Question
Use two files Money.h and Money.cpp which contain a Money class. You should download these files and add to them as specified below. //Money.h #include
Use two files Money.h and Money.cpp which contain a Money class. You should download these files and add to them as specified below.
//Money.h
#includeusing namespace std; class Money { private: int dollars; int cents; public: Money(); void setDollars(int d) { dollars = d; } void setCents(int c) { cents = c; } int getDollars() const { return dollars; } int getCents() const { return cents; } int dollarsToCents() const { return dollars * 100 + cents; } };
//Money.cpp
#include#include "Money.h" using namespace std; Money::Money() { dollars = 0; cents = 0; }
Add the following operator functions to the class. You will need to add the prototypes to the header file (Money.h) and the corresponding functions to the implementation file (Money.cpp).
operator== function returns true if two money objects are the same, false otherwise. Two money objects are the same if the dollars are the same and the cents are the same.
operator!= function returns true if two money objects are not the same, false otherwise. Two money objects are not the same if either the dollars or the cents are not the same.
operator<< should display a Money object in the form $5.06. To do this you need to display a $, dollars, . and cents.
Note if you have 5 dollars and 25 cents, the logic above will display $5.25, but if you have 6 dollars and 7 cents the logic above will display $6.7. So this function will require an if statement that displays a 0 if cents is < 10.
operator>> prompts the user to enter the dollars and cents and store it in the Money object
operator+ will add together the dollars and add together the cents of the two money objects
Example:
$5.25 + $6.30 = 11 dollars and 55 cents ($11.55) $5.25 + $6.90 = 11 dollars and 115 cents ($11.115), should be ($12.15)
This function will require an if statement, if cents becomes >= 100 you will need to add 1 to dollars (dollars++) and subtract 100 from cents (cents = cents 100)
Create a driver program lastname6.cpp that tests all the operators you added to the class. You can create as many Money objects as you need to test your class. Your driver program should contain documentation that shows what functions / operators you are testing. Use the final version of the Length class, as a guide on how to document your program.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
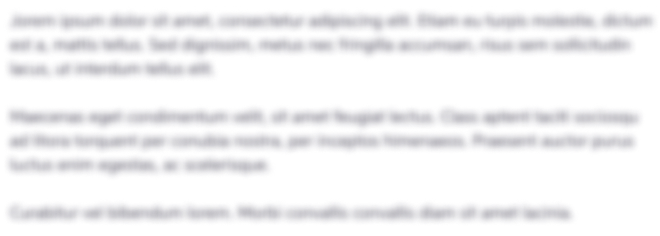
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started