Question
Using C++ in VS please In this assignment will demonstrate your understanding of the following: C++ classes; Implementing a class in C++; Operator overloading with
Using C++ in VS please
In this assignment will demonstrate your understanding of the following:
- C++ classes;
- Implementing a class in C++;
- Operator overloading with chaining;
- Preprocessor directives #ifndef, #define, and #endif;
- this the pointer to the current object.
In this assignment you will implement the Date class and test its functionality. Consider the following class declaration for the class date:
Consider the following class declaration for the class date:
class Date
{
public:
Date(); //default constructor; sets m=01, d=01, y=0001
Date(unsigned m, unsigned d, unsigned y); //explicit-value constructor to set date equal to
today's //date. Use 2-digits for month (m) and day (d), and 4-digits for year (y); this function
should //print a message if a leap year. void display();//output Date object to the screen int
getMonth();//accessor to output the month int getDay();//accessor to output the day int
getYear();//accessor to output the year void setMonth(unsigned m);//mutator to change the month
void setDay(unsigned d);//mutator to change the day void setYear(unsigned y);//mutation to change
this year
friend ostream & operator<<(ostream & out, Date & dateObj); //overloaded operator<< as a friend
//function with chaining
//you may add other functions if necessary
private: int myMonth, myDay, myYear; //month, day, and year of a Date obj respectively
};
You will implement all the constructors and member functions in the class Date. Please see the comments that follow each function prototype in the Date class declaration above; these comments describe the functionality that the function should provide to the class. The output of your program should match the given output shown, EXACTLY!
Notes:
Information on Month: 1 = January, 2 = February, 3= March, , 12 = December
- Test the functionality of your class in date_driver.cpp in the following order and include messages for each test:
- Test default constructor
- Test display
- Test getMonth
- Test getDay
- Test getYear
- Test setMonth
- Test setDay
- Test setYear
- See sample output below. YOUR PROGRAM OUTPUT SHOULD MATCH EXACTLY!
- See skeleton below.
S A M P L E O U T P U T FOR Date Assignment#2
Default constructor has been called
01\01\0001
Explicit-value constructor has been called
11\14\1953
Explicit-value constructor has been called
Month = 25 is incorrect
Explicit-value constructor has been called
02\29\2020
This is a leap year
Explicit-value constructor has been called
day = 30 is incorrect
Explicit-value constructor has been called
Year = 0000 is incorrect
Explicit-value constructor has been called
Month = 80 is incorrect day = 40 is incorrect Year = 0000 is incorrect
11\14\1953
11
14 1953
myDate: 11\12\2015 test22Date: 02\29\2020 herDate: 11\14\1953
Skeleton
#include
#include
#include
//#include "date.h"
using namespace std;
//********************************************************************************************* //*****
D A T E . h
//This declaration should go in date.h
#ifndef DATE_H
#define DATE_H
class Date
{
public:
Date(); //default constructor; sets m=01, d=01, y=0001
Date(unsigned m, unsigned d, unsigned y); //explicit-value constructor to set date equal to
today's //date. Use 2-digits for month (m) and day (d), and 4-digits for year (y); this function
should //print a message if a leap year. void display();//output Date object to the screen int
getMonth();//accessor to output the month int getDay();//accessor to output the day int
getYear();//accessor to output the year void setMonth(unsigned m);//mutator to change the month
void setDay(unsigned d);//mutator to change the day void setYear(unsigned y);//mutation to change
this year
friend ostream & operator<<(ostream & out, Date & dateObj); //overloaded operator<< as a friend
//function with chaining
//you may add other functions if necessary
private: int myMonth, myDay, myYear; //month, day, and year of a Date obj
respectively
};
#endif
//*****************
//*****************
//
D A T E . C P P
//This stub (for now) should be implemented in date.cpp
//*************************************************************************************
//Name: Date
//Precondition: The state of the object (private data) has not been initialized
//Postcondition: The state has been initialized to today's date
//Description: This is the default constructor which will be called automatically when //an object is declared. It will initialize the state of the class //
//*************************************************************************************
Date::Date()
{
}
//*************************************************************************************
//Name: Date
//Precondition:
//Postcondition:
//Description:
//
//
//*************************************************************************************
Date::Date(unsigned m, unsigned d, unsigned y)
{
}
//************************************************************************************
//Name: Display
//Precondition:
//Postcondition:
//Description:
//
//
//*************************************************************************************
void Date::display()
{
}
//*************************************************************************************
//Name: getMonth
//Precondition:
//Postcondition:
//Description:
//
//
//*************************************************************************************
int Date::getMonth()
{
return 1;
}
//*************************************************************************************
//Name: getDay
//Precondition:
//Postcondition:
//Description:
//
//
//*************************************************************************************
int Date::getDay()
{
return 1;
}
//*************************************************************************************
//Name: getYear
//Precondition:
//Postcondition:
//Description:
//
//
//*************************************************************************************
int Date::getYear()
{
return 1;
}
//*************************************************************************************
//Name: setMonth
//Precondition:
//Postcondition:
//Description:
//
//
//*************************************************************************************
void Date::setMonth(unsigned m)
{
}
//*************************************************************************************
//Name: setDay
//Precondition:
//Postcondition:
//Description:
//
//
//*************************************************************************************
void Date::setDay(unsigned d)
{
}
//*************************************************************************************
//Name: getYear
//Precondition:
//Postcondition:
//Description:
//
//
//*************************************************************************************
void Date::setYear(unsigned y)
{
}
ostream & operator<<(ostream & out, Date& dateObj)
{
return out;
}
//*********************************************************************************************
//********************************************************************************************* //
D A T E D R I V E R . C P P
//EXAMPLE OF PROGRAM HEADER
/********************************************************************************************
Name: Z#:
Course: Date: Due Time:
Total Points: Assignment 3: Date program
Description:
*******************************************************************************************/
int main()
{
Date myDate;
Date herDate(11,14, 1953);
Date test1Date(25, 1, 1982); //should generate error message that bad month
Date test22Date(2, 29, 2020); //ok, should say leep year
Date test3Date(2, 30, 2021); //should generate error message that bad day
Date test4Date(1,25,0000); //should generate error message that bad year
Date test5Date(80,40,0000); //should generate error message that bad month, day and year
herDate.display();
cout< cout< cout< myDate.setMonth(11); myDate.setDay(12); myDate.setYear(2015); cout<<"myDate: "< return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
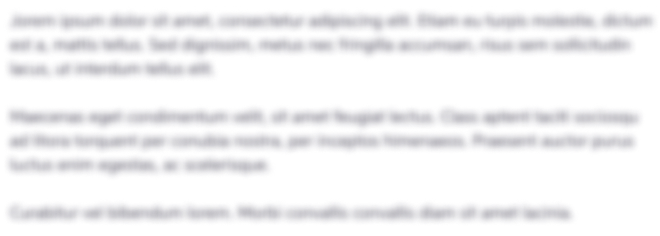
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started