Question
Unit 4 Assignment 2: Coding Project (Bubble Sort) Scenario You are an instructor and have 10 students in a class. You need to sort their
Unit 4 Assignment 2: Coding Project (Bubble Sort)
Scenario
You are an instructor and have 10 students in a class. You need to sort their final grades from highest to lowest for your gradebook. You also need to post them from lowest to highest for a report to the administration department. Create an array called studentGrades and populate the array with the following grades: 65, 95, 75, 55, 56, 90, 98, 88, 97, and 78. Implement a bubble sort that will provide the information in both highest to lowest and lowest to highest order.
Create one method called sortArrayDes(). Implement a bubble sort algorithm that will sort from highest to lowest.
Create a second method called sortArrayAsc(). Implement a bubble sort algorithm that will sort from lowest to highest.
Create a third method called printArray, which will display the results of both sorts.
Expected Output
Bubble Sort Descending.
98 97 95 90 88 78 75 65 56 55
Bubble Sort Ascending.
55 56 65 75 78 88 90 95 97 98
I have been trying several different methods from my text books, videos, and online searches but I can't figure out where I'm going wrong. I use C#; This is what I have so far:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks;
namespace ConsoleApp46 { class Program { public enum SortType { Ascending, Descending } public static void BubbleSort(int[] studentGrades, SortType sortOrder) { int i; int j; int temp;
if (studentGrades == null) { return; }
for (i = studentGrades.Length - 1; i >= 0; i--) { for (j = 1; j <= i; j++) { bool swap = false; switch (sortOrder) { case SortType.Ascending : swap = studentGrades[j - 1] > studentGrades[j]; break;
case SortType.Descending : swap = studentGrades[j - 1] > studentGrades[j]; break; } if (swap) { temp = studentGrades[j - 1]; studentGrades[j - 1] = studentGrades[j]; studentGrades[j] = temp; } } Console.Write(studentGrades + " ");
} } static void Main(string[] args) {
int[] studentGrades = { 65, 95, 75, 55, 56, 90, 98, 88, 97, 78 }; } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
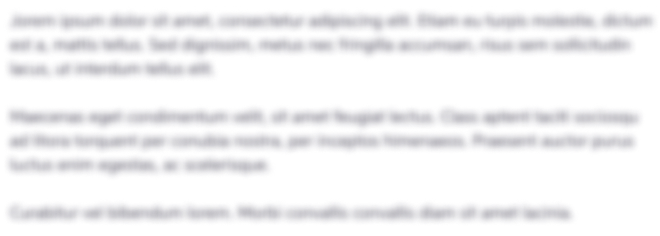
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started