Question
Use the Package inheritance hierarchy created in the code given below to create a program that displays the address information and calculates the shipping costs
Use the Package inheritance hierarchy created in the code given below to create a program that displays the address information and calculates the shipping costs for several Packages. The program should contain a vector of Package pointers to objects of classes TwoDayPackage and OvernightPackage . Loop through the vector to process the Package s polymorphically. For each Package , invoke get functions to obtain the address information of the sender and the recipient, then print the two addresses as they would appear on mailing labels. Also, call each Package s calculateCost member function and print the result. Keep track of the total shipping cost for all Packages in the vector , and display this total when the loop terminates.
Solution 11.9):- // Package.h #ifndef PACKAGE_H #define PACKAGE_H class Package { private: string senderName; string senderAddress; string recipientName; string recipientAddress; float weight; float costPerOunce; public: Package(string sender_n, string sender_addr, string recipient_n, string recipient_addr, float wei); void setsenderName(string sender_n); string getsenderName(); void setsenderAddress(string sender_addr); string getsenderAddress(); void setrecipientName(string recipient_n); string getrecipientName(); void setrecipientAddress(string recipient_addr); string getrecipientAddress(); void setweight(float w); float getweight(); void setcostperounce(float cost); float getcostperounce(); virtual float calculateCost(); }; #endif +=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // Package.cpp #include using namespace std; #include "Package.h" Package::Package(string sender_n, string sender_addr, string recipient_n, string recipient_addr, float wei) { senderName = sender_n; senderAddress = sender_addr; recipientName = recipient_n; recipientAddress = recipient_addr; costPerOunce = 0.5; if (wei > 0.0) { weight = wei; } else { weight = 0.0; } } void Package::setsenderName(string sender_n) { senderName = sender_n; } string Package::getsenderName() { return senderName; } void Package::setsenderAddress(string sender_addr) { senderAddress = sender_addr; } string Package::getsenderAddress() { return senderAddress; } void Package::setrecipientName(string recipient_n) { recipientName = recipient_n; } string Package::getrecipientName() { return recipientName; } void Package::setrecipientAddress(string recipient_addr) { recipientAddress = recipient_addr; } string Package::getrecipientAddress() { return recipientAddress; } void Package::setweight(float w) { weight = (w < 0.0) ? 0.0 : w; } float Package::getweight() { return weight; } void Package::setcostperounce(float cost) { costPerOunce = (cost < 0.0) ? 0.0 : cost; } float Package::getcostperounce() { return costPerOunce; } float Package::calculateCost() { float result; result = weight * costPerOunce; return result; } +=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // TwoDayPackage.h #ifndef TWODAYDELIVERY_H #define TWODAYDELIVERY_H #include "Package.h" class TwoDayPackage : public Package { private: float flatFee; public: TwoDayPackage(string sender_n, string sender_addr, string recipient_n, string recipient_addr, float wei); float getFlatFee(); void setFlatFee(double flatFee); float calculateCost(); }; #endif +=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // TwoDayPackage.cpp #include using namespace std; #include "TwoDayPackage.h" TwoDayPackage::TwoDayPackage(string sender_n, string sender_addr, string recipient_n, string recipient_addr, float wei) : Package(sender_n, sender_addr, recipient_n, recipient_addr, wei) { setFlatFee(2.0); } float TwoDayPackage::getFlatFee() { return flatFee; } void TwoDayPackage::setFlatFee(double flat_fee) { this->flatFee = flat_fee; } float TwoDayPackage::calculateCost() { double result; result = getFlatFee() * getweight() + (getweight() * getcostperounce()); return result; } +=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // OvernightPackage.h #ifndef OVERNIGHT_H #define OVERNIGHT_H #include "Package.h" class OvernightPackage : public Package { private: float additionalCostPerOunce; public: OvernightPackage(string sender_n, string sender_addr, string recipient_n, string recipient_addr, float wei); float calculateCost(); float getAdditionalCostPerOunce(); void setAdditionalCostPerOunce(float delivery_fee); }; #endif +=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // OvernightPackage.cpp #include using namespace std; #include "OverNightPackage.h" OvernightPackage::OvernightPackage(string sender_n, string sender_addr, string recipient_n, string recipient_addr, float wei) : Package(sender_n, sender_addr, recipient_n, recipient_addr, wei) { setAdditionalCostPerOunce(5.0); } float OvernightPackage::getAdditionalCostPerOunce() { return additionalCostPerOunce; } void OvernightPackage::setAdditionalCostPerOunce(float delivery_fee) { additionalCostPerOunce = delivery_fee; } float OvernightPackage::calculateCost() { float result; result = getAdditionalCostPerOunce() * getweight() + (getweight() * getcostperounce()); return result; } +=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // main.cpp #include #include using namespace std; #include "TwoDayPackage.h" #include "OvernightPackage.h" int main() { const int SIZE = 4; cout << fixed << setprecision(2); OvernightPackage item1("James", "RaceCourse Raod,Banglore", "Billy", "ChurchRoad,Mumbai", 5); TwoDayPackage item2("Smith", "WhiteField,Banglore", "Kane", "Andheri,Mumbai", 10); Package item3("Thomson", "BesentRoad,Vijayawada", "Adams", "WestSideRoad,Kolkatta", 4); TwoDayPackage item4("Mike", "Madiwala,Banglore", "Sachin", "RailStreet,Jaipur", 3); Package* items[4] = { &item1, &item2, &item3, &item4 }; cout << "*******************" << endl; cout << "Package delivery information list" << endl; cout << endl; for (int i = 0; i < SIZE; i++) { cout << " Package " << (i + 1) << ":" << endl; cout << "Sender :" << endl; cout << items[i]->getsenderName() << " "; cout << items[i]->getsenderAddress() << " "; cout << " "; cout << "Recipient :" << endl; cout << items[i]->getrecipientName() << " "; cout << items[i]->getsenderAddress() << " "; cout << " "; cout << "Cost: $" << items[i]->calculateCost() << endl; cout << "*************" << endl; } return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
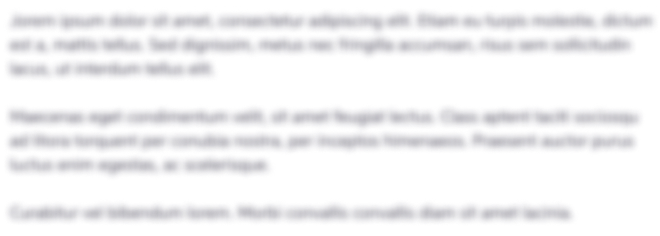
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started