Question
Using code from repl.it create a more robust MyVector class that will include more functionality. You will achieve this by writing additional methods to add
Using code from repl.it create a more robust MyVector
class that will include more functionality. You will achieve this by writing additional methods to add values, remove values, and maintain sorted values if needed. This class also needs the ability to be initialized with different data sources (files, other vectors, and arrays).C++
Right now the example code is a mish-mash of disconnected code snippets collected as we review last semester. There is a basic linked list class that has half the functionality of a stack. There are methods to print files, and load files into arrays. The class even has an overloaded constructor that loads an array into the linked list. But we need to clean up this code and turn it into a usable class that you or anyone would want to use. To do this we need to define exactly what we want our class to do. I will list out the requirements for you to fulfill below. Right now we will assume that we store integers, but we will rectify this in the upcoming programs when we overload operators and add type templates to our class.
Requirements
- Your class will be a basic container type of integers that can grow or shrink easily without the need to copy values over to newly allocated memory when resizing (I will explain this more in class).
- Initializing your class instance:
- From an array
V1 = new MyVector(int *A, int size)
: adds each item from an array type
- From a file
V1 = new MyVector(string FileName)
: read until eof loading each value into list
- From another instance of same type (this is known as a Copy Constructor and we discuss soon)
V2 = new MyVector(MyVector V1)
: traverseother.list
adding each value tothis.list
- From an array
- Adding items to your class instance:
- To the front
V.pushFront(int val)
: adds single value to front ofthis.list
V.pushFront(MyVector V2)
: adds entireother.list
to front ofthis.list
- To the rear
V.pushRear(int val)
: adds single value to rear ofthis.list
V.pushRear(MyVector V2)
: adds entireother.list
to rear ofthis.list
- At a specified location (if it exists)
V.pushAt(int loc,int val)
- In ascending or descending order (order set by constructor or setter)
V.inOrderPush(int val)
: adds single value to proper location in order to maintain order (ascending or descending)- There are some problems with this method. Ask me in class.
- To the front
- Removing items from a class instance:
- From the front
val = V.popFront()
: removes single value from front of list
- From the rear
val = V.popRear()
: removes single value from rear of list
- A specified location
val = V.popAt(int loc)
: removes single value from an indexed location if index between0 and size of list -1
- By a search than removal (if it exists)
loc = V.find(int val)
: find location of item (index) if it existsval = V.popAt(int loc)
) : then use index to remove item
- From the front
- Printing
- Output should look similar to: [v1, v2, v3, ... , vn]
Tests
Run this code with your class and make sure you get same output. The example below is writing to stdout only. I want your test program to write to stdout AND a file called test.out
. Your name, date and course (Fall 2143) should be written at top of output file (with your code):
Example Output File (portion of)
Name Date Fall 2143 [25, 20, 18, 18, 20, 25] [11, 25, 33, 47, 51] [9, 11, 25, 27, 33, 47, 51, 63] [25, 20, 18, 18, 20, 25, 9, 11, 25, 27, 33, 47, 51, 63] etc...
Example Code Run
int x = 0; MyVector v1; v1.pushFront(18); v1.pushFront(20); v1.pushFront(25); v1.pushRear(18); v1.pushRear(20); v1.pushRear(25); v1.print();// [25, 20, 18, 18, 20, 25]int A[] = {11,25,33,47,51}; MyVector v2(A,5); v2.print();// [11, 25, 33, 47, 51] v2.pushFront(9); v2.inOrderPush(27); v2.pushRear(63); v2.print();// [9, 11, 25, 27, 33, 47, 51, 63] v1.pushRear(v2); v1.print();// [25, 20, 18, 18, 20, 25, 9, 11, 25, 27, 33, 47, 51, 63] x = v1.popFront(); x = v1.popFront(); x = v1.popFront(); v1.print();// [18, 20, 25, 9, 11, 25, 27, 33, 47, 51, 63]cout<
Step by Step Solution
3.45 Rating (152 Votes )
There are 3 Steps involved in it
Step: 1
Code include include using namespace std class MyVector public vector vec constructo...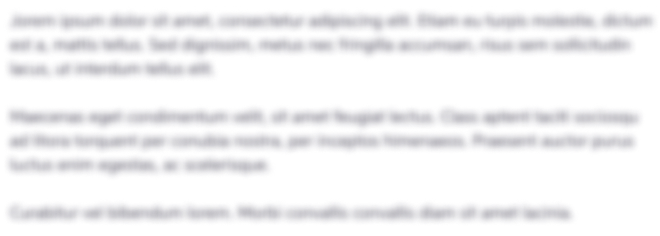
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started