Question
Turn the class in the following code into three different files. A main and you class should be in a source file and a header
Turn the class in the following code into three different files. A main and you class should be in a source file and a header file. I keep getting the "undefined reference to" error.
#include
using namespace std;
//TicTacToe class class TicTacToe { private: char game [3][3]; public: TicTacToe () {} bool playerTurn (char num, char Player); bool WinCheck (char Player, bool gameOver); bool DrawCheck(bool gameOver); void Gameboard(); void display(); }; // display function void TicTacToe::display() { int i = 0; int r = 0;
for ( i = 0; i < 3; i++) { for (r = 0; r < 3; r++) { if (r < 2) { cout << game[i][r] << " | "; } else { cout << game [i][r] << endl; } } } } //Board function void TicTacToe::Gameboard() { int n = 1; int i = 0; int r = 0;
for (i = 0; i < 3; i++) { for (r = 0; r < 3; r++) { game[i][r] = '0' + n; n++; } } }
//check for winner bool TicTacToe::WinCheck(char Player, bool gameOver) {
for ( int i = 0; i < 3; i++) { if (game[i][0] == game [i][1] && game [i][1] == game [i][2]) gameOver = true; }
for ( int i = 0; i < 3; i++) { if (game[0][i] == game [1][i] && game [1][i] == game [2][i]) gameOver = true; }
if (game [0][0] == game [1][1] && game [1][1] == game [2][2]) { gameOver = true; }
if (game [0][2] == game[1][1] && game [1][1] == game [2][0]) { gameOver = true; }
if (gameOver == true) { cout<< "Player " << Player << " wins! "; } return gameOver; }
//player turn function, to check entered option is valid or not bool TicTacToe::playerTurn(char num, char Player) { int i = 0; int r = 0; for (i = 0; i < 3; i++) { for ( r = 0; r < 3; r++) { if (game[i][r] == num) { game[i][r] = Player; return true; } } } return false; }
//check if it is a draw bool TicTacToe::DrawCheck(bool gameOver) { int n = 1; int i = 0; int r = 0; int counter = 0; for (i = 0; i < 3; i++) { for (r = 0; r < 3; r++) { if (game [i][r] == '0' +n) { counter++; } n++; } } if (counter < 1) { cout << " Draw "; gameOver = true; } return gameOver; }
//main function int main() { bool finish = false; bool gameOver = false; bool isValid; int number; char Player, num; srand(time(NULL));
number = rand()%2; if(number == 0) Player = 'X'; else Player = 'O';
cout <<"Tic Tac Toe" << endl;
TicTacToe myGame; //object for TicTacToe class //call the game board function myGame.Gameboard();
//display the board myGame.display(); do { isValid = false;
if(Player == 'X') { //get user choice Player = 'O'; cout << " Player " << Player << ", your turn:" << endl; cin >> num; isValid = myGame.playerTurn(num, Player); if(!isValid) while (!isValid) { cout << "Invalid selection choose again:" << endl; cin >> num; isValid = myGame.playerTurn(num, Player); } } else { Player = 'X'; cout << " Player " << Player << "'s turn" << endl; while (!isValid) { number = rand()%9+1; num = '0' + number; isValid = myGame.playerTurn(num, Player); } } myGame.display(); gameOver = myGame.WinCheck(Player, gameOver); gameOver = myGame.DrawCheck(gameOver);
if(gameOver == true) { cout << "Do you want to Play again? Y/N "; cin >> num; if (num == 'Y' || num == 'y') { cout << endl; myGame.Gameboard(); gameOver = false; } else { cout << "Bye " << endl; finish = true; } } }while(!finish);
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
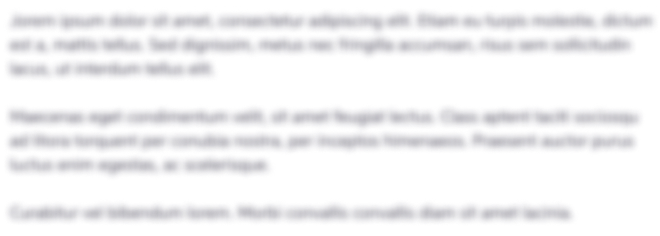
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started