Question
Use the Student and Name classes below. Student class: public class Student { private Name fullName; private String id; // Identification number public Student() {
Use the Student and Name classes below.
Student class:
public class Student
{
private Name fullName;
private String id; // Identification number
public Student()
{
fullName = new Name();
id = "";
} // end default constructor
public Student(Name studentName, String studentId)
{
fullName = studentName;
id = studentId;
} // end constructor
public void setStudent(Name studentName, String studentId)
{
setName(studentName); // Or fullName = studentName;
setId(studentId); // Or id = studentId;
} // end setStudent
public void setName(Name studentName)
{
fullName = studentName;
} // end setName
public Name getName()
{
return fullName;
} // end getName
public void setId(String studentId)
{
id = studentId;
} // end setId
public String getId()
{
return id;
} // end getId
public String toString()
{
return id + " " + fullName.toString();
} // end toString
} // end Student
} // end Student
Name Class:
public class Name
{
private String first; // First name
private String last; // Last name
public Name()
{
} // end default constructor
public Name(String firstName, String lastName)
{
first = firstName;
last = lastName;
} // end constructor
public void setName(String firstName, String lastName)
{
setFirst(firstName);
setLast(lastName);
} // end setName
public String getName()
{
return toString();
} // end getName
public void setFirst(String firstName)
{
first = firstName;
} // end setFirst
public String getFirst()
{
return first;
} // end getFirst
public void setLast(String lastName)
{
last = lastName;
} // end setLast
public String getLast()
{
return last;
} // end getLast
public void giveLastNameTo(Name aName)
{
aName.setLast(last);
} // end giveLastNameTo
public String toString()
{
return first + " " + last;
} // end toString
} // end Name
{
aName.setLast(last);
} // end giveLastNameTo
public String toString()
{
return first + " " + last;
} // end toString
} // end Name
Assume you have a ListInterface
Write statements from the client perspective to accomplish the following tasks.
Display the first name of each student on the list.
Switch the first and last students in the list.
Do not interchange the first and last names of any student- switch the first and last students on the list.
Notes:
Do not make any assumptions about the length of the list. This means your code should work with empty lists, one-element lists, or longer lists.
Do not use the toArray() method.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
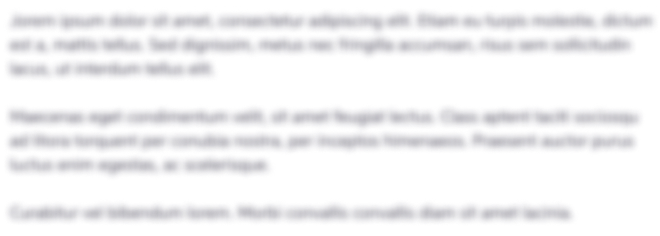
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started