Question
to complete the code for double linked list. only the DLinkedList class to be completed as instructions given in the comments. class DLinkedListNode: # An
to complete the code for double linked list.
only the DLinkedList class to be completed as instructions given in the comments.
class DLinkedListNode:
# An instance of this class represents a node in Doubly-Linked List
def __init__(self,initData,initNext,initPrevious):
self.data = initData
self.next = initNext
self.previous = initPrevious
if initNext != None:
self.next.previous = self
if initPrevious != None:
self.previous.next = self
def getData(self):
return self.data
def setData(self,newData):
self.data = newData
def getNext(self):
return self.next
def getPrevious(self):
return self.previous
def setNext(self,newNext):
self.next = newNext
def setPrevious(self,newPrevious):
self.previous = newPrevious
class DLinkedList:
# An instance of this class represents the Doubly-Linked List
def __init__(self):
self.__head=None
self.__tail=None
self.__size=0
def search(self, item):
current = self.__head
found = False
while current != None and not found:
if current.getData() == item:
found= True
else:
current = current.getNext()
return found
def index(self, item):
current = self.__head
found = False
index = 0
while current != None and not found:
if current.getData() == item:
found= True
else:
current = current.getNext()
index = index + 1
if not found:
index = -1
return index
def add(self, item):
# TODO:adds a new node (containing the item as its data) to the head of the list.
pass
def remove(self, item):
# TODO:removes the first element in the list that is equal to item. If the item is not in the list, the list is not changed and an exception should NOT be raised.
pass
def append(self, item):
# TODO:adds a new node (containing the item as its data) to the tail of the list.
pass
def insert(self, pos, item):
# TODO:adds a new node (containing the item as its data) at the given position in the list. For example, if pos is 0, then the new node goes at the beginning (head) of the list. pos must be an integer, and for this exercise, cannot be negative.
pass
def pop1(self):
# TODO: removes and returns the last item from the list
pass
def pop(self, pos=None):
# TODO: removes and returns the item in the given position. An error should be raised if the position is outside of the list. pos must be an integer, and for this exercise, cannot be negative.
# Hint - incorporate pop1 when no pos argument is given
pass
def searchLarger(self, item):
# TODO: returns the position of the first element that is larger than item, or -1 if there is no larger item.
pass
def getSize(self):
# TODO:returns the number of elements in the list.
pass
def getItem(self, pos):
# TODO:returns the item at the given position. An exception should be raised if the position is outside of the list. pos must be an integer, and it can be positive OR negative.
pass
def __str__(self):
# TODO:returns a string of the elements in the doubly-linked list, with one space in between each element.
pass
def test():
linked_list = DLinkedList()
is_pass = (linked_list.getSize() == 0)
assert is_pass == True, "fail the test"
linked_list.add("World")
linked_list.add("Hello")
is_pass = (str(linked_list) == "Hello World")
assert is_pass == True, "fail the test"
is_pass = (linked_list.getSize() == 2)
assert is_pass == True, "fail the test"
is_pass = (linked_list.getItem(0) == "Hello")
assert is_pass == True, "fail the test"
is_pass = (linked_list.getItem(1) == "World")
assert is_pass == True, "fail the test"
is_pass = (linked_list.getItem(0) == "Hello" and linked_list.getSize() == 2)
assert is_pass == True, "fail the test"
is_pass = (linked_list.pop(1) == "World")
assert is_pass == True, "fail the test"
is_pass = (linked_list.pop() == "Hello")
assert is_pass == True, "fail the test"
is_pass = (linked_list.getSize() == 0)
assert is_pass == True, "fail the test"
int_list2 = DLinkedList()
for i in range(0, 10):
int_list2.add(i)
int_list2.remove(1)
int_list2.remove(3)
int_list2.remove(2)
int_list2.remove(0)
is_pass = (str(int_list2) == "9 8 7 6 5 4")
assert is_pass == True, "fail the test"
for i in range(11, 13):
int_list2.append(i)
is_pass = (str(int_list2) == "9 8 7 6 5 4 11 12")
assert is_pass == True, "fail the test"
for i in range(21, 23):
int_list2.insert(0,i)
is_pass = (str(int_list2) == "22 21 9 8 7 6 5 4 11 12")
assert is_pass == True, "fail the test"
is_pass = (int_list2.getSize() == 10)
assert is_pass == True, "fail the test"
int_list = DLinkedList()
is_pass = (int_list.getSize() == 0)
assert is_pass == True, "fail the test"
for i in range(0, 1000):
int_list.append(i)
correctOrder = True
is_pass = (int_list.getSize() == 1000)
assert is_pass == True, "fail the test"
for i in range(0, 200):
if int_list.pop() != 999 - i:
correctOrder = False
is_pass = correctOrder
assert is_pass == True, "fail the test"
is_pass = (int_list.searchLarger(200) == 201)
assert is_pass == True, "fail the test"
int_list.insert(7,801)
is_pass = (int_list.searchLarger(800) == 7)
assert is_pass == True, "fail the test"
is_pass = (int_list.getItem(-1) == 799)
assert is_pass == True, "fail the test"
is_pass = (int_list.getItem(-4) == 796)
assert is_pass == True, "fail the test"
if is_pass == True:
print ("=========== Congratulations! Your have finished exercise 2! ============")
if __name__ == '__main__':
test()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
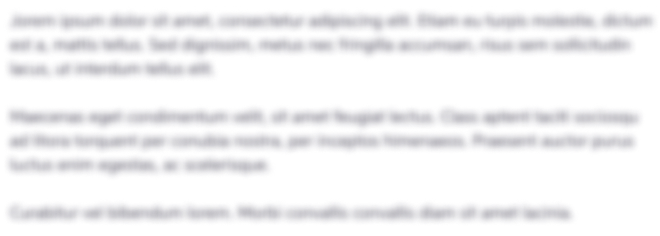
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started