Question
USE JAVA. Write a computer program that prompts the user for one number, n for the number of items in the array to sort, and
USE JAVA. Write a computer program that prompts the user for one number, n for the number of items in the array to sort, and create and sort 1000 different arrays of this size timing the run to get an average time to sort an array of this size. Then do the following:
Initiate a variable running_time to 0
Create a for loop that iterates 1000 times.
In the body of the loop,
Create an array of n random integers
Get the time and set this to start-time (notice the sort is started after each array is built. You want to time the srt process only). You will have to figure out what the appropriate command is in the programming language you are using to find the time (Important: Do not start the timer until after the array is created).
Use bubble sort to sort the array
Get the time and set this to end-time Subtract start-time from end-time and add the result to total_time
Once the program has run, note
The number of items sorted
The average running time for each array (total_time/1000)
Repeat the process using 500, 2500 and 5000 as the size of the array.
PLEASE FOLLOW THESE INSTRUCTIONS EXACTLY.
This is my code so far:
import java.util.Random; import java.util.Scanner; public class BubbleSort { public static void main(String args[]){ Scanner sc = new Scanner(System.in); System.out.println("Enter Array Size: "); int n = sc.nextInt(); //Randomly filling array with numbers o to 9999 inclusive int[] randomNumbers = new int[n]; Random rand = new Random(); for (int i = 0; i < randomNumbers.length; i++) { int j = rand.nextInt(10000); randomNumbers[i] = j; } //Printing numbers in array with their positions System.out.println("Before Bubble Sort "); for (int i = 0; i < randomNumbers.length; i++) { System.out.println("Position " + i + " : " + randomNumbers[i]); } //Bubble sort algorithm for (int c = 0 ; c < ( n - 1 ); c++) { for (int d = 0 ; d < n - c - 1; d++) { if (randomNumbers[d] > randomNumbers[d+1]) { //numbers in descending order //Swap elements int swap = randomNumbers[d]; randomNumbers[d] = randomNumbers[d+1]; randomNumbers[d+1] = swap; } } } //Printing result after bubble sort System.out.println("After Sorting Using Bubble Sort: "); for (int i = 0; i < randomNumbers.length; i++) { System.out.println("Position " + i + " : " + randomNumbers[i]); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
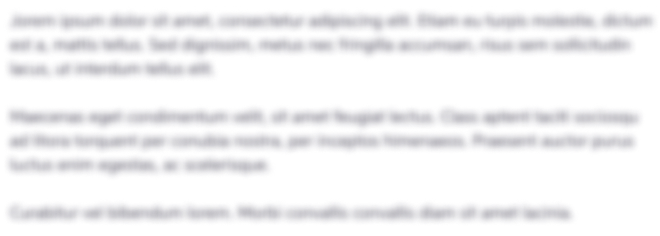
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started