Question
To be able to code an abstract class structure with attributes and methods with appropriate access specifiers. To demonstrate the concept of interfaces. Provide practice
To be able to code an abstract class structure with attributes and methods with appropriate access specifiers. To demonstrate the concept of interfaces. Provide practice creating objects of different types, overloaded methods, ArrayLists, encapsulation, and static variables.
Use the inventory classes from your previousassignment, but change the super class to an abstract class.
Add the use of a static variable to keep track of the total number of objects in the inventory. Display the number of inventory items.
Use encapsulation and related setters and getters for the inventory ID number.
Make an interface (printItem) that is implemented in each subclass.The method will print all the fields of an object.For this assignment, do not use an overridden toString. Access the elements and provide labels using direct attribute access.
Make at least 3 objects of each subclass and store them in an ArrayList for each type.Using a loop and your overridden printItem method, print out all the items in each ArrayList to display the entire inventory to the user.
PREVIOUS ASSIGNMEMT class InventoryItem {
public int supplyLevel;
public int count;
public int id;
public double price;
//Default Constructor
public InventoryItem() {
this.supplyLevel = 0;
this.count = 0;
this.id = 0;
this.price = 0.0;
}
//Overloaded Constructor
public InventoryItem(int supplyLevel,
int count,
int id,
double price)
{
this.supplyLevel = supplyLevel;
this.count = count;
this.id = id;
this.price = price;
}
//toString method
@Override
public String toString() {
return "InventoryItem [supplyLevel=" + supplyLevel + ", count=" + count + ", id=" + id + ", price=" + price
+ "]";
}
}
class Charcoal extends InventoryItem {
String typeOfCharcoal;
String hardnessLevel;
//Default Constructor
public Charcoal(String typeOfCharcoal,
String hardnessLevel)
{
super();
this.typeOfCharcoal = "";
this.hardnessLevel = "";
}
//Overloaded Constructor
public Charcoal(int supplyLevel,
int count,
int id,
double price,
String typeOfCharcoal,
String hardnessLevel)
{
super(supplyLevel, count, id, price);
this.typeOfCharcoal = typeOfCharcoal;
this.hardnessLevel = hardnessLevel;
}
@Override
public String toString() {
return "Charcoal [typeOfCharcoal=" + typeOfCharcoal + ", hardnessLevel=" + hardnessLevel + ", supplyLevel=" + supplyLevel
+ ", count=" + count + ", id=" + id + ", price=" + price + "]";
}
}
class Juice extends InventoryItem {
int shelfLife;
boolean organic;
//Default Constructor
public Juice(int shelfLife, boolean organic) {
super();
this.shelfLife = 0;
this.organic = true;
}
//Overloaded Constructor
public Juice(int supplyLevel, int count, int id, double price, int shelfLife, boolean organic) {
super(supplyLevel, count, id, price);
this.shelfLife = shelfLife;
this.organic = organic;
}
//toString method
@Override
public String toString() {
return "juice [shelfLife=" + shelfLife + ", organic=" + organic + ", supplyLevel=" + supplyLevel + ", count="
+ count + ", id=" + id + ", price=" + price + "]";
}
}
public class Main{
public static void main(String args[]) {
Charcoal Charcoal1 = new Charcoal(100, 75, 1, 45, "Neutral", "Good");
Charcoal Charcoal2 = new Charcoal(100, 85, 2, 35, "Hard", "Medium");
Charcoal Charcoal3 = new Charcoal(100, 95, 3, 25, "Soft", "Low");
InventoryItem[] charcoalArray = new InventoryItem[3];
charcoalArray[0]=Charcoal1;
charcoalArray[1]=Charcoal2;
charcoalArray[2]=Charcoal3;
Juice juice1 = new Juice(10, 10, 1, 18, 2, true);
Juice juice2 = new Juice(9, 4, 2, 14, 1, false);
Juice juice3 = new Juice(8, 7, 3, 20, 1, true);
InventoryItem[] juiceArray = new InventoryItem[3];
juiceArray[0] = juice1;
juiceArray[1] = juice2;
juiceArray[2] = juice3;
for(int i=0;i
System.out.println(charcoalArray[i].toString());
}
for(int i=0;i
System.out.println(juiceArray[i].toString());
}
}
}
Step by Step Solution
3.35 Rating (155 Votes )
There are 3 Steps involved in it
Step: 1
1 Abstract Superclass Java public abstract class InventoryItem private static int totalItems 0 Stati...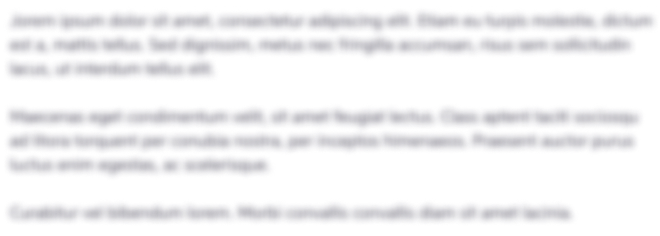
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started