Question
USING CCS PROGRAMMING PLEASE MODIFY THE CODE BELOW TO DO THE FOLLOWING: -Click button S1 to double the LED blinking frequency. -Click button S2 to
USING CCS PROGRAMMING PLEASE MODIFY THE CODE BELOW TO DO THE FOLLOWING:
-Click button S1 to double the LED blinking frequency.
-Click button S2 to decrease the LED blinking frequency by half.
-Define a minimum 2-4 Hz and a maximum 24 Hz blinking frequencies.
#include
#include
#include
#include
#define DCO_FREQ 48e6 //unit: Hz; DCO nominal frequencies: 1.5, 3, 6, 12, 24,
48 MHz.
#define TIMER0_FREQ 1 //unit: Hz
#define SYSTICK_FREQ 200 //unit: Hz
#define RED_LED GPIO_PIN0
#define GREEN_LED GPIO_PIN1
#define BLUE_LED GPIO_PIN2
#define BUTTON_S1 GPIO_PIN1
#define NUM_DEBOUNCE_CHECKS 10 //For 50 msec debounce time.
#define NUM_DISP_TEXT_LINE 4
//function prototypes
void initDevice(void);
void initGPIO(void);
void initTimer(void);
void initUART(void);
void uart0_transmitStr(const char *str);
// global variables
uint32_t clockMCLK;
uint8_t currentLED = RED_LED;
volatile uint32_t buttonStateIndex;
volatile uint32_t buttonS1_pressed = 0; //0: unpressed, 1: pressed
const char *terminalDisplayText[NUM_DISP_TEXT_LINE] =
{
" ",
"UART and User Button Demo ",
"R: red, G: green, B:blue, H: Help ",
"> "
};
void main(void)
{
uint32_t i;
uint8_t data;
initDevice();
initGPIO();
initTimer();
initUART();
Interrupt_enableMaster();
Timer32_startTimer(TIMER32_0_BASE, false);
// Initial display on terminal.
for(i=0; i { uart0_transmitStr(terminalDisplayText[i]); } while(1) { // Handle UART communicataion from host PC to MSP432. if(UART_getInterruptStatus(EUSCI_A0_BASE, EUSCI_A_UART_RECEIVE_INTERRUPT_FLAG)) { data = UART_receiveData(EUSCI_A0_BASE); UART_clearInterruptFlag(EUSCI_A0_BASE, EUSCI_A_UART_RECEIVE_INTERRUPT_FLAG); switch(data) { case 'R': case 'r': currentLED = RED_LED; uart0_transmitStr("Blink red LED. > "); break; case 'G': case 'g': currentLED = GREEN_LED; uart0_transmitStr("Blink green LED. > "); break; case 'B': case 'b': currentLED = BLUE_LED; uart0_transmitStr("Blink blue LED. > "); break; case 'H': case 'h': for(i=0; i { uart0_transmitStr(terminalDisplayText[i]); } break; } } //end of if if(buttonS1_pressed) { buttonS1_pressed = 0; uart0_transmitStr("Button S1 is pressed. > "); // And you can do other things here in response to button S1 being pressed. } } //end of while } void initDevice(void) { WDT_A_holdTimer(); //stop Watchdog timer //Change VCORE to 1 to support a frequency higher than 24MHz. //See data sheet for Flash wait-state requirement for a given frequency. PCM_setPowerState(PCM_AM_LDO_VCORE1); FlashCtl_setWaitState(FLASH_BANK0, 1); FlashCtl_setWaitState(FLASH_BANK1, 1); //Enable FPU for DCO Frequency calculation. FPU_enableModule(); //Only use DCO nominal frequencies: 1.5, 3, 6, 12, 24, 48MHz. CS_setDCOFrequency(DCO_FREQ); //Divider: 1, 2, 4, 8, 16, 32, 64, or 128. //SMCLK is used by UART. CS_initClockSignal(CS_MCLK, CS_DCOCLK_SELECT, CS_CLOCK_DIVIDER_1); CS_initClockSignal(CS_HSMCLK, CS_DCOCLK_SELECT, CS_CLOCK_DIVIDER_8); CS_initClockSignal(CS_SMCLK, CS_DCOCLK_SELECT, CS_CLOCK_DIVIDER_16); clockMCLK = CS_getMCLK(); } void initGPIO(void) { //Configure P2.0, P2.1, P2.2 as output. //P2.0, P2.1, P2.2 are connected to a RGB tri-color LED on LaunchPad. GPIO_setAsOutputPin(GPIO_PORT_P2, GPIO_PIN0|GPIO_PIN1|GPIO_PIN2); //Configure P1.1 (button S1) as an input and enable interrupts. GPIO_setAsInputPinWithPullUpResistor(GPIO_PORT_P1, GPIO_PIN1); GPIO_clearInterruptFlag(GPIO_PORT_P1, GPIO_PIN1); GPIO_interruptEdgeSelect(GPIO_PORT_P1, GPIO_PIN1, GPIO_HIGH_TO_LOW_TRANSITION); GPIO_enableInterrupt(GPIO_PORT_P1, GPIO_PIN1); Interrupt_enableInterrupt(INT_PORT1); } void initTimer(void) { Timer32_initModule(TIMER32_0_BASE, TIMER32_PRESCALER_1, TIMER32_32BIT, TIMER32_PERIODIC_MODE); Timer32_setCount(TIMER32_0_BASE, clockMCLK/TIMER0_FREQ); Timer32_enableInterrupt(TIMER32_0_BASE); Interrupt_enableInterrupt(INT_T32_INT1); // Enable Timer32_0 interrupt in the interrupt controller. //SysTick is clocked with the CPU free running clock, MCLK. //The set period must be between 1 and 16,777,215. SysTick_setPeriod(clockMCLK/SYSTICK_FREQ); } void initUART(void) { //Configuration for 3MHz SMCLK, 9600 baud rate. //Calculated using the online calculator that TI provides at: //http://software-dl.ti.com/msp430/msp430_public_sw/mcu/msp430/MSP430BaudRateConver ter/index.html const eUSCI_UART_Config config = { EUSCI_A_UART_CLOCKSOURCE_SMCLK, //SMCLK Clock Source 19, //BRDIV = 19 8, //UCxBRF = 8 0, //UCxBRS = 0 EUSCI_A_UART_NO_PARITY, //No Parity EUSCI_A_UART_LSB_FIRST, //MSB First EUSCI_A_UART_ONE_STOP_BIT, //One stop bit EUSCI_A_UART_MODE, //UART mode EUSCI_A_UART_OVERSAMPLING_BAUDRATE_GENERATION //Oversampling }; //Configure GPIO pins for UART. RX: P1.2, TX:P1.3. GPIO_setAsPeripheralModuleFunctionInputPin(GPIO_PORT_P1, GPIO_PIN2|GPIO_PIN3, GPIO_PRIMARY_MODULE_FUNCTION); UART_initModule(EUSCI_A0_BASE, &config); UART_enableModule(EUSCI_A0_BASE); } //Transmit a string through UART0. void uart0_transmitStr(const char *str) { uint32_t len, i=0; len = strlen(str); while(i < len) { UART_transmitData(EUSCI_A0_BASE, str[i++]); while(!UART_getInterruptStatus(EUSCI_A0_BASE, EUSCI_A_UART_TRANSMIT_COMPLETE_INTERRUPT_FLAG)); UART_clearInterruptFlag(EUSCI_A0_BASE, EUSCI_A_UART_TRANSMIT_COMPLETE_INTERRUPT_FLAG); } } //Timer32_0 ISR void T32_INT1_IRQHandler(void) { Timer32_clearInterruptFlag(TIMER32_0_BASE); if(GPIO_getInputPinValue(GPIO_PORT_P2, GPIO_PIN0|GPIO_PIN1|GPIO_PIN2)) { GPIO_setOutputLowOnPin(GPIO_PORT_P2, GPIO_PIN0|GPIO_PIN1|GPIO_PIN2); } else { GPIO_setOutputHighOnPin(GPIO_PORT_P2, currentLED); } } //Port P1 ISR void PORT1_IRQHandler(void) { uint8_t status; status = GPIO_getEnabledInterruptStatus(GPIO_PORT_P1); GPIO_clearInterruptFlag(GPIO_PORT_P1, status); if(status & BUTTON_S1) { //Start SysTick for debouncing button uart0_transmitStr("Button S1 interrupt triggered. > "); buttonStateIndex = 0; SysTick->VAL = 0; //To cause immediate reload to SysTick counter. SysTick_enableModule(); //Enable and start the SysTick counter. SysTick_enableInterrupt(); } } //SysTick ISR void SysTick_Handler(void) { uint8_t buttonState; //P1.1 is configured with a pull-up resistor, so P1.1 = 0 when pressed. //buttonState = 1 when pressed, which is opposite to P1.1 value. buttonState = GPIO_getInputPinValue(GPIO_PORT_P1, BUTTON_S1); buttonState = BUTTON_S1 & (~buttonState); buttonStateIndex++; if((buttonState & BUTTON_S1) == 0) { SysTick_disableInterrupt(); SysTick_disableModule(); } else if(buttonStateIndex >= NUM_DEBOUNCE_CHECKS) { //Button S1 is pressed SysTick_disableInterrupt(); SysTick_disableModule(); buttonS1_pressed = 1; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
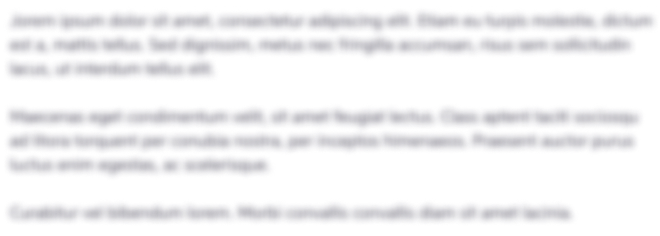
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started