Question
Topic 12: Exceptions Requirements chapter 12 zyLab: Exceptions This project will contains two template files in zyBooks chapter 12 (and at the end of this
Topic 12: Exceptions
Requirements chapter 12 zyLab: Exceptions
This project will contains two template files in zyBooks chapter 12 (and at the end of this document):
Auto.java a class definition
EvaluateAuto.java contains main() method
Use the template files provided to complete the following exercises. And include complete JavaDoc style documentation for both files.
Each step of the instructions is associated with specific tests, so you can test your program as you go along.
Program Requirements
An automobile dealership needs to do calculations for their cars, based on the gas tank capacity and average range the car can travel on one tank of gas.
Write a program to create objects that represent home mortgage loans.
Test 1 (5 pts):
Within the EvaluateAuto class:
- Write code to read an automobile make/model, gas tank capacity and average range the car can drive on one tank from the user, using the prompts as shown below.
- After reading the both inputs, display a "Both inputs valid integers" message (no error checking required here)
Example:
Enter make and model of car:
Honda Accord
Enter tank capacity (in whole gallons):
10
Enter average range (in whole miles):
250
Both inputs valid integers
Tests 2 through 4 (10 pts, 10 pts, 5 pts):
Within the EvaluateAuto class:
- Add an exception handler that includes:
- A try block around the code that reads the second two user inputs.
- If no exceptions are automatically thrown,
- A try block around the code that reads the second two user inputs.
the code will still display the same "Both inputs valid integers" message as before
- A catch block to catch the InputMismatchException thrown if the user does not enter an integer for one of the integer inputs.
- The catch block should display
Error - did not enter a valid integer value
Test 5 (10 pts):
Within the Auto class:
- Add a constructor that has parameters for the data fields.
- Add a getters for the data fields.
Test 6 (5 pts):
Within the Auto class:
- Add a calcMPG() method that will divide avgRange by tankCapacity to determine and return an integer containing the average whole mile per gallon the car gets
(NOTE: use integer division to ignore any remainder).
Test 7 (10 pts):
Within the EvaluateAuto class:
- Add code to the try block in the main method to:
- Display a blank line
- Create an object with the user entered values as arguments to the constructor
- Use the object created to call the calcMPG() method and display the results in this format:
Honda Accord gets approximately 10 miles per gallon
(using any necessary getters)
Test 8 (10 pts):
Within the EvaluateAuto class:
- Add a second catch block that will catch the ArithmeticException that may be automatically thrown when the calcMPG () method is called and the tank capacity is 0 during the division.
- The catch block should display
Error - cannot determine mpg when tank capacity is 0
Test 9 to 11 (25 pts total):
Within the Auto class:
- Add a displayMakeAd() method that will:
- Find the first space in the makeModel string (that separates the make and model)
- When the String does not contain a space, throw a RuntimeException with the message
"Error invalid make model"
- Otherwise display only the make stored in the Auto object, within the following type of message: Always buy make!
For example:
Always buy Honda!
Within the EvaluateAuto class:
- Add code to the try block in the main method to:
- Use the object created to call the displayMakeAd() method.
- Add a third catch block that will catch the RuntimeException that may be thrown when the
displayMakeAd() method is called.
-
- The catch block should display the message from the RuntimeException object
- Warning: Do NOT display a quoted String here
display the message from the RuntimeException object
Submission
You can develop and test all of your code directly in the zyLab area
or start with the templates on the next page in NetBeans and copy your code over when done. After you finish coding your solution, submit in zyLab for testing.
-
- Class description
*
- @author
- @version
*/
public class Auto {
private String makeModel;
private int tankCapacity;
private int avgRange;
// Insert your code here
}
EvaluateAuto.java template
/**
*
*/ import java.util.InputMismatchException; import java.util.Scanner; public class EvaluateAuto { public static void main(String[] args) { // Insert your code here } } |
1: Compare output for reading inputkeyboard_arrow_up
4 / 4
Input
Honda Accord 15 375
Your output correctly starts with
Enter make and model of car: Enter tank capacity (in whole gallons): Enter average range (in whole miles): Both inputs valid integers
2: Compare output for bad input value of tank capacitykeyboard_arrow_up
0 / 10
Output differs. See highlights below. Special character legend
Input
Honda Accord 1.5 375
Your output starts with
Enter make and model of car: Enter tank capacity (in whole gallons): Did not enter a valid integer value Enter average range (in whole miles): Both inputs valid integers Error - cannot determine mpg when tank capacity is 0 Always buy Accord!
Expected output starts with
Enter make and model of car: Enter tank capacity (in whole gallons): Error - did not enter a valid integer value
3: Compare output for bad input value of rangekeyboard_arrow_up
0 / 10
Output differs. See highlights below. Special character legend
Input
Honda Accord 15 twenty
Your output starts with
Enter make and model of car: Enter tank capacity (in whole gallons): Enter average range (in whole miles): Both inputs valid integers Honda Accord gets approximately 0 miles per gallon Always buy Accord!
Expected output starts with
Enter make and model of car: Enter tank capacity (in whole gallons): Enter average range (in whole miles): Error - did not enter a valid integer value
4: Compare output for good input valueskeyboard_arrow_up
5 / 5
Input
Chevy SS 19 380
Your output correctly starts with
Enter make and model of car: Enter tank capacity (in whole gallons): Enter average range (in whole miles): Both inputs valid integers
5: Unit testkeyboard_arrow_up
9 / 9
Tests constructor and getters within Auto class
Test feedback
PASSED: Constructor correctly set all data values and they are accessible via getters
6: Unit testkeyboard_arrow_up
5 / 5
Tests calcMPG() method within Auto class
Test feedback
PASSED: calcMPG calculates and returns miles per gallon correctly
7: Compare output of mpgkeyboard_arrow_up
0 / 10
Output differs. See highlights below. Special character legend
Input
Honda Accord 15 375
Your output starts with
Enter make and model of car: Enter tank capacity (in whole gallons): Enter average range (in whole miles): Both inputs valid integers Honda Accord gets approximately 25 miles per gallon A
Expected output starts with
Enter make and model of car: Enter tank capacity (in whole gallons): Enter average range (in whole miles): Both inputs valid integers Honda Accord gets approximately 25 miles per gallon
8: Compare output when tank capacity is 0keyboard_arrow_up
0 / 10
Output differs. See highlights below. Special character legend
Input
Honda Accord 0 375
Your output
Enter make and model of car: Enter tank capacity (in whole gallons): Enter average range (in whole miles): Both inputs valid integers Error - cannot determine mpg when tank capacity is 0 Always buy Accord!
Expected output
Enter make and model of car: Enter tank capacity (in whole gallons): Enter average range (in whole miles): Both inputs valid integers Error - cannot determine mpg when tank capacity is 0
9: Unit testkeyboard_arrow_up
0 / 10
Tests RuntimeException is thrown correctly when no space in makeModel
Your output
java.lang.NullPointerException
10: Compare output when RuntimeException is thrown keyboard_arrow_up
0 / 5
Output differs. See highlights below. Special character legend
Input
Tahoe 21 400
Your output
Enter make and model of car: Enter tank capacity (in whole gallons): Enter average range (in whole miles): Both inputs valid integers Tahoe gets approximately 19 miles per gallon null
Expected output
Enter make and model of car: Enter tank capacity (in whole gallons): Enter average range (in whole miles): Both inputs valid integers Tahoe gets approximately 19 miles per gallon Error - invalid make model
11: Compare make output when no exception is thrownkeyboard_arrow_up
0 / 10
Output differs. See highlights below. Special character legend
Input
Chevy Tahoe 21 400
Your output
Enter make and model of car: Enter tank capacity (in whole gallons): Enter average range (in whole miles): Both inputs valid integers Chevy Tahoe gets approximately 19 miles per gallon Always buy Tahoe!
Expected output
Enter make and model of car: Enter tank capacity (in whole gallons): Enter average range (in whole miles): Both inputs valid integers Chevy Tahoe gets approximately 19 miles per gallon Always buy Chevy!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
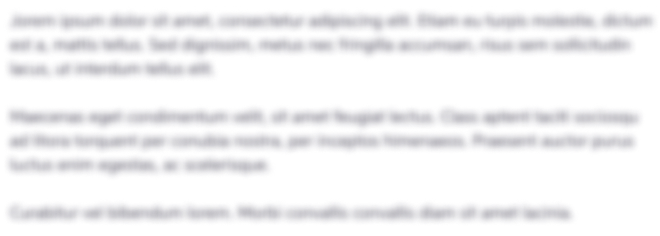
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started