Answered step by step
Verified Expert Solution
Question
1 Approved Answer
use the node adt IN PYTHON import node as N def sumnc (node_chain): - Purpose: Given a node chain with numeric data values, calculate the
use the node adt
IN PYTHON
import node as N def sumnc (node_chain): - Purpose: Given a node chain with numeric data values, calculate the sum of the data values. Pre-conditions: :param node_chain: a node-chain, possibly empty, containing numeric data values Post-condition: None Return :return: the sum of the data values in the node chain def sumnc (node chain): III Purpose: Given a node chain with numeric data values, calculate the sum of the data values. | Pre-conditions: :param node_chain: a node-chain, possibly empty, containing numeric data values Post-condition: None Return :return: the sum of the data values in the node chain return None def count_in(node_chain, value): Purpose: Counts the number of times a value appears in a node chain Pre-conditions: :param node_chain: a node chain, possibly empty :param value: a data value Return: :return: The number times the value appears in the node chain IF IT IT return None def replace_in(node chain target replacement): Purpose: Replaces each occurrence of the target value with the replacement Pre-conditions: :param node_chain: a node-chain, possibly empty :param target: a value that might appear in the node chain :param replacement: the value to replace the target Pre-conditions: Each occurrence of the target value in the chain is replaced with the replacement value. Return: None return None In this question you'll write three functions for node-chains. On Moodle, you can find a starter file called a5q4.py, with all the functions and doc-strings in place, and your job is to write the bodies of the functions. You will also find a test script named a5q4_testing.py. It has a bunch of test cases pre-written for you. Read it carefully! Also, use to_string() to help you test and debug your functions. (a) (6 points) Implement the function sumnc() (the nc suggests node chain). The interface for the function is: def sumnc (node_chain): Purpose: Given a node chain with numeric data values, calculate the sum of the data values. Pre-conditions: :param node_chain: a node-chain, possibly empty, containing numeric data values Post-condition: None Return :return: the sum of the data values in the node chain A demonstration of the application of the function is as follows: empty-chain = None chain = N.node (1, N.node (2, N.node (3))) print('empty chain has the sum', sumnc (empty-chain)) print('chain has the sum', sumnc (chain)) The output from the demonstration is as follows: empty chain has the sum o chain has the sum 6 (b) (6 points) Implement the function count_in(). The interface for the function is: def count_in(node_chain, value): Purpose: Counts the number of times a value appears in a node chain Pre-conditions: :param node_chain: a node chain, possibly empty :param value: a data value Return: :return: The number times the value appears in the node chain A demonstration of the application of the function is as follows: empty-chain - None chain = N.node(1, N.node (2, N.node (1))) print('empty chain has', count_in(empty-chain, 1), 'occurrences of the valpe 1') print('chain has', count_in(chain, 1), 'occurrences of the value 1') The output from the demonstration is as follows: empty chain has O occurrences of the value 1 chain has 2 occurrences of the value 1 (c) (6 points) Implement the function replace_in(). The interface for the function is: def replace_in(node_chain, target, replacement): Purpose: Replaces each occurrence of the target value with the replacement Pre-conditions: :param node_chain: a node-chain, possibly empty :param target: a value that might appear in the node chain :param replacement: the value to replace the target Pre-conditions: Each occurrence of the target value in the chain is replaced with the replacement value. Return: None A demonstration of the application of the function is as follows: chain1 = N.node (1 , N.node(1, N.node (9))) chain2 = N.node (2, N.node (7, N.node (15))) print('chaini before:', to_string(chaini)) replace_in(chaini, 1, 10) print('chain after:', to_string(chains)) print('chain2 before:' , to-string(chain2)) replace_in(chain2, 7, 1007) print('chain2 after:', to_string(chain2)) The output from the demonstration is as follows: chaini before: [1| + -]-->[ 1 | *-]-->[ 91/1 chaint after: [ 10 ] +-]-->[ 10 ] +-] -->[ 9/1 chain2 before: [ 2 | +-]-->[ 7 | *-]-->[ 15 | / ] chain2 after: [ 2 | *-]-->[ 1007 | *-]-->[ 15 /] Evaluation 6 marks: Your function sumnc(): - Uses the Node ADT to sum the data values in the chain correctly. - Works on node-chains of any length. - If your function uses for-loops. Python lists, or the Python sum() function, you will get ZERO marks. 6 marks: Your function count_in(): - Uses the Node ADT to count the data values in the chain correctly. - Works on node-chains of any length. - If your function uses for-loops, or Python lists, you will get ZERO marks. 6 marks: Your function replace_in(): - Uses the Node ADT to replace the data values in the chain correctly. - Works on node-chains of any length. - Does not create any new nodes. - If your function uses for-loops, or Python lists, you will get ZERO marks. import node as N def sumnc (node_chain): - Purpose: Given a node chain with numeric data values, calculate the sum of the data values. Pre-conditions: :param node_chain: a node-chain, possibly empty, containing numeric data values Post-condition: None Return :return: the sum of the data values in the node chain def sumnc (node chain): III Purpose: Given a node chain with numeric data values, calculate the sum of the data values. | Pre-conditions: :param node_chain: a node-chain, possibly empty, containing numeric data values Post-condition: None Return :return: the sum of the data values in the node chain return None def count_in(node_chain, value): Purpose: Counts the number of times a value appears in a node chain Pre-conditions: :param node_chain: a node chain, possibly empty :param value: a data value Return: :return: The number times the value appears in the node chain IF IT IT return None def replace_in(node chain target replacement): Purpose: Replaces each occurrence of the target value with the replacement Pre-conditions: :param node_chain: a node-chain, possibly empty :param target: a value that might appear in the node chain :param replacement: the value to replace the target Pre-conditions: Each occurrence of the target value in the chain is replaced with the replacement value. Return: None return None In this question you'll write three functions for node-chains. On Moodle, you can find a starter file called a5q4.py, with all the functions and doc-strings in place, and your job is to write the bodies of the functions. You will also find a test script named a5q4_testing.py. It has a bunch of test cases pre-written for you. Read it carefully! Also, use to_string() to help you test and debug your functions. (a) (6 points) Implement the function sumnc() (the nc suggests node chain). The interface for the function is: def sumnc (node_chain): Purpose: Given a node chain with numeric data values, calculate the sum of the data values. Pre-conditions: :param node_chain: a node-chain, possibly empty, containing numeric data values Post-condition: None Return :return: the sum of the data values in the node chain A demonstration of the application of the function is as follows: empty-chain = None chain = N.node (1, N.node (2, N.node (3))) print('empty chain has the sum', sumnc (empty-chain)) print('chain has the sum', sumnc (chain)) The output from the demonstration is as follows: empty chain has the sum o chain has the sum 6 (b) (6 points) Implement the function count_in(). The interface for the function is: def count_in(node_chain, value): Purpose: Counts the number of times a value appears in a node chain Pre-conditions: :param node_chain: a node chain, possibly empty :param value: a data value Return: :return: The number times the value appears in the node chain A demonstration of the application of the function is as follows: empty-chain - None chain = N.node(1, N.node (2, N.node (1))) print('empty chain has', count_in(empty-chain, 1), 'occurrences of the valpe 1') print('chain has', count_in(chain, 1), 'occurrences of the value 1') The output from the demonstration is as follows: empty chain has O occurrences of the value 1 chain has 2 occurrences of the value 1 (c) (6 points) Implement the function replace_in(). The interface for the function is: def replace_in(node_chain, target, replacement): Purpose: Replaces each occurrence of the target value with the replacement Pre-conditions: :param node_chain: a node-chain, possibly empty :param target: a value that might appear in the node chain :param replacement: the value to replace the target Pre-conditions: Each occurrence of the target value in the chain is replaced with the replacement value. Return: None A demonstration of the application of the function is as follows: chain1 = N.node (1 , N.node(1, N.node (9))) chain2 = N.node (2, N.node (7, N.node (15))) print('chaini before:', to_string(chaini)) replace_in(chaini, 1, 10) print('chain after:', to_string(chains)) print('chain2 before:' , to-string(chain2)) replace_in(chain2, 7, 1007) print('chain2 after:', to_string(chain2)) The output from the demonstration is as follows: chaini before: [1| + -]-->[ 1 | *-]-->[ 91/1 chaint after: [ 10 ] +-]-->[ 10 ] +-] -->[ 9/1 chain2 before: [ 2 | +-]-->[ 7 | *-]-->[ 15 | / ] chain2 after: [ 2 | *-]-->[ 1007 | *-]-->[ 15 /] Evaluation 6 marks: Your function sumnc(): - Uses the Node ADT to sum the data values in the chain correctly. - Works on node-chains of any length. - If your function uses for-loops. Python lists, or the Python sum() function, you will get ZERO marks. 6 marks: Your function count_in(): - Uses the Node ADT to count the data values in the chain correctly. - Works on node-chains of any length. - If your function uses for-loops, or Python lists, you will get ZERO marks. 6 marks: Your function replace_in(): - Uses the Node ADT to replace the data values in the chain correctly. - Works on node-chains of any length. - Does not create any new nodes. - If your function uses for-loops, or Python lists, you will get ZERO marksStep by Step Solution
There are 3 Steps involved in it
Step: 1
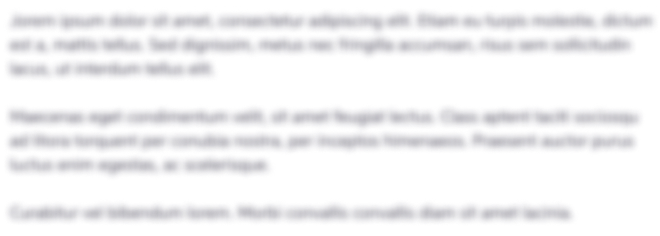
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started