Question
use the given outlines below for linked_queue.py and linked_queue.tests.py #linked_queue.py from __future__ import annotations from typing import Any, Optional class Node: def __init__(self, value: Any,
use the given outlines below for linked_queue.py and linked_queue.tests.py
#linked_queue.py
from __future__ import annotations
from typing import Any, Optional
class Node: def __init__(self, value: Any, nxt: Optional[Node]) -> None: self.value = value self.next = nxt
def __repr__(self) -> str: return "Node(%r, %r)" % (self.value, self.next)
def __eq__(self, other) -> bool: return ( isinstance(other, Node) and self.value == other.value and self.next == other.next )
class LinkedQueue: def __init__(self) -> None: self.head: Optional[Node] = None self.tail: Optional[Node] = None self.size = 0
def empty_queue() -> LinkedQueue: ...
def enqueue(queue: LinkedQueue, value: Any) -> None: ...
def dequeue(queue: LinkedQueue) -> Any: ...
def peek(queue: LinkedQueue) -> Any: ...
def is_empty(queue: LinkedQueue) -> bool: ...
def size(queue: LinkedQueue) -> int: ...
#linked_queue_tests.py
from __future__ import annotations
import unittest
from linked_queue import ( Node, dequeue, empty_queue, enqueue, is_empty, peek, size, )
class Tests(unittest.TestCase): def test_enqueue_one_value(self) -> None: my_queue = empty_queue() enqueue(my_queue, 10)
self.assertEqual(my_queue.head, Node(10, None)) self.assertEqual(my_queue.tail, Node(10, None)) self.assertEqual(my_queue.size, 1)
if __name__ == "__main__": unittest.main()
Details of each operation are given below; you will implement these operations for a link-based implementation and for a circular array implementation. You must verify, via test cases, that your implementations behave as expected (i.e., that they "work"). - empty_queue This function takes no arguments and returns an empty queue. - enqueue This function takes a queue and a value as arguments and adds the value to the "end" of the queue. Because both of our implementations will mutate the data structure, this function need not (and should not) return anything. - dequeue This function takes a queue as an argument and removes (and returns) the value at the "front" of the queue. If the queue is empty, then this operation should raise an IndexError. Again, because we will be mutating the data structure itself with the removal, we will only return the value being dequeued. - peek This function takes a queue as an argument and returns (without removing) the value at the "front" of the queue. If the queue is empty, then this operation should raise an IndexError. - is_empty This function takes a queue as an argument and returns whether or not the queue is empty. - size This function takes a queue as an argument and returns the number of items in the queue. For both implementations, these should all be O(1) (i.e., constant time) operations. 2 Linked Queue In a file named linked_queue.py, provide a data definition for a LinkedQueue. As discussed in class, there must be a way to add items to the back of the list and remove items from the front of the list. Implement the functions listed above. Be sure that all of them are O(1). Place your test cases in a file named 1 inked_queue_tests.pyStep by Step Solution
There are 3 Steps involved in it
Step: 1
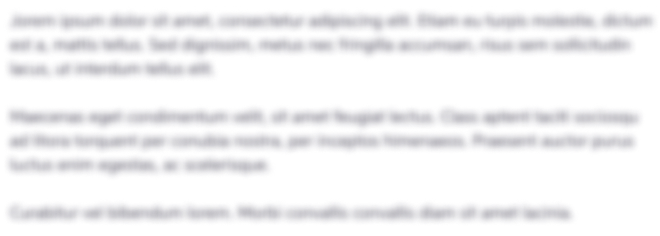
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started