Question
USE CPP A stack that does not allow duplicates The ADT allows only a single copy of an object in the stack. Stack is unchanged
USE CPP
A stack that does not allow duplicates The ADT allows only a single copy of an object in the stack. Stack is unchanged if a duplicate object is being added to it. - support the usual peek(), pop(), push(newElement) methods - Also, the following operations: -- find(x) that finds the location of an element x in the stack. The first element in the stack has location number one. The second pushed element has location number two and so on. -- peek1() returns the first pushed element
Data structure: Use circular linked data.
Specify: proper preconditions and postconditions of each method
Exceptions handling: - The stack has a limit. The stack throws a user exception whenever a client push an item to a full stack. The size of the stack is arbitrary. - Also, throws a user exception whenever peek or pop operations being called on an empty stack
Design Decisions: - Separate the the ADT specification from its implementation using cpp Interface - Make the ADT generic
Testing: - write in a main() function sufficient test code to test all the methods that the ADT support - check corner cases; i.e. pushing to full stack, peeking into an empty stack, pushing a duplicate item, ..etc
Time efficiency: - peek1() has to run in a constant time O(1)
Naming Convention: - Class (including interfaces and user exceptions) names are written in UpperCamelCase. - Methods are written lowerCamelCase. - Constant names use CONSTANT_CASE: all uppercase letters, with each word separated from the next by a single underscore - Local variable (including method parameters) names are written in lowerCamelCase Diagram
Step by Step Solution
There are 3 Steps involved in it
Step: 1
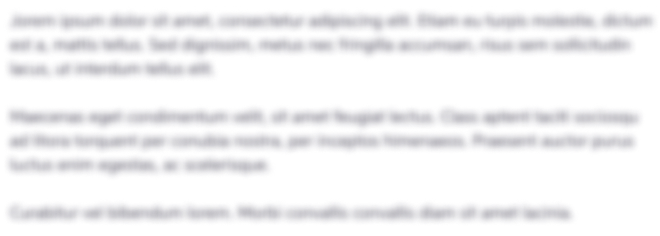
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started