Question
Use Python Please! Try to provide the right solution based on the info given below. Do not copy paste other solutions or I will rate
Use Python Please!
Try to provide the right solution based on the info given below. Do not copy paste other solutions or I will rate you down and report you.
LOGIC PY:
import itertools class Sentence(): def evaluate(self, model): """Evaluates the logical sentence.""" raise Exception("nothing to evaluate") def formula(self): """Returns string formula representing logical sentence.""" return "" def symbols(self): """Returns a set of all symbols in the logical sentence.""" return set() @classmethod def validate(cls, sentence): if not isinstance(sentence, Sentence): raise TypeError("must be a logical sentence") @classmethod def parenthesize(cls, s): """Parenthesizes an expression if not already parenthesized.""" def balanced(s): """Checks if a string has balanced parentheses.""" count = 0 for c in s: if c == "(": count += 1 elif c == ")": if count
def __repr__(self): return self.name def evaluate(self, model): try: return bool(model[self.name]) except KeyError: raise EvaluationException(f"variable {self.name} not in model") def formula(self): return self.name def symbols(self): return {self.name} class Not(Sentence): def __init__(self, operand): Sentence.validate(operand) self.operand = operand def __eq__(self, other): return isinstance(other, Not) and self.operand == other.operand def __hash__(self): return hash(("not", hash(self.operand))) def __repr__(self): return f"Not({self.operand})" def evaluate(self, model): return not self.operand.evaluate(model) def formula(self): return "" + Sentence.parenthesize(self.operand.formula()) def symbols(self): return self.operand.symbols() class And(Sentence): def __init__(self, *conjuncts): for conjunct in conjuncts: Sentence.validate(conjunct) self.conjuncts = list(conjuncts) def __eq__(self, other): return isinstance(other, And) and self.conjuncts == other.conjuncts def __hash__(self): return hash( ("and", tuple(hash(conjunct) for conjunct in self.conjuncts)) )
def __repr__(self): conjunctions = ", ".join( [str(conjunct) for conjunct in self.conjuncts] ) return f"And({conjunctions})" def add(self, conjunct): Sentence.validate(conjunct) self.conjuncts.append(conjunct) def evaluate(self, model): return all(conjunct.evaluate(model) for conjunct in self.conjuncts) def formula(self): if len(self.conjuncts) == 1: return self.conjuncts[0].formula() return " ".join([Sentence.parenthesize(conjunct.formula()) for conjunct in self.conjuncts]) def symbols(self): return set.union(*[conjunct.symbols() for conjunct in self.conjuncts]) class Or(Sentence): def __init__(self, *disjuncts): for disjunct in disjuncts: Sentence.validate(disjunct) self.disjuncts = list(disjuncts) def __eq__(self, other): return isinstance(other, Or) and self.disjuncts == other.disjuncts def __hash__(self): return hash( ("or", tuple(hash(disjunct) for disjunct in self.disjuncts)) ) def __repr__(self): disjuncts = ", ".join([str(disjunct) for disjunct in self.disjuncts]) return f"Or({disjuncts})" def evaluate(self, model): return any(disjunct.evaluate(model) for disjunct in self.disjuncts) def formula(self): if len(self.disjuncts) == 1: return self.disjuncts[0].formula() return " ".join([Sentence.parenthesize(disjunct.formula()) for disjunct in self.disjuncts]) def symbols(self): return set.union(*[disjunct.symbols() for disjunct in self.disjuncts]) class Implication(Sentence): def __init__(self, antecedent, consequent): Sentence.validate(antecedent) Sentence.validate(consequent) self.antecedent = antecedent self.consequent = consequent def __eq__(self, other): return (isinstance(other, Implication) and self.antecedent == other.antecedent and self.consequent == other.consequent) def __hash__(self): return hash(("implies", hash(self.antecedent), hash(self.consequent))) def __repr__(self): return f"Implication({self.antecedent}, {self.consequent})" def evaluate(self, model): return ((not self.antecedent.evaluate(model)) or self.consequent.evaluate(model)) def formula(self): antecedent = Sentence.parenthesize(self.antecedent.formula()) consequent = Sentence.parenthesize(self.consequent.formula()) return f"{antecedent} => {consequent}" def symbols(self): return set.union(self.antecedent.symbols(), self.consequent.symbols()) class Biconditional(Sentence): def __init__(self, left, right): Sentence.validate(left) Sentence.validate(right) self.left = left self.right = right def __eq__(self, other): return (isinstance(other, Biconditional) and self.left == other.left and self.right == other.right) def __hash__(self): return hash(("biconditional", hash(self.left), hash(self.right))) def __repr__(self): return f"Biconditional({self.left}, {self.right})" def evaluate(self, model): return ((self.left.evaluate(model) and self.right.evaluate(model)) or (not self.left.evaluate(model) and not self.right.evaluate(model))) def formula(self): left = Sentence.parenthesize(str(self.left)) right = Sentence.parenthesize(str(self.right)) return f"{left} {right}" def symbols(self): return set.union(self.left.symbols(), self.right.symbols()) def model_check(knowledge, query): """Checks if knowledge base entails query.""" def check_all(knowledge, query, symbols, model): """Checks if knowledge base entails query, given a particular model.""" # If model has an assignment for each symbol if not symbols: # If knowledge base is true in model, then query must also be true if knowledge.evaluate(model): return query.evaluate(model) return True else: # Choose one of the remaining unused symbols remaining = symbols.copy() p = remaining.pop() # Create a model where the symbol is true model_true = model.copy() model_true[p] = True # Create a model where the symbol is false model_false = model.copy() model_false[p] = False # Ensure entailment holds in both models return (check_all(knowledge, query, remaining, model_true) and check_all(knowledge, query, remaining, model_false)) # Get all symbols in both knowledge and query symbols = set.union(knowledge.symbols(), query.symbols()) # Check that knowledge entails query return check_all(knowledge, query, symbols, dict())
4-Queens Problem as a Logical Problem (40) In this part you are expected solve 4-Queens problem using propositional logic and the model checking approach. Please use the logic.py module encolsed with the homework. This is the same module that we discussed in the class. Below we export logic.py module. Note that the logic.py need to be at the same folder as this notebook from logic import In this question, we will have a propositional symbol dij (qij) for each possible placement of queen on row i and column j. qij is true when there is a queen on row i and column j. For example 9o2 (902) is true if we have a queen on the first row and third column. Note that the indexing starts at O. We have 16 symbols. # We define a symbol qij that is true when there is queen in row i and column symbols - 0 knowledge = And() for row in range(4): for col in range(4): symbols.append(Symbol(f"q{row}{col}')) Next, you will add statements regarding there will exactly one queen on each row, and exactly one queen on each column, and queens must not attack each other diagnoally to your knowledge base. You probably need to define these using for loops as there will be numerous sentences. I recommend checking the examples shown in the class. 3/5 12/20/21, 10:16 PM Assignment pynb-Colaboratory # Exactly one queen in row i # Exactly one queen in column] # At most one queen in each diagonal Finally you apply model checking to see which symbols are true or are not false. def check_knowledge(knowledge): for symbol in symbols: if model_check(knowledge, symbol): print(f"{symbol): YES") elif not model_check(knowledge, Not (symbol)): print("symbol): MAYBE") check_knowledge(knowledge) If you have correctly defined your knowledge base, this code should print 981: MAYBE 902: MAYBE 910: MAYBE 913: MAYBE 920: MAYBE 923: MAYBE 931: MAYBE 932: MAYBE In order to find a specific solution, we need to add the place of the knowledge base as well. knowledge.add(Symbol("q81")) check_knowledge(knowledge) If you have correctly defined your knowledge base you should see 901: YES 913: YES 4/5 Assignment4.ipynb. Colaboratory 12/20/21, 10:16 PM 920: YES 432: YESStep by Step Solution
There are 3 Steps involved in it
Step: 1
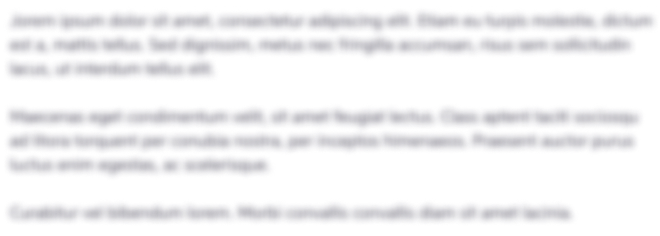
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started