Question
using C++ to implement the circular buffer according to the functions below : #ifndef H_circularBuffer #define H_circularBuffer #include #include using namespace std; //Definition of the
using C++ to implement the circular buffer according to the functions below :
#ifndef H_circularBuffer
#define H_circularBuffer
#include
#include
using namespace std;
//Definition of the node
template
struct nodeType
{
Type info;
nodeType
};
template
class circularBuffer
{
protected:
int count; //determines number of items that can be processed
int size; //number of nodes to hold items to process
nodeType
nodeType
public:
circularBuffer();
circularBuffer(int size);
//default constructor
//Initializes the buffer to an empty state with size empty slots
//Postcondition: size nodes allocated, count = 0, nextToWrite
// and nextToRead both point to first node to write to.
~circularBuffer();
//destructor
//Postcondition: The buffer object is destroyed. All nodes deallocated
void Dequeue();
bool isEmpty() const;
//Function to determine whether the buffer is empty.
//Postcondition: Returns true if the buffer is empty,
// otherwise returns false.
bool isFull() const;
//Function to determine whether the buffer is full.
//Postcondition: Returns true if the buffer is full,
// otherwise returns false.
void clear();
//Function to 'delete' all nodes from the buffer. In reality this means
// all nodes are available for writing i.e. buffer is re-initialized
//Postcondition: nextToRead = nextToWrite and count = 0;
void displayBuffer () const;
//Function to output the info contained in each node.
int length() const;
//Function to return the number of records in the buffer.
//Postcondition: The value of count is returned.
int capacity() const;
//Function to return the number of slots in the buffer.
//Postcondition: The value of size is returned.
Type& read();
//Function to read the next record for processing.
//Precondition: The buffer must be nonempty, or the function
// throws an exception
//Postcondition: count is decremented by 1, the value pointed at by nextToRead
// is return`ed, and nextToRead is moved to the next node in the buffer,
// and count is decremented by 1.
void write(circularBuffer *q, const Type & newItem);
//Function to write new record for processing.
//Precondition: If the buffer is nonempty, count must be
// between zero and (size-1), otherwise the write
// throws an approate exception.
//Postcondition: newtItem is placed into the node pointed at by nextToWrite.
// nextToWrite is moved to point to the next node in the buffer,
// and count is incremented by 1.
};
// Implement the provided methods here
Step by Step Solution
There are 3 Steps involved in it
Step: 1
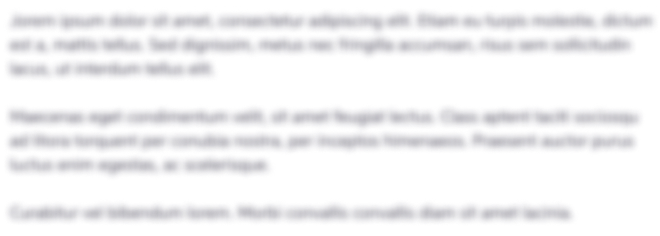
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started