Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Use Visual Studio // You are given a partially completed program that creates a list of books, say for library database. // Each book has
Use Visual Studio // You are given a partially completed program that creates a list of books, say for library database. // Each book has the this information: book name, publication year, library name, number of copies of that book. // The struct 'bookDetails' holds information of one book. Note that library name is enum type. // An array of structs called 'list' is made to hold a list of books. // To begin, you should trace through the given code and understand how it works. // Please read the instructions above each required function and follow the directions carefully. // If you modify any of the given code, the return types, or the parameters, you risk getting compile error // because you are not allowed to modify main (). // You can use string library functions. // WRITE COMMENTS FOR IMPORANT STEPS OF YOUR CODE. #include #include #include #include // needed to use tolower() #pragma warning(disable: 4996) // for Visual Studio Only #define MAX_BOOKS 20 #define MAX_NAME_LENGTH 35 typedef enum { noble = 0, hayden } library; // enumeration type library struct bookDetails { char bookName[MAX_NAME_LENGTH]; int pubYear; // publication year library libName; int noOfCopies; }; struct bookDetails list[MAX_BOOKS]; // declare list of books int count = 0; // the number of books currently stored in the list (initialized at 0) // forward declaration of functions (already implmented) void flushStdIn(); void executeAction(char); void save(char* fileName); // functions that need implementation: int add(char* bookName_input, int pubYear_input, char* libName_input, int noOfCopies_input); // 20 points void display(); // 10 points void sort(); // 10 points void load(char* fileName); // 10 points int main() { load("Book_List.txt"); // load list of books from file (if it exists). Initially there will be no file. char choice = 'i'; // initialized to a dummy value do { printf(" CSE240 HW5 "); printf("Please enter your selection: "); printf("\t a: add a new book to the list "); printf("\t d: display entire list of books "); printf("\t s: sort books by name "); printf("\t q: quit "); choice = tolower(getchar()); flushStdIn(); executeAction(choice); } while (choice != 'q'); save("Book_List.txt"); // save list of books to file (overwrites file, if it exists) return 0; } // flush out leftover ' ' characters void flushStdIn() { char c; do c = getchar(); while (c != ' ' && c != EOF); } // ask for details from user for the given selection and perform that action void executeAction(char c) { char bookName_input[MAX_NAME_LENGTH]; unsigned int pubYear_input, noOfCopies_input, add_result= 0; char libName_input[10]; switch (c) { case 'a': // input book details from user printf(" Please enter book name: "); fgets(bookName_input, sizeof(bookName_input), stdin); bookName_input[strlen(bookName_input) - 1] = '\0'; // discard the trailing ' ' char printf("Please enter publication year: "); scanf("%d", &pubYear_input); flushStdIn(); printf("Please enter library name (noble/hayden): "); fgets(libName_input, sizeof(libName_input), stdin); libName_input[strlen(libName_input) - 1] = '\0'; // discard the trailing ' ' char printf("Please enter no. of copies of book: "); scanf("%d", &noOfCopies_input); flushStdIn(); // add the book to the list add_result = add(bookName_input, pubYear_input, libName_input, noOfCopies_input); if (add_result == 0) printf(" That book is already on the list! "); else printf(" Book successfully added to the list! "); break; case 's': sort(); break; case 'd': display(); break; case 'q': break; default: printf("%c is invalid input! ",c); } } // Q1 : add (20 points) // This function is used to insert a new book into the list. You can simply insert the new book to the end of list (array of structs). // Do not allow the book to be added to the list if it already exists in the list. You can do that by checking the names of the books already in the list. // If the book already exists then return 0 without adding it to the list. If the book does not exist in the list then go on to add the book at the end of the list and return 1. // NOTE: You must convert the string 'libName_input' to an enum type and store it in the list because the struct has enum type for library name. // You can assume that user will input library name in lowercase. // Hint: 'count' holds the number of books currently in the list int add(char* bookName_input, int pubYear_input, char* libName_input, int noOfCopies_input) { return 0; // Comment out this line when implementing this function // It is added initially to avoid compile error } // Q2 : display (10 points) // This function displays the list of books with the book details (struct elements) of each book. // Parse through the book list and print the book details one after the other. See expected output screenshots in homework question file. // NOTE: Library name is stored in the struct as enum type. You need to display 'Noble' or 'Hayden' as library name for the book. void display() { } // Q3 : sort (10 points) // This function is used to sort the book list alphabetically by book name. // Parse the book list and compare the book names to check which one should appear before the other in the list. // Sorting should happen within the list. That is, you should not create a new list of books having sorted books. // Hint: One of the string library function can be useful to implement this function because the sorting needs to happen by book name which is a string. // Use a temp struct if you need to swap two book structs in your logic void sort() { struct bookDetails bookTemp; // needed for swapping book structs. Not absolutely necessary to use. // enter your code here // display message for user to check the result of sorting. printf(" Book list sorted! Use display option 'd' to view sorted list. "); } // save() is called at the end of main() // This function saves the array of structures to file. It is already implemented. // You should understand how this code works so that you know how to use it for future homework. // It will also help you with 'load' function. void save(char* fileName) { FILE* file; int i, libnameValue=0; file = fopen(fileName, "wb"); fwrite(&count, sizeof(count), 1, file); // First, store the number of books in the list // Parse the list and write book details to file1 // for (i = 0; iExpected output of each function add: C: \Users\kunal\source epos\Project6\Debug>Project6.exe ook List.txt not found. Initially there will be no saved file CSE240 HW5S Please enter your selection: a: add a new book to the list s: sort books by name d: display entire list of books q: quit a
Step by Step Solution
There are 3 Steps involved in it
Step: 1
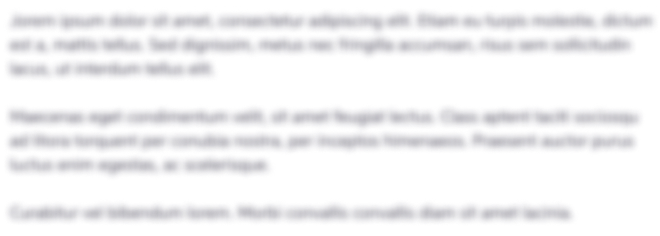
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started