Question
// TO DO: add your implementation and JavaDocs public class MyAbacus implements Abacus { // ADD MORE PRIVATE MEMBERS HERE IF NEEDED! // Remember: Using
// TO DO: add your implementation and JavaDocs
public class MyAbacus implements Abacus {
// ADD MORE PRIVATE MEMBERS HERE IF NEEDED!
// Remember: Using an array in this class = no credit on the project!
public MyAbacus(int base) {
//constructor
// throws IllegalArgumentException if base is invalid
// remember: an abacus should always have at least one
// column!
}
public int getBase() {
// O(1)
return -1; //default return, make sure to remove/change
}
public int getNumPlaces() {
// O(1)
return -1; //default return, make sure to remove/change
}
public int getBeadsTop(int place) {
// O(1)
return -1; //default return, make sure to remove/change
}
public int getBeadsBottom(int place) {
// O(1)
return -1; //default return, make sure to remove/change
}
public boolean equals(MyAbacus m) {
// O(N) where N is the number of places currently
// in use by the abacus
return false; //default return, make sure to remove/change
}
public DynArr310 add(String value) {
// Hints:
// see: https://docs.oracle.com/javase/8/docs/api/java/lang/Integer.html#parseInt-java.lang.String-int-
// and: https://docs.oracle.com/javase/8/docs/api/java/lang/String.html#charAt-int-
// Also... I personally found a recursive helper function really, really useful here...
// Important: each Abacus in the DynArr310 returned should
// be a copy of this abacus. If you just add this abacus over and over
// you'll just get the final abacus shown multiple times in the GUI.
return null; //default return, make sure to remove/change
}
// --------------------------------------------------------
// example testing code... edit this as much as you want!
// --------------------------------------------------------
public static void main(String[] args) {
//this is the sequence from the project description
Abacus a = new MyAbacus(10);
DynArr310 steps;
AbacusGUI.printAbacus(a);
AbacusGUI.fullPrintAdd(a, "36");
AbacusGUI.fullPrintAdd(a, "12");
AbacusGUI.fullPrintAdd(a, "2");
AbacusGUI.fullPrintAdd(a, "12");
AbacusGUI.fullPrintAdd(a, "10");
AbacusGUI.fullPrintAdd(a, "2");
AbacusGUI.fullPrintAdd(a, "68");
AbacusGUI.fullPrintAdd(a, "50");
AbacusGUI.fullPrintAdd(a, "10");
AbacusGUI.fullPrintAdd(a, "5");
AbacusGUI.fullPrintAdd(a, "3");
AbacusGUI.fullPrintAdd(a, "128");
AbacusGUI.fullPrintAdd(a, "3000000");
}
}
https://docs.oracle.com/javase/8/docs/api/java/lang/String.html#charAt-int- // Also... I personally found a recursive helper function really, really useful here... // Important: each Abacus in the DynArr310 returned should // be a copy of this abacus. If you just add this abacus over and over // you'll just get the final abacus shown multiple times in the GUI. return null; //default return, make sure to remove/change } // -------------------------------------------------------- // example testing code... edit this as much as you want! // -------------------------------------------------------- public static void main(String[] args) { //this is the sequence from the project description Abacus a = new MyAbacus(10); DynArr310 steps; AbacusGUI.printAbacus(a); AbacusGUI.fullPrintAdd(a, "36"); AbacusGUI.fullPrintAdd(a, "12"); AbacusGUI.fullPrintAdd(a, "2"); AbacusGUI.fullPrintAdd(a, "12"); AbacusGUI.fullPrintAdd(a, "10"); AbacusGUI.fullPrintAdd(a, "2"); AbacusGUI.fullPrintAdd(a, "68"); AbacusGUI.fullPrintAdd(a, "50"); AbacusGUI.fullPrintAdd(a, "10"); AbacusGUI.fullPrintAdd(a, "5"); AbacusGUI.fullPrintAdd(a, "3"); AbacusGUI.fullPrintAdd(a, "128"); AbacusGUI.fullPrintAdd(a, "3000000"); } }
//--------------------------------------------------------
//DO NOT EDIT ANYTHING BELOW THIS LINE
//--------------------------------------------------------
/**
* Interface for an abacus for the AbacusGUI to use.
*
*/
public interface Abacus {
/**
* Gets the number base of the abacus. This base
* will never be an odd number and never be less
* than 2.
*
* @return the number base of the abacus
*/
public int getBase();
/**
* Returns the number of places (bead columns) the
* abacus is using to represent the number. This
* will never be less than one.
*
* @return the number of places in use
*/
public int getNumPlaces();
/**
* Gets the number of beads in the top area of the
* abacus which are in-use for a given place. (The
* number of beads in the top which are pushed down
* to the center.)
*
* @param place the beads column of interest (0 is the right-most place)
* @return the number of beads currently in use
* @throws IndexOutOfBoundsException if the place requested is not in use
*/
public int getBeadsTop(int place);
/**
* Gets the number of beads in the bottom area of the
* abacus which are in-use for a given place. (The
* number of beads in the bottom which are pushed up
* to the center.)
*
* @param place the beads column of interest (0 is the right-most place)
* @return the number of beads currently in use
* @throws IndexOutOfBoundsException if the place requested is not in use
*/
public int getBeadsBottom(int place);
/**
* Adds the given string representation of a number to
* the current value of the abacus. The abacus is updated
* to this new position. It returns the steps to perform
* the add (snap shots of the abacus at each step). The
* abacus may be left in an "improper state" if the
* provided arguements are invalid.
*
*
A snapshot is required for each of the following steps:
*
- - the initial state
*
- - the final state
*
- - expansions (beads should not be moved, the abacus just
* becomes bigger/smaller)
*
- - exchanges (beads are exchanged in one step)
*
- - movement of X beads up OR down (not both at the same time)
* in ONE place on the bottom OR top of the abacus (not both
* at the same time)
*
*
*
* @param value the string representation of the value to add (e.g. "100" in base 10, or "1f" in base 16)
* @return the different positions the abacus was in (including the start and finish states)
* @throws NumberFormatException if string is not correct for the base
*/
public DynArr310
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
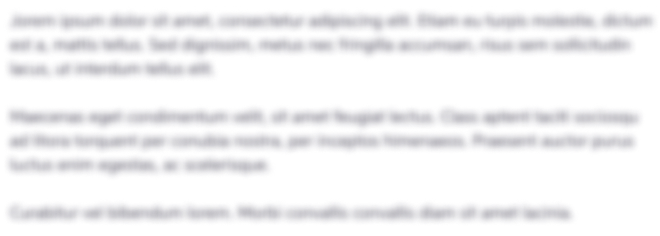
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started