Question
Type in C++ programming! struct definitions For this lab, you will get more practice working with C pointers, memory allocation, and recursive struct definitions. This
Type in C++ programming!
- struct definitions
For this lab, you will get more practice working with C pointers, memory allocation, and recursive struct definitions. This will be a continuation of Lab 05 and will also allow you to complete part of Program 05.
Again you are working with the following node definition:
// a recursive data type definition which
// serves as the nodes of a list
typedef struct node
{
int data;
struct node* next;
} node;
And it is assumed that in Lab 05: you wrote the following three functions:
// create an empty list - set *node = NULL
void create_list(node** head);
// add a new node to the front of the list
void add(node* *head, node* new_node);
// return the number of nodes in the list
int list_len(const node* head);
Finally, in case you have not successfully written a free_list function: one is given here
// free the entire list and set *head = NULL
void free_list(node* *head)
{
if (*head)
{
free_list( &((*head)->next) );
free(*head);
*head = NULL;
}
}
- Your job in this lab is to modify your list.h and/or list.c files (from Lab 05) such that:
- The function add will now add the new node to the existing list such that the list is left in ascending order, based on the values of the node's data components. Note: carefully consider the cases of: empty list; list of length one; and lists of length two or more.
- Integrate the given free_list function
- Your code works with the given main.c file
- You are also given the contents of a new main.c file (which also may not be modified):
#include
#include
#include "list.h"
int main(int args, char* argv[])
{
node* head;
// create an empty list
create_list(&head);
// add some nodes to the list
node* new_node;
int i;
for (i = 1; i
{
// create a new node
new_node = (node*)malloc(sizeof(node));
// set its data field to a random number [0, 100)
new_node->data = rand() % 100;
// its next field is set in the add function
// as well as adjusting the head pointer
add(&head, new_node);
}
// make copy of head for traversal
node* my_head = head;
// cheap print list
while (my_head)
{
printf("data = %d ", my_head->data);
my_head = my_head->next;
}
// free all of the nodes left in the list
free_list(&head);
return 0;
}
Recursive struct definitions For this lab, you will get more practice working with C pointers, memory allocation, and recursive struct definitions. This will be a continuation of Lab 05 and will also allow you to complete part of Program 05. Again you are working with the following node definition: // a recursive data type definition which // serves as the nodes of a list typedef struct node int data; struct node* next; } node; And it is assumed that in Lab 05: you wrote the following three functions: // create an empty list - set *node = NULL void create_list (node** head); // add a new node to the front of the list void add (node* *head, node* new_node); // return the number of nodes in the list int list_len (const node* head); Finally, in case you have not successfully written a free_list function: one is given here // free the entire list and set *head = NULL void free_list (node* *head) if ("head) free_list(&(("head) ->next)); free ("head); "head = NULL; Your job in this lab is to modify your list.h and/or list.c files (from Lab 05) such that: 1. The function add will now add the new node to the existing list such that the list is left in ascending order, based on the values of the node's data components. Note: carefully consider the cases of: empty list: list of length one; and lists of length two or more. 2. Integrate the given free_list function 3. Your code works with the given main.c file You are also given the contents of a new main.c file (which also may not be modified): #includeStep by Step Solution
There are 3 Steps involved in it
Step: 1
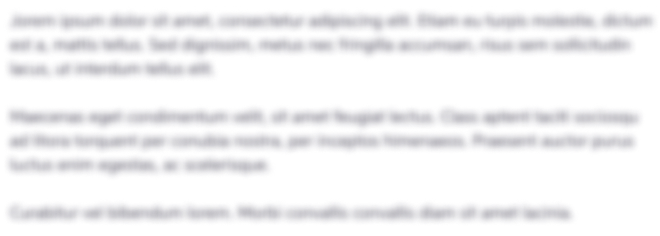
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started