for the averag e waiting time Also calculate the number of overflows. Use a seed of 100 for the random number generator, so the output you get should have the same values as the output from the following tests System Test l: the input is in boldface) Please enter the mean arrival time: 3 Please enter the mean service time: 5 Please enter the maximum arrival time: 25Step by Step Solution
There are 3 Steps involved in it
Step: 1
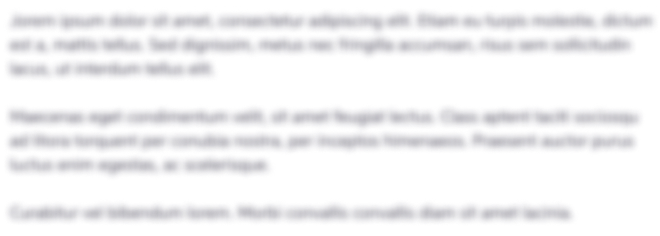
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started