Question
Trish at Bargain Used Books continues to use the programs you developed for her. You expect that at some point, Trish will ask you to
Trish at Bargain Used Books continues to use the programs you developed for her. You expect that at some point, Trish will ask you to extend the types of items that she currently sells. So you've decided to update the program you did for Lab 03 to use functions.
You will be rewriting Lab03P3.py to use functions. Your updated program will not change what the program does, but it will make it easier to enhance.
The instructor has provided a file called Lab05P1-FillThisIn.py. Download that file and rename it Lab05P1.py.
- Copy that file into your PyCharm project.
- Change the program header to include your name and the date.
The partial program is made up of 4 functions:
main - DO NOT CHANGE ANY OF THE CODE IN THIS FUNCTION.
This function is already complete. It calls the other 3 functions to accomplish its work:
- The main function calls get_item_count and then get_item_total 3 times to get the item costs for workbooks, textbooks, and magazines.
- Then the main function calls calc_and_display_receipt to print the receipt.
get_item_count
This function will need to be implemented.
- This function takes one parameters:
- max_allowed - maximum number of items the user can enter
- The function will ask the user to enter the number of items. If the user enters less than 0 or more than the max allowed, an error message will be printed and they will be asked to enter a different value. See the sample output for an example.
- The function will return the number of items the user enters.
HINT: This function is only asking about a SINGLE item type. It might be the workbooks, textbooks, magazines, or something else. Notice the prompt to the user just asks for an ITEM, not a specific item type. The solution the instructors came up with has only 5 lines of code in the implementation for this function. If you have more than that, you may not be implementing this function correctly.
get_item_total
This function will need to be implemented.
- This function takes two parameters:
- num_items - number of items
- unit_price - the cost of each item
- The function calculates the total cost for the items and returns that value.
calc_and_display_receipt
This function will need to be implemented.
- This function takes three parameters:
- wb_total - total cost of workbooks
- tb_total - total cost of textbooks
- m_total - total cost of magazines
- The function will calculate the total before tax.
- The function will calculate the sales tax using the function you created. Use the provided global constant for the sales tax.
- The function will calculate the total after tax.
- Then the function will print a receipt that contains the following information:
- Total cost of workbooks (if zero, skip this step)
- Total cost of textbooks (if zero, skip this step)
- Total cost of magazines (if zero, skip this step)
- A divider line
- Subtotal before tax
- Amount of tax to be charged
- Total including tax
Here are some sample outputs of running the program:
Workbooks
Enter the number of items: 8
Textbooks
Enter the number of items: 3
Magazines
Enter the number of items: 4
Workbooks: $68.00
Textbooks: $72.00
Magazines: $23.80
---------------------
Subtotal: 163.80
Tax: 9.83
Amount due: 173.63
Workbooks
Enter the number of items: -5
The number of items must be between 0 and 40
Enter the number of items: 3
Textbooks
Enter the number of items: 0
Magazines
Enter the number of items: -2
Number of items must be between 0 and 25
Enter the number of items: 4
Workbooks: $25.50
Magazines: $23.80
---------------------
Subtotal: 49.30
Tax: 2.96
Amount due: 52.26
NOTE: We are not handling issues where a user types an invalid integer, that is, an entry which cannot be converted using the int() function. We will handle that in a future lab.
Run this program. Take a screenshot of the results. Name the screenshot Lab05P1-ouput.jpg.
Submit both files, Lab05P1.py and Lab05P1-output.jpg, to Blackboard for credit.
Partial Program
# Global Constants - Use these in your program where it makes sense
TAX_RATE = 0.06 # 6% sales tax
WB_MAX = 40
WB_COST = 8.50
TB_MAX = 10
TB_COST = 24.00
MAG_MAX = 25
MAG_COST = 5.95
# The get_item_count function takes one parameter:
# max_allowed - Maximum number of items the user can enter
#
# This function will ask the user to enter the number of items and
# validate the number entered is between 0 and the max_allowed
# inclusive. The number of items the user enters is returned.
def get_item_count(): # You should fill in the parameters needed
pass # Replace pass with your implementation
# The get_item_total function takes two parameters:
# num_items - Number of items
# unit_price - The cost of each item
#
# This function calculates the total cost for the items and
# returns that value.
def get_item_total(): # You should fill in the parameters needed
pass # Replace pass with your implementation
# The calc_and_display_receipt function will calculate all the
# necessary values and display the receipt. It takes three parameters:
# wb_total - Total cost of workbooks
# tb_total - Total cost of textbooks
# m_total - Total cost of magazines
#
# This function will calculate total before tax, the tax on the total,
# and the total after tax is added.
#
# The receipt should display the total cost of workbooks, textbooks, and
# magazines IF the item cost is greater than 0. The receipt should also
# include the subtotal, tax, and total.
def calc_and_display_receipt(): # You should fill in the parameters needed
pass # Replace pass with your implementation
# main function - DO NOT MAKE ANY CHANGES TO CODE BELOW THIS LINE!
def main():
print('Workbooks')
wb_item_count = get_item_count(WB_MAX)
wb_total = get_item_total(wb_item_count, WB_COST)
print('Textbooks')
tb_item_count = get_item_count(TB_MAX)
tb_total = get_item_total(tb_item_count, TB_COST)
print('Magazines')
m_item_count = get_item_count(MAG_MAX)
m_total = get_item_total(m_item_count, MAG_COST)
calc_and_display_receipt(wb_total, tb_total, m_total)
# Call the main function.
main()
.
Step by Step Solution
3.34 Rating (148 Votes )
There are 3 Steps involved in it
Step: 1
Global Constants Use these in your program where it makes sense TAXRATE 006 6 sales tax WBMAX 40 WBC...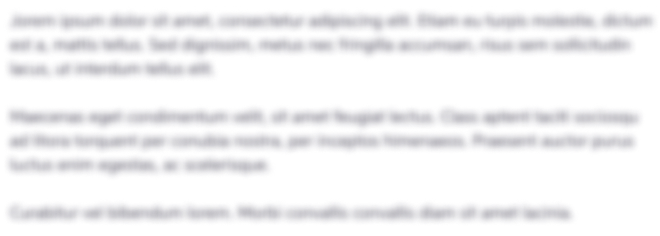
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started