Question
To complete this project, you must submit the following: Note: All class files must be submitted because the entire package of files is required for
To complete this project, you must submit the following:
Note: All class files must be submitted because the entire package of files is required for the application to run.
Driver.java Class File Submit your modified Driver.java class file. Be sure to include in-line comments for all your changes and additions.
Cruise.java Class File Submit your modified Cruise.java class file. Be sure to include in-line comments for all your changes and additions.
Ship.java Class File Submit the Ship.java class file, even though you were not required to make changes to it for this project.
Passenger.java Class File Submit the Dog.java class file, even though you were not required to make changes to it for this project.
Supporting Materials
The envisioned system will include three primary components: ships, cruises, and passengers. The Luxury Ocean Cruise Outings company does not own ships; instead, it creates cruises using available ships. Once a ship is placed in service, it is available for cruises. Passengers can be added to cruises. Here are the relationships of these components:
- Ships have unique names.
- Cruises have unique names and are assigned to ships.
- Ships can have multiple cruises.
- Each ship has a finite number of passenger cabins (Balcony, Ocean View, Suite, and Interior). Overbooking is not permitted.
- Passengers are assigned to cruises.
System Menu
We require a simple text-based menu system that should resemble the illustration below.
public class Cruise {
// Class Variables private String cruiseName; private String cruiseShipName; private String departurePort; private String destination; private String returnPort;
// Constructor - default Cruise() { }
// Constructor - full Cruise(String tCruiseName, String tShipName, String tDeparture, String tDestination, String tReturn) { cruiseName = tCruiseName; cruiseShipName = tShipName; departurePort = tDeparture; destination = tDestination; returnPort = tReturn; }
// Accessors public String getCruiseName() { return cruiseName; }
public String getCruiseShipName() { return cruiseShipName; }
public String getDeparturePort() { return departurePort; }
public String getDestination() { return destination; }
public String getReturnPort() { return returnPort; }
// Mutators public void setCruiseName(String tVar) { cruiseName = tVar; }
public void setCruiseShipName(String tVar) { cruiseShipName = tVar; }
public void setDeparturePort(String tVar) { departurePort = tVar; }
public void setDestination(String tVar) { destination = tVar; }
public void setReturnPort(String tVar) { returnPort = tVar; }
// print cruise details public void printCruiseDetails() {
// complete this method
}
// method added to print ship's name vice memory address @Override public String toString() { return cruiseName; } }
import java.util.ArrayList; import java.util.Scanner;
import static java.lang.Integer.parseInt;
public class Driver {
// class variables (add more as needed) private static ArrayList
public static void main(String[] args) {
initializeShipList(); // initial ships initializeCruiseList(); // initial cruises initializePassengerList(); // initial passengers
// add loop and code here that accepts and validates user input // and takes the appropriate action. include appropriate // user feedback and redisplay the menu as needed
}
// hardcoded ship data for testing // Initialize ship list public static void initializeShipList() { add("Candy Cane", 20, 40, 10, 60, true); add("Peppermint Stick", 10, 20, 5, 40, true); add("Bon Bon", 12, 18, 2, 24, false); add("Candy Corn", 12, 18, 2, 24, false); }
// hardcoded cruise data for testing // Initialize cruise list public static void initializeCruiseList() { Cruise newCruise = new Cruise("Southern Swirl", "Candy Cane", "Miami", "Cuba", "Miami"); cruiseList.add(newCruise); }
// hardcoded cruise data for testing // Initialize passenger list public static void initializePassengerList() { Passenger newPassenger1 = new Passenger("Neo Anderson", "Southern Swirl", "STE"); passengerList.add(newPassenger1);
Passenger newPassenger2 = new Passenger("Trinity", "Southern Swirl", "STE"); passengerList.add(newPassenger2);
Passenger newPassenger3 = new Passenger("Morpheus", "Southern Swirl", "BAL"); passengerList.add(newPassenger3); }
// custom method to add ships to the shipList ArrayList public static void add(String tName, int tBalcony, int tOceanView, int tSuite, int tInterior, boolean tInService) { Ship newShip = new Ship(tName, tBalcony, tOceanView, tSuite, tInterior, tInService); shipList.add(newShip); }
public static void printShipList(String listType) { // printShipList() method prints list of ships from the // shipList ArrayList. There are three different outputs // based on the listType String parameter: // name - prints a list of ship names only // active - prints a list of ship names that are "in service" // full - prints tabbed data on all ships
if (shipList.size()
// complete this code block
public class Passenger {
// Class variables private String passengerName; private String passengerCruise; private String passengerRoomType;
// Constructor - default Passenger() { }
// Constructor - full Passenger(String pName, String pCruise, String pRoomType) { passengerName = pName; passengerCruise = pCruise; passengerRoomType = pRoomType; // should be BAL, OV, STE, or INT }
// Accessors public String getPassengerName() { return passengerName; }
public String getPassengerCruise() { return passengerCruise; }
public String getPassengerRoomType() { return passengerRoomType; }
// Mutators public void setPassengerName(String tVar) { passengerName = tVar; }
public void setPassengerCruise(String tVar) { passengerCruise = tVar; }
public void setPassengerRoomType(String tVar) { passengerRoomType = tVar; }
// print method public void printPassenger() { int spaceCount; String spaces1 = ""; String spaces2 = ""; spaceCount = 20 - passengerName.length(); for (int i = 1; i
System.out.println(passengerName + spaces1 + passengerCruise + spaces2 + passengerRoomType); }
// method added to print passenger's name vice memory address @Override public String toString() { return passengerName; }
}
public class Ship {
// Class Variables private String shipName; private int roomBalcony; private int roomOceanView; private int roomSuite; private int roomInterior; private boolean inService;
// Constructor - default Ship() { }
// Constructor - full Ship(String tName, int tBalcony, int tOceanView, int tSuite, int tInterior, boolean tInService) { shipName = tName; roomBalcony = tBalcony; roomOceanView = tOceanView; roomSuite = tSuite; roomInterior = tInterior; inService = tInService; }
// Accessors public String getShipName() { return shipName; }
public int getRoomBalcony() { return roomBalcony; }
public int getRoomOceanView() { return roomOceanView; }
public int getRoomSuite() { return roomSuite; }
public int getRoomInterior() { return roomInterior; }
public boolean getInService() { return inService; }
// Mutators public void setShipName(String tVar) { shipName = tVar; }
public void setRoomBalcony(int tVar) { roomBalcony = tVar; }
public void setRoomOceanView(int tVar) { roomOceanView = tVar; }
public void setRoomSuite(int tVar) { roomSuite = tVar; }
public void setRoomInterior(int tVar) { roomInterior = tVar; }
public void setInService(boolean tVar) { inService = tVar; }
// print method public void printShipData() { int spaceCount; String spaces = ""; spaceCount = 20 - shipName.length();
for (int i = 1; i
System.out.println(shipName + spaces + roomBalcony + "\t" + roomOceanView + "\t" + roomSuite + "\t" + roomInterior + "\t\t" + inService); }
// method added to print ship's name vice memory address @Override public String toString() { return shipName; } }
Luxury Ocean Cruise Outings System Menu [1] Add Ship [2] Edit Ship [3] Add Cruise [4] Edit Cruise [5] Add Passenger [6] Edit Passenger [x] Exit System [A] Print Ship Names [B] Print Ship In Service List [C] Print Ship Full List [D] Print Cruise List [E] Print Cruise Details [F] Print Passenger List Enter a menu selection: Luxury Ocean Cruise Outings System Menu [1] Add Ship [2] Edit Ship [3] Add Cruise [4] Edit Cruise [5] Add Passenger [6] Edit Passenger [x] Exit System [A] Print Ship Names [B] Print Ship In Service List [C] Print Ship Full List [D] Print Cruise List [E] Print Cruise Details [F] Print Passenger List Enter a menu selectionStep by Step Solution
There are 3 Steps involved in it
Step: 1
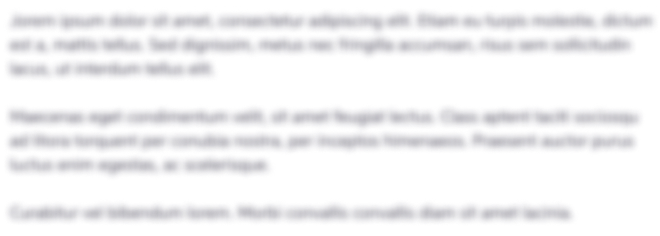
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started