Question
Trying to sort an array of 1's, 2's, and 3's on value ( i know there are easier sort methods, but i need to do
Trying to sort an array of 1's, 2's, and 3's on value ( i know there are easier sort methods, but i need to do it in linear time and make it "in-place"). the program keeps returning all 0's in the final array. what is wrong with my code?
import java.util.Arrays;
public class sort123 {
public static void main(String[] args) {
int[] A = {1,1,2,3,2};
int count[] = new int [4];
for (int i = 0; i < 4; i++) {
count[i] = 0;
}
for (int j = 0; j < A.length; j++) {
count[A[j]]++;
}
int [] dest = new int[3];
dest[0] = 0;
for (int i = 1 ; i < 3; i++) {
dest[i] = dest[i-1] + count[i-1];
}
int[] B = new int [A.length];
// move item from A to B
for(int j = 0 ; j < A.length; j++) {
B[dest[A[j]]] = A[j];
A = B;
}
System.out.println(java.util.Arrays.toString(A));
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
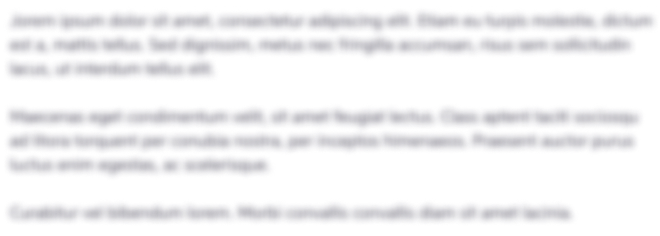
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started