Question
Unix systems keep track of which users log in, when they do it and where they do it from. To see an example of this,
Unix systems keep track of which users log in, when they do it and where they do it from.
To see an example of this, try out the last command on your Unix system: [Loki] :~:> last
This will give you a listing of all of the people who have logged in to the system for the current month, with the
most recent logins reported at the beginning of the list. To page through the results using the less
command in Unix, use this command instead:
[Loki] :~:> last | less This pipes the output through the less command, which allows you to page through the output and use vim -like control to scroll forward and backward through the output.
To process the previous months logins,
try this:
[Loki] :~:> last f /var/log/wtmp.1 | less
A typical line of output looks something like one of the following two lines:
rfulkers pts/46 137.48.186.243 Thu Sep 17 11:52 - 13:58 (02:06)
rfulkers pts/56 pc-185-13.ist.un Thu Sep 17 11:11 - 16:37 (05:25)
As you can see, there are 7 logical fields contained within the output of the last command, and there are 10
actual pieces of information.
The logical fields are the TRUNCATED_ACCOUNT, TERMINAL, HOSTNAME,
DATE, LOGIN, LOGOUT, and DURATION.
The DATE field is made up of 3 separate pieces of information
(DAYOFWEEK, MONTH, DATE), and there is a dash between the LOGIN and LOGOUT fields.
The TERMINAL field will likely be of the form pts/# or pts/##, though there may be variations. If you would like to investigate the
last command more, type man last at the Unix prompt. One of the shortcomings of the last command is that there is no summation or reporting facility to tell someone using the command an overall summary of how long someone has used the system over the course of a month or how many times the person connected. For a company that might charge for hourly usage of their system, it would be helpful if there was a utility that would generate these kinds of basic statistics as well as provide a listing of all logins for a specific account. For this assignment, youll be writing a program to display summary information about a single user's account on the system. Here are the specifications for your program; make sure you read and understand all requirements before proceeding:
1. Your program must take one command-line argument, which will be the name of the account to
summarize. If no command-line argument is given, display a usage statement and exit the program. You
can assume that the account exists; no valiation of this fact is necessary.
2. Your program will then process the output from last and display a summary of that accounts activity including the following information: account name, how many logins were executed, and the time inHH:MM format (not DD+HH:MM).
1. You must display each login entry, prefaced by a login # (sequential numbers starting at 1).
See the final page for a complete example of the output.
2. Make sure your final output follows this formatting, where the time should be in the format HH:MM, where HH and MM (hours and minutes) should both be two digits in length, including leading 0s if necessary.
The user's full account name, logins and time should each be displayed on separate lines in that order:
FULL_ACCOUNT_NAME
NUMBER_OF_LOGINS
TOTAL_TIME_IN_HH:MM
Here are some useful observations and Perl tools you can use to implement this program's solution.
To grab the output of an external program, try the following small program. Notice the use of backticks instead of single quotes. This distinction is important. The following example grabs the output of the finger command:
#!/usr/bin/env perl
use Modern::Perl;
my @capture = `finger`;
# BACKTICKS! NOT SINGLE QUOTES!
# You would want to use something similar in your program
# process every line of output and print it out
For my $line(@capture){
Print "Here's a line: $line ";
}
The last field in each entry of the output of the last command looks like (DD+HH:MM), where DD is a number of days for that session(one or two digits), HH is the number of hours for that session (always two digits) and MM is the number of minutes for that session (also always two digits). The DD+ portion is
optional and only appears if the person was logged in for more than 24 hours.
The last field may also contain the text still logged in if the user is, indeed, still logged in.
Yo u d o n o t need to calculate a duration for these entries, but they must count toward the total count of logins.
Write a program that processes a command-line argument and produces a usage statement if no command-line argument is given.
Write a program with Perl that processes an external Unix command.
Use regular expressions to identify and extract relevant pieces of a string of text.
Grabbing Output
Format of the duration the user was logged in
Perl
The only input into the program will come from the command line argument passed into the program.
There won't be any use of the <> operator in this program.
Remember to use use Modern::Perl; in your program.
Remember to use the correct shebang line in your program.
How will you go about grabbing all of the entries that match and then process them?
You may assume that the account provided on the command line is valid and that the account exists.
Whether or not that individual has any logins is a condition that should be handled appropriately.
You will need to make sure that you handle accounts that have the D+HH:MM format listed for their logins.
To get a listing of accounts that match this, use the Unix grep command like this: [Loki] :~:> last | grep +
This will give you a listing of accounts that have entries in the D+HH:MM format that you can then test in your program.
Make sure to handle if the command-line argument that is provided is longer than 8 characters. last
only records the first 8 characters of a user's account, so you'll need to handle if someone provides bartsimpson for the command-line argument and locate only bartsimp entries from the output of last.
You must have an Honor Pledge on file for the course for the program to be graded.
You must have complete header documentation as outlined in the Course Materials section of Blackboard.
You must use at least one regular expression in your program, since this assignment is meant to reinforce
the material that's recently been covered in class.
Points will be deducted if your program generates warnings with the
use Modern::Perl; pragma to a maximum of 5 points, 1 point per warning.
See the output at the end of this assignment or run the sample program on Loki for an actual example.
Your hours and minutes must be in two-digit format; in other words, single digits should have a leading
0 to pad the results, like 00:00.
Make sure to stop the program if no command-line argument is given or too many command line
arguments are provided.
Provide a usage statement if no command-line argument is given, and make
sure to use $0 in your usage statement to produce the correct output.
Do not process partial command-line arguments, such as if the program were run with
./lastsummary.pl art, it shouldn't bring up a summary of all logins that contain the text art.
The only listings that should match would be a username of art, so no listings for bartsimp, for instance, should show up.
Do not convert the time summary to days, hours and minutes.
Make sure your total time output is only in
total hours and minutes.
Do not use the last account format of last or last account | grep account to get just information for a particular user.
Your program will not be graded if you use either of these methods to
obtain your user list.
One of the goals of this program is to practice matching with regexes.
Your output capture statement should look like this:
my@lastoutput=`last`;
You can run a sample to check your output results against on Loki with the following command:
~rfulkerson/samples/lastsummary
Perl
Sample Run
username@Loki:~$ lastsummary joe smith
Usage: lastsummary login
username@Loki:~$ lastsummary
Usage: lastsummary login
username@Loki:~$ lastsummary noone
Here is a listing of the logins for noone:
Here is a summary of the time spent on the system for noone:
noone
0
00:00
Sample Run
username@Loki:~$ lastsummary rfulkerson
Here is a listing of the logins for rfulkerson:
1. rfulkers pts/3 pki174b-01.ist.u Tue Sep 7 09:49 still logged in
2. rfulkers pts/7 ip72-206-101-146 Mon Sep 6 20:26 - 21:18 (00:51)
3. rfulkers pts/5 ip72-206-101-146 Mon Sep 6 20:24 - 21:18 (00:53)
4. rfulkers pts/3 vulcan.ist.unoma Sat Sep 4 19:51 - 20:09 (00:18)
5. rfulkers pts/1 ip72-206-101-146 Fri Sep 3 07:37 - 21:29 (13:51)
6. rfulkers pts/6 137.48.177.164 Thu Sep 2 11:54 - 13:17 (01:23)
7. rfulkers pts/3 pki174b-01.ist.u Thu Sep 2 09:26 - 11:48 (02:22)
Here is a summary of the time spent on the system for rfulkerson:
rfulkerson
7
19:38
Sample Run
username@Loki:~$ lastsummary mtucker
Here is a listing of the logins for mtucker:
1. mtucker pts/2 ip68-13-82-64.om Fri Sep 3 07:54 - 20:02 (2+12:07)
2. mtucker pts/0 ip68-13-82-64.om Thu Sep 2 19:45 - 20:15 (00:30)
3. mtucker pts/1 137.48.208.181 Thu Sep 2 13:39 - 16:50 (03:11)
Here is a summary of the time spent on the system for mtucker:
mtucker
3
63:48
Step by Step Solution
There are 3 Steps involved in it
Step: 1
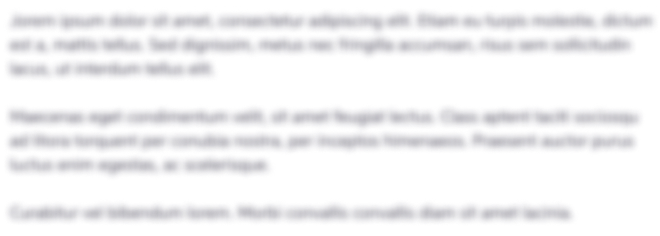
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started