Question
Two issues with this code that I need help (both code and explaining it) with: First, my public Month plus does not compile as it
Two issues with this code that I need help (both code and explaining it) with:
First, my public Month plus does not compile as it wants a return statement. I have a return as part of the for loop and am not sure what I would put outside of that or how I would move that return correctly.
Secondly I am very confused by DisplayStyle. I added abbreviations to each of my months at the beginning, but looking further, I am wondering if this is something that java includes. There was a separate enum set up as DisplayStyle that just has two values SHORT and LONG. Any help explaining that would be much appreciated though I am most concerned about my month plus return issue.
/** * An Enum representing the US months of the year. */ public enum Month { JANUARY(0,31,"JAN"), FEBRUARY(1,28,"FEB"), MARCH(2,31,"MAR"), APRIL(3,30,"APR"), MAY(4,31,"MAY"), JUNE(5,30,"JUN"), JULY(6,31,"JUL"), AUGUST(7,31,"AUG"), SEPTEMBER(8,30,"SEP"), OCTOBER(9,31,"OCT"), NOVEMBER(10,30,"NOV"), DECEMBER(11,31,"DEC"); private int order; private int dayTotals; private String SHORT; private Month(int o, int dT, String SHORT){ this.order = o; this.dayTotals = dT; this.SHORT = SHORT; } /** * Get the number of days in the month. Leap years are not considered. * Ex: January -> 31 * @return the number of days in a month */ public int getLength() { return this.dayTotals; } /** * Adds numberOfMonths to the current Month and returns what month it will then be. * @param numberOfMonths the number of months to look ahead * @return what month it will be numberOfMonths after the current month */ public Month plus(int numberOfMonths) { if (numberOfMonths <0){ throw new IllegalArgumentException("Number of months must be positive."); } int newOrderValue = (this.order + numberOfMonths)%12; for (Month month : Month.values()){ if (month.order == newOrderValue){ //this is where I returned the answer return month; } } //this is where I am getting an error when I try to compile saying I am missing a return statement. } /** * Return the name of the month in the requested style, either SHORT or default to LONG. For some months, * SHORT may be equal to LONG. * Ex: A SHORT style would return 'Jan' and the LONG style would return 'January' * @param style - the style the month should be returned in * @return the display name of the current month according to the requested style. */ public String getDisplayName(DisplayStyle style) { //TODO: Implement me return ""; } @Override public String toString(){ return name().substring(0,1) + name().substring(1).toLowerCase(); } }
I don't know if it helps, but I do have the testing code (provided to us as part of the assignment.
/** * This class will be used to write test methods that interact with the Month enum. */ public class MonthTester { public void testGetLengthMethod() { System.out.println("Testing getLength() method."); /* Month jan = Month.JANUARY; System.out.println("Testing: " + jan); int length = jan.getLength(); if(length == 31) { System.out.println(" Got the right number of days for January!"); } else { System.out.println(" Incorrect number of days for January. Should be 31, but got: " + length); } Month feb = Month.FEBRUARY; System.out.println("Testing: " + feb); length = feb.getLength(); if(length == 28) { System.out.println(" Got the right number of days for February!"); } else { System.out.println(" Incorrect number of days for February. Should be 28, but got: " + length); } Month june = Month.JUNE; System.out.println("Testing: " + june); length = june.getLength(); if(length == 30) { System.out.println(" Got the right number of days for June!"); } else { System.out.println(" Incorrect number of days for June. Should be 30, but got: " + length); } */ } public void testPlusMethod() { System.out.println("Testing plus() method."); /* Month jan = Month.JANUARY; System.out.println("Testing: " + jan + " plus 6 months."); Month sixMonthsLater = jan.plus(6); if(sixMonthsLater == Month.JULY) { System.out.println(" Correct: landed in July"); } else { System.out.println(" Landed in the wrong month. Should be July, but got: " + sixMonthsLater); } System.out.println("Testing: " + jan + " plus 18 months."); Month eighteenMonthsLater = jan.plus(18); if(eighteenMonthsLater == Month.JULY) { System.out.println(" Correct: landed in July"); } else { System.out.println(" Landed in the wrong month. Should be July, but got: " + eighteenMonthsLater); } Month aug = Month.AUGUST; System.out.println("Testing: " + aug + " plus 7 months."); Month sevenMonthsLater = aug.plus(7); if(sevenMonthsLater == Month.MARCH) { System.out.println(" Correct: landed in March"); } else { System.out.println(" Landed in the wrong month. Should be March, but got: " + sevenMonthsLater); } */ } public void testGetDisplayNameMethod() { System.out.println("Testing getDisplayName() method."); /* DisplayStyle shortStyle = DisplayStyle.SHORT; DisplayStyle longStyle = DisplayStyle.LONG; Month june = Month.JUNE; System.out.println("Testing: " + june + "."); String shortJune = june.getDisplayName(shortStyle); String longJune = june.getDisplayName(longStyle); if(shortJune.equals("June") && longJune.equals("June")) { System.out.println(" Correct: the short and long display are both: " + longJune); } else { System.out.println(" Incorrect display names. Expected Long: June, Short: June. Got Long: " + longJune + " Short: " + shortJune); } Month may = Month.MAY; System.out.println("Testing: " + may + "."); String shortMay = may.getDisplayName(shortStyle); String longMay = may.getDisplayName(longStyle); if(shortMay.equals("May") && longMay.equals("May")) { System.out.println(" Correct: the short and long display are both: " + longMay); } else { System.out.println(" Incorrect display names. Expected Long: May, Short: May. Got Long: " + longMay + " Short: " + shortMay); } Month july = Month.JULY; System.out.println("Testing: " + july + "."); String shortJuly = july.getDisplayName(shortStyle); String longJuly = july.getDisplayName(longStyle); if(shortJuly.equals("July") && longJuly.equals("July")) { System.out.println(" Correct: the short and long display are both: " + longJuly); } else { System.out.println(" Incorrect display names. Expected Long: July, Short: July. Got Long: " + longJuly + " Short: " + shortJuly); } Month sept = Month.SEPTEMBER; System.out.println("Testing: " + sept + "."); String shortSept = sept.getDisplayName(shortStyle); String longSept = sept.getDisplayName(longStyle); if(shortSept.equals("Sept") && longSept.equals("September")) { System.out.println(" Correct: the short and long display are different. Long: " + longSept + " Short: " + shortSept); } else { System.out.println(" Incorrect display names. Expected Long: September, Short: Sept. Got Long: " + longSept + " Short: " + shortSept); } Month oct = Month.OCTOBER; System.out.println("Testing: " + oct + "."); String shortOct = oct.getDisplayName(shortStyle); String longOct = oct.getDisplayName(longStyle); if(shortOct.equals("Oct") && longOct.equals("October")) { System.out.println(" Correct: the short and long display are different. Long: " + longOct + " Short: " + shortOct); } else { System.out.println(" Incorrect display names. Expected Long: October, Short: Oct. Got Long: " + longOct + " Short: " + shortOct); } */ } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
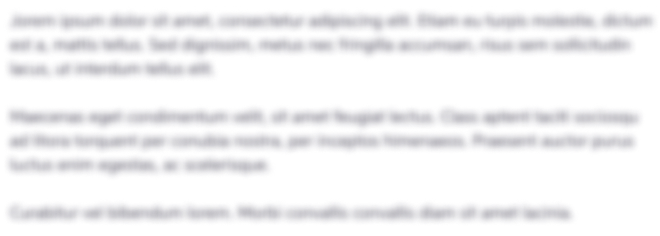
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started