Question
Use C++ write this Learning Objectives: - implementing an application using if/else and loops - introductory use of file input and output - validating user
Use C++ write this
Learning Objectives:
- implementing an application using if/else and loops
- introductory use of file input and output
- validating user input and formatting output
General Description:
Write a program that simulates the action of a gas pump. At startup, the pump reads a file that contains
the number of gallons available and price per gallon of three octanes of gas (High, Medium and Low).
This information is then printed on the screen.
For each customer, the pump starts by displaying a logo and asking the customer if they are a rewards
customer or not. If yes, the rewards number is entered and a per-gallon discount is determined.
The customer then selects an octane (H/M/L) and enters a number of gallons, or indicates a fill up. For
a fill up, an estimate is simulated as a random number of gallons between 1 and 8. If not enough gas is
available to satisfy the customers request, the number of gallons is changed to the amount remaining in
the pump and the customer is informed.
The pump then simulates dispensing gasoline by printing the amount dispensed on the screen after each
gallon is dispensed, reporting approximately every two seconds, until the number of gallons indicated
has been dispensed.
Finally, a receipt is printed on the screen and, after a pause, the process repeats for the next customer.
The pump technician can shut down the pump by entering shutdown to the question Are you a
rewards customer? This is a corporate secret and not to be revealed to the customer. On shutdown,
the pump reports the gallons of each octane currently available on the screen, then writes this data
along with the current prices per gallon to a text file before ending the program.
Specifications:
General Formatting:
- all floating point values should be displayed and written with 2 digits past the decimal point
- when prompted for input, there should be at least one space after the prompt so user input is
not jammed up with the prompt. Ex:
Enter your age: 19 this
Enter your age:19 not this!
2
Files:
- Input File: named pumpin.txt
The first line contains three numbers, the number of gallons in the pumps tank of High Octane,
Medium Octane and Low Octane, in that order.
The second line contains the price per gallon for each octane in the same order.
Example: (we are very low on High Octane in this exampleonly 8.9 gallons
left!):
8.9 789.3 845.6
2.45 2.25 2.05
- Output File: named pumpout.txt
Contains the same information and format as the input file, reflecting the
data at the time the pump is shut down.
- Suggestion: Add these two files to the Resource Files section of the Solution
Explorer. This is not required, but may make dealing with the files easier.
On Startup:
The program should read the six values from the input file and display them formatted as shown in this
example. The pumps tanks hold a
maximum of 999.99 gallons each,
and the price of gas per gallon will
be 99.99 or less.
If the program is unable to open the input file, it should display a message Unable to read input file,
pause, and exit the program. You may test this by
(temporarily) changing the name of the file to
something else.
Asking if rewards customer:
Display a logo as shown.
Make up your own name of
the gas station, and include
your name somewhere in the
logo.
Ask the user Are you a rewards customer? (Y/N): Valid responses are Y,y,N,n and shutdown. When
an invalid response is entered, display Please enter Y or N.and repeat the question. Repeat this until
the customer enters a valid answer.
Design Hint: note that at this point, one of two options will happen: the program continues with a
customer sale, or the shut down is performed and the program ends. This segment of code acts as
Input for a Sentinel-Controlled Loop, where the Sentinel Value is shutdown. See more below.
3
Asking Rewards Number and Calculating PPG (Price Per Gallon) Discount:
If this customer is a rewards customer, ask the customer Enter customer rewards number: You may
assume they will enter an integer number.
Calculate the PPG discount as the modulus of the customer number by 10 and add 1. This results in a
number 1-10. Divide this result by 100 to get the discount in cents. Example:
customer number = 2135679
2135679 % 10 is 9
9 + 1 is 10
10 / 100 = 0.10 = PPG discount
Of course, if the customer is NOT a rewards customer, do not ask them to enter a number and the PPG
Discount is 0.00.
Whether a rewards customer or not, print the PPG Discount as shown in the examples. The $ should line
up with the customers input for both Are you a and Enter rewards.. as shown. Also, print a blank
line before displaying the prices per gallon in the next section.
Displaying Prices Per Gallon:
The prices are the standard prices, read from the input file,
minus the PPG Discount calculated. Format the price list as
shown here. There should be a blank line above and another
below the price list.
Selecting an Octane:
Ask the user Select octane (H/M/L): as shown in the
example. Valid entries are H,h,M,m,L, and l. When the user
enters an invalid entry, print Please enter H, M or L.
Repeat this until the user enters a valid entry. After a valid
entry is entered, print a blank line.
Getting number of gallons:
Ask the user Enter number of gallons (-1 to Fill it up): You may assume the customer will
enter an integer or floating point number. When -1 (or any number less than 0) is entered, simulate the
number of gallons as a random number between 1 and 8. Use the rand() function, which returns a
random number (use modulus to get a number 0 to 7, then add 1). Report this number as detected by a
sensor with the message: Sensor
reports g gallons needed to fill
up, where g is the random number of
gallons calculated.
4
Check for empty tanks:
It is possible this pump is almost empty for some octane of gasoline. The amount in each tank is read
when the pump starts. When gasoline is dispensed to a customer, the amount dispensed is subtracted
from the tanks current total.
If the number of gallons requested is more than is available, give the customer all the remaining supply
by changing the number of gallons requested to the amount left in the pumps tank (emptying the tank).
Print Sorry, our tank is nearly empty. We only have g gallons available. Note that if the tank
is empty, the amount requested will be 0 gallons and the pump will continue delivering 0 gallons at 0
cost.
Simulate Pumping Gas:
A message on the total number of gallons pumped so far is printed every 2 seconds. The pump pumps
gallon every two seconds. Note that
the last message should report the
original number of gallons requested,
and so may not be an equal gallon.
One way to get a Visual Studio C++
program to wait (this may not work
in other versions of C++) is to use the
Sleep(n) function, where n is the
number of milliseconds (note the
capital S). To use this function, the program must #include
Start with the message Pumping gas... with a blank line before and after the set of messages.
Printing a receipt:
Format the receipt as shown here, including lining up the values as shown. The octane value printed
should NOT be simply H, M or L as originally entered by the user, but should be fully-spelled out as High,
Medium or Low. The decimal points for the
PPG and total cost should always line up. You
may assume no one purchase will exceed
$999.99.
Feel free to change the text on the Thank You
line, but it must have a Thank You line.
There should be a pause after printing the receipt.
After the pause, the process should repeat for the next customer, staring with printing the logo.
5
Shut Down:
When the user enters shutdown when asked Are you a rewards customer, the pump performs the
following shut down operations and the program ends.
It should print the current
tank readings (the number
of gallons in each of the
three tanks) as shown in
the example.
It should also write the
tank readings on one line, and the three price-per-gallon values on a second line, to a file called
pumpout.txt See details on files above.
Finally, the program prints Pump shut down and then does a pause before the program ends
Step by Step Solution
There are 3 Steps involved in it
Step: 1
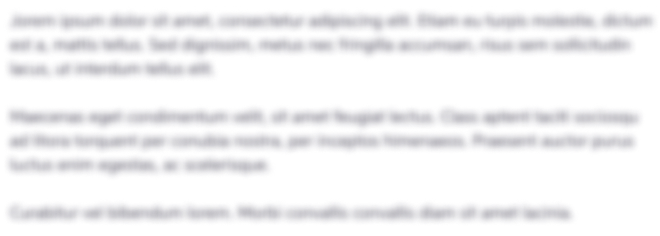
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started