Question
TypeError: 'int' object is not callable class MedianMaintainHeap: class MaxHeap: def __init__(self): self.H = [None] self.size = 0 def size(self): return len(self.H)-1 def __repr__(self): return
TypeError: 'int' object is not callable class MedianMaintainHeap:
class MaxHeap:
def __init__(self):
self.H = [None]
self.size = 0
def size(self):
return len(self.H)-1
def __repr__(self):
return str(self.H[1:])
def satisfies_assertions(self):
for i in range(2, len(self.H)):
assert self.H[i] <=self.H[i//2], f'Maxheap property fails at position {i//2},parent elt: {self.H[i//2]}, child elt: {self.H[i]}'
def max_element(self):
return self.H[1]
def bubble_up(self, index):
# your code here
assert index >= 1
if index == 1:
return
parent_index = index // 2
if self.H[parent_index] >self.H[index]:
return
else:
self.H[parent_index],self.H[index] = self.H[index], self.H[parent_index]
self.bubble_up(parent_index)
def bubble_down(self, index):
# your code here
assert index >= 1 and index
rchild_index = 2 * index + 1
# set up the value of left child to theelement at that index if valid, or else make it +Infinity
lchild_value = self.H[lchild_index] iflchild_index < len(self.H) else -float('inf')
# set up the value of right child tothe element at that index if valid, or else make it +Infinity
rchild_value = self.H[rchild_index] ifrchild_index < len(self.H) else -float('inf')
# If the value at the index is lessthanor equal to the maximum of two children, then nothing else todo
if self.H[index] >=max(lchild_value, rchild_value):
return
# Otherwise, find the index and valueof the smaller of the two children.
# A useful python trick is tocompare
max_child_value, max_child_index = max((lchild_value, lchild_index), (rchild_value, rchild_index))
# Swap the current index with the leastof its two children
self.H[index], self.H[max_child_index]= self.H[max_child_index], self.H[index]
# Bubble down on the maximum childindex
self.bubble_down(max_child_index)
# Function: insert
# Insert elt into minheap
# Use bubble_up/bubble_down function
def insert(self, elt):
# your code here
self.H.append(elt)
self.size = self.size + 1
i = self.size
self.bubble_up(i)
# Function: delete_max
# delete the largest element in the heap. Usebubble_up/bubble_down
def delete_max(self):
# your code here
if self.size <= 0:
return None
elif self.size == 1:
self.size = 0
return self.size +1
else:
# Get root of the heap(The min value of the heap)
minVal = self.H[1]
# 'MinHeap' object has noattribute 'heap'
# self.heap[1] =self.H[self.size]
# so changing above lineto avoid error
# Move the last value ofthe heap to the root
self.H[1] =self.H[self.size]
# Decrease the size ofthe heap
self.size = self.size -1
# Pop the last valuesince a copy was set on the minVal
self.H.pop()
# Bubble down the root(value at index 1) to keep the heap property
# Not bubble_up
i = 1
self.bubble_down(i) ##Check min value
# return the value
return minVal
class MedianMaintainingHeap: def __init__(self): self.hmin = MinHeap() self.hmax = MaxHeap() def satisfies_assertions(self): if self.hmin.size() == 0: assert self.hmax.size() == 0 return if self.hmax.size() == 0: assert self.hmin.size() == 1 return # 1. min heap min element >= max heap max element assert self.hmax.max_element() <= self.hmin.min_element(), f'Failed: Max element of max heap = {self.hmax.max_element()} > min element of min heap {self.hmin.min_element()}' # 2. size of max heap must be equal or one lessthan min heap. s_min = self.hmin.size() s_max = self.hmax.size() assert (s_min == s_max or s_max == s_min -1 ), f'Heap sizes are unbalanced. Min heap size = {s_min} and Maxheap size = {s_max}' def __repr__(self): return 'Maxheap:' + str(self.hmax) + ' Minheap:'+str(self.hmin) def get_median(self): if self.hmin.size() == 0: assert self.hmax.size() == 0, 'Sizes are not balanced' assert False, 'Cannot ask for median from empty heaps' if self.hmax.size() == 0: assert self.hmin.size() == 1, 'Sizes are not balanced' return self.hmin.min_element() # your code here if self.hmax.size() == self.hmin.size(): median = (self.hmax_element() + self.hmin_element()) else: median = self.hmin.min_element() return median # function: balance_heap_sizes # ensure that the size of hmax == size of hmin or size of hmax +1 == size of hmin # If the condition above does not hold, move the max element from max heap into the min heap or # vice versa as needed to balance the sizes. # This function could be called from insert/delete_median methods def balance_heap_sizes(self): # your code here if (self.hmin.size() > self.hmax.size() == self.hmin.size() + 1): self.hmax.insert(self.hmin.min_element()) self.hmin.delete_min() self.hmax.bubble_down(self.hmax.size()) return elif (self.hmin.size() < self.hmax.size() == self.hmin.size()): self.hmax.insert(self.hmin.max_element()) self.hmin.delete_max() self.hmin.bubble_down(self.hmin.size()) return else: return def insert(self, elt): # Handle the case when either heap is empty if self.hmin.size() == 0: # min heap is empty -- directly insert into min heap self.hmin.insert(elt) return if self.hmax.size() == 0: # max heap is empty -- this better happen only if min heap has size 1. assert self.hmin.size() == 1 if elt > self.hmin.min_element(): # Element needs to go into the min heap current_min = self.hmin.min_element() self.hmin.delete_min() self.hmin.insert(elt) self.hmax.insert(current_min) # done! else: # Element goes into the max heap -- just insert it there. self.hmax.insert(elt) return # Now assume both heaps are non-empty # your code here if (elt > self.hmin.min_element()): self.hmin.insert(elt) else: self.hmax.insert(elt) self.balance_heap_sizes() return def delete_median(self): self.hmax.delete_max() self.balance_heap_sizes()
m = MedianMaintainingHeap()
print('Inserting 1, 5, 2, 4, 18, -4, 7, 9')
m.insert(1)
print(m)
print(m.get_median())
m.satisfies_assertions()
assert m.get_median() == 1, f'expected median = 1, your codereturned {m.get_median()}'
m.insert(5)
print(m)
print(m.get_median())
m.satisfies_assertions()
assert m.get_median() == 3, f'expected median = 3.0, your codereturned {m.get_median()}'
m.insert(2)
print(m)
print(m.get_median())
m.satisfies_assertions()
assert m.get_median() == 2, f'expected median = 2, your codereturned {m.get_median()}'
m.insert(4)
print(m)
print(m.get_median())
m.satisfies_assertions()
assert m.get_median() == 3, f'expected median = 3, your codereturned {m.get_median()}'
m.insert(18)
print(m)
print(m.get_median())
m.satisfies_assertions()
assert m.get_median() == 4, f'expected median = 4, your codereturned {m.get_median()}'
m.insert(-4)
print(m)
print(m.get_median())
m.satisfies_assertions()
assert m.get_median() == 3, f'expected median = 3, your codereturned {m.get_median()}'
m.insert(7)
print(m)
print(m.get_median())
m.satisfies_assertions()
assert m.get_median() == 4, f'expected median = 4, your codereturned {m.get_median()}'
m.insert(9)
print(m)
print(m.get_median())
m.satisfies_assertions()
assert m.get_median()== 4.5, f'expected median = 4.5, your codereturned {m.get_median()}'
print('All tests passed: 15 points')
Inserting 1, 5, 2, 4, 18, -4, 7, 9
---------------------------------------------------------------------------TypeError Traceback (most recent call last) in 2 print('Inserting 1, 5, 2, 4, 18, -4, 7, 9') 3 ----> 4 m.insert(1) 5 print(m) 6 print(m.get_median()) in insert(self, elt) 57 def insert(self, elt): 58 # Handle the case when either heap is empty---> 59 if self.hmin.size() == 0: 60 # min heap is empty -- directly insert into min heap 61 self.hmin.insert(elt)TypeError: 'int' object is not callable
Step by Step Solution
There are 3 Steps involved in it
Step: 1
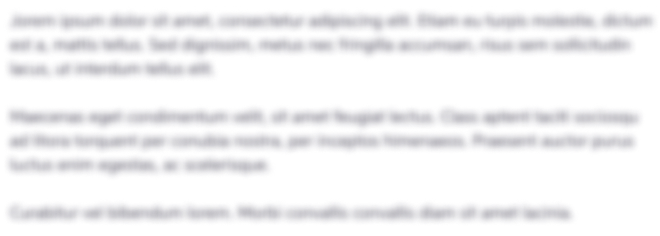
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started