Question
Use C++ Task 1: Create a structure called employee that contains two members: an employee number (type int) and the employees compensation (in dollars; type
Use C++
Task 1: Create a structure called employee that contains two members: an employee number (type int) and the employees compensation (in dollars; type float). Ask the user to fill in this data for three employees, store it in three variables of type struct employee, and then display the information for each employee.
Task 2: Create an employee class, basing it on Task 1. The member data should comprise an int for storing the employee number and a float for storing the employees compensation. Member functions should allow the user to enter this data and display it. Write a main() that allows the user to enter data for three employees and display it.
Task 3: Create a structure of type date that contains three members: the month, the day of the month, and the year, all of type int. (Or use day-month-year order if you prefer.) Have the user enter a date in the format 12/31/2001, store it in a variable of type struct date, then retrieve the values from the variable and print them out in the same format.
Task 4: Start with the date structure in Task 3 and transform it into a date class. Its member data should consist of three ints: month, day, and year. It should also have two member functions: getdate(), which allows the user to enter a date in 12/31/02 format, and showdate(), which displays the date.
Task 5: In ocean navigation, locations are measured in degrees and minutes of latitude and longitude. Thus if youre lying off the mouth of Papeete Harbor in Tahiti, your location is 149 degrees 34.8 minutes west longitude, and 17 degrees 31.5 minutes south latitude. This is written as 14934.8 W, 1731.5 S. There are 60 minutes in a degree. (An older system also divided a minute into 60 seconds, but the modern approach is to use decimal minutes instead.) Longitude is measured from 0 to 180 degrees, east or west from Greenwich, England, to the international dateline in the Pacific. Latitude is measured from 0 to 90 degrees, north or south from the equator to the poles. Create a class angle that includes three member variables: an int for degrees, a float for minutes, and a char for the direction letter (N, S, E, or W). This class can hold either a latitude variable or a longitude variable. Write one member function to obtain an angle value (in degrees and minutes) and a direction from the user, and a second to display the angle value in 17959.9 E format. Also write a three-argument constructor. Write a main() program that displays an angle initialized with the constructor, and then, within a loop, allows the user to input any angle value, and then displays the value. You can use the hex character constant \xF8, which usually prints a degree () symbol.
Task 6: Create a class that includes a data member that holds a serial number for each object created from the class. That is, the first object created will be numbered 1, the second 2, and so on. To do this, youll need another data member that records a count of how many objects have been created so far. (This member should apply to the class as a whole; not to individual objects. What keyword specifies this?) Then, as each object is created, its constructor can examine this count member variable to determine the appropriate serial number for the new object. Add a member function that permits an object to report its own serial number. Then write a main() program that creates three objects and queries each one about its serial number. They should respond I am object number 2, and so on.
Task 7: Use inheritance and classes to represent a deck of playing cards. Create a Card class that stores the suit (e.g. Clubs, Diamonds, Hearts, Spades), and name (e.g. Ace, 2, 10, Jack) along with appropriate accessors, constructors, and mutators.
Next, create a Deck class that stores a vector of Card objects. The default constructor should create objects that represent the standard 52 cards and store them in the vector. The Deck class should have functions to:
Print every card in the deck
Shuffle the cards in the deck. You can implement this by randomly swapping every card in the deck.
Add a new card to the deck. This function should take a Card object as a parameter and add it to the vector.
Remove a card from the deck. This removes the first card stored in the vector and returns it.
Sort the cards in the deck ordered by name.
Next, create a Hand class that represents cards in a hand. Hand should be derived from Deck. This is because a hand is like a more specialized version of a deck; we can print, shuffle, add, remove, or sort cards in a hand just like cards in a deck. The default constructor should set the hand to an empty set of cards.
Finally, write a main function that creates a deck of cards, shuffles the deck, and creates two hands of 5 cards each. The cards should be removed from the deck and added to the hand. Test the sort and print functions for the hands and the deck.
Finally, return the cards in the hand to the deck and test to ensure that the cards have been properly returned. You may add additional functions or class variables as desired to implement your solution.
Task 8: Define a class named Payment that contains a member variable of type float that stores the amount of the payment and appropriate accessor and mutator methods. Also create a member function named paymentDetails that outputs an English sentence that describes the amount of the payment.
Next define a class named CashPayment that is derived from Payment. This class should redefine the paymentDetails function to indicate that the payment is in cash. Include appropriate constructor(s).
Define a class named CreditCardPayment that is derived from Payment. This class should contain member variables for the name on the card, expiration date, and credit card number. Include appropriate constructor(s). Finally, redefine the paymentDetails function to include all credit card information in the printout.
Create a main method that creates at least two CashPayment and two CreditCardPayment objects with different values and calls paymentDetails for each.
Task 9: Listed below is code to play a guessing game in which two players attempt to guess a number. Your task is to extend the program with objects that represent either a human player or a computer player.
bool checkForWin(int guess, int answer) { if (answer == guess) { cout << "You're right! You win!" << endl; return true; } else if (answer < guess) cout << "Your guess is too high." << endl; else cout << "Your guess is too low." << endl; return false;
} void play(Player &player1, Player &player2) { int answer = 0, guess = 0; answer = rand() % 100; bool win = false; while (!win) { cout << "Player 1's turn to guess." << endl; guess = player1.getGuess(); win = checkForWin(guess, answer); if (win) return; cout << "Player 2's turn to guess." << endl; guess = player2.getGuess(); win = checkForWin(guess, answer); } }
The play function takes as input two Player objects. Define the Player class with a virtual function named getGuess(). The implementation of Player::getGuess() can simply return 0.
Next, define a class named HumanPlayer derived from Player. The implementation of HumanPlayer::getGuess() should prompt the user to enter a number and return the value entered from the keyboard. Next, define a class named ComputerPlayer derived from Player.
The implementation of ComputerPlayer::getGuess() should randomly select a number from 0 to 100. Finally, construct a main function that invokes play(Player &player1, Player &player2)with two instances of a HumanPlayer (human vs. human), an instance of a HumanPlayer and ComputerPlayer (human vs. computer), and two instances of ComputerPlayer (computer vs. computer).
Step by Step Solution
There are 3 Steps involved in it
Step: 1
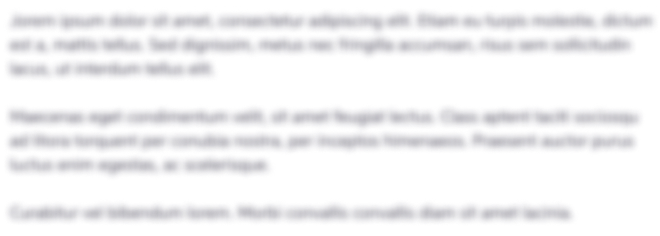
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started