Question
Using Java implement the Crazy Eights card game. Here are therules of our version of the game: Each player is initially dealt n cards
Using Java implement the Crazy Eights card game. Here are therules of our version of the game:
• Each player is initially dealt n cards from a deck of 52cards, where n is specified by the user.
• The winner of the game is the first person to discard (play)all the cards in their hand.
• To begin play after the cards are dealt, one card is drawnfrom the deck and placed on top of a discard pile (which is emptywhen the game starts).
• At their turn, each player must play a card that matches thetop card on the pile. The matching is determined as follows:
O cards of the same suit match
O cards of the same rank match
O A card with rank 8 can be used to change the suit of thecurrent card on top of the pile. This current top card is nowdeemed to have a rank of 8 and the new suit that was justdeclared.
• If a player is unable to play at their turn, they must drawthe top card from the deck and lose their turn.
• If no more cards are available in the deck, then the top cardon the discard pile is retained, and all other cards in the discardpile are added to the deck, which is then shuffled. And the gamethen resumes, from where it stopped.
• The game ends when there is a winner, or when no player canplay a card, and there
are no more cards in the deck.
Your task is to code:
• a game that enforces these rules
• a human player that can select cards and play the game
• a basic computer player that just plays the first card itcan
Do this by coding the following classes:
• CrazyEightPlayer that extends CardPlayer
• CrazyEightComputerPlayer that extendsCrazyEightPlayer
• CrazyEightHumanPlayer that extendsCrazyEightPlayer
• CrazyEightGame that extends CardGame
Finally, test this on a driver defined in a Driver.java filethat plays a crazy eight game between a human player and a computerplayer, each with 5 cards per hand initially.
HERE IS WHAT I HAVE CODED:
/**
* This class provides definitions for all the abstract methodsin
* its ancestors. The class simulates the computer playing,
* and simply randomly chooses the next card to play.
*
*/
import java.util.*;
public class HigherCardComputerPlayer extendsHigherCardPlayer
{
public HigherCardComputerPlayer(String name, int rounds){
super(name,rounds);
}
@Override
public void updateRoundsWon(){
rounds++;
}
@Override
public int getRoundsWon(){
return rounds;
}
@Override
public void dealCard(Card card){
hand.addCard(card);
}
@Override
public Card takeTurn(){
ArrayList cards = hand.getCards();
Random rng = new Random();
int index = rng.nextInt(cards.size());
return cards.remove(index);
}
}
/**
* This class updates how many rounds this player has won.
* It also retrieves how many rounds this player has won.
*
*/
package cardgame;
public abstract class HigherCardPlayer extends CardPlayer
{
protected int rounds;
protected Hand hand = new Hand(5);
public HigherCardPlayer(String name, int rounds){
super(name);
this.rounds = rounds;
}
public abstract void updateRoundsWon();
public abstract int getRoundsWon();
}
/**
* this class has a method called takeTurn that returns the card tobe played,
* from the hand held by this player. It also has a method calleddealCard that
* adds a card to the hand held by this player.
*
*/
package cardgame;
public abstract class CardPlayer {
private String name;
public CardPlayer(String name) //player name
{
this.name = name;
}
public abstract Card takeTurn();
public abstract void dealCard(Card card);
public String toString()
{
return name;
}
}
import java.util.*;
public abstract class CrazyEightPlayer extends CardPlayer
{
protected int rounds;
protected Hand hand = new Hand(5);
public CrazyEightPlayer(String name, int rounds){
super(name);
this.rounds = rounds;
}
public abstract void updateRoundsWon();
public abstract int getRoundsWon();
}
import java.util.*;
public class CrazyEightGame extends CardGame
{
protected Deck deck = new Deck();
private int currentRounds=0;
private int maxRounds;
ArrayList drawPile = new ArrayList();
ArrayList discardPile = new ArrayList();
Scanner in = new Scanner(System.in);
public CrazyEightGame(CrazyEightPlayer [] players, introunds){
super(players);
System.out.println("how many cards in each players hand");
int num = in.nextInt();
for(CrazyEightPlayer p : players){
for(int i = 0; i < num - 1; i++){
p.dealCard(deck.deal());
}
}
this.maxRounds = rounds;
}
public boolean isGameOver(){
return currentRounds > maxRounds;
}
public String getWinner(){
int maxScore = 0;
String winner = "";
for(CardPlayer c : players){
CrazyEightPlayer hc = (CrazyEightPlayer) c;
if(hc.getRoundsWon() > maxScore){
winner = hc.toString();
maxScore = hc.getRoundsWon();
}
}
return winner;
}
public void play(){
while(!isGameOver()){
Card highest = null;
CrazyEightPlayer winning = null;
for(CardPlayer c : players){
CrazyEightPlayer hc = (CrazyEightPlayer) c;
hc.dealCard(deck.deal());
Card playerCard = hc.takeTurn();
System.out.println(c.toString() + " played " +playerCard.toString());
if(highest==null){
highest = playerCard;
}
if(playerCard.compareTo(highest) >= 0){
highest = playerCard;
winning = hc;
}
}
winning.updateRoundsWon();
System.out.println(winning.toString() + " won!");
currentRounds ++;
}
}
}
/**
* this class loops through all players while game is notover,
* having each player take a turn until game is over, thenuses
* getWinner to display winner. It also checks to see if the game isover.
*
*/
package cardgame;
public abstract class CardGame {
protected CardPlayer[] players;
public CardGame(CardPlayer [] players)
{
this.players = players;
}
public abstract void play();
/* loops through all players while game is not over,
* having each player take a turn until game is over, thenuses
* getWinner to display winner */
public abstract boolean isGameOver();
/* check if game is over */
public abstract String getWinner();
/* returns the winner(s) */
}
/**
* this class provides implementations for all the abstract methodsin CardGame,
* that play the Higher card game as described. In theconstructor,
* it also creates the deck and deals out the cards to theplayers.
*
*/
public class HigherCardGame extends CardGame
{
protected Deck deck = new Deck();
private int currentRounds=0;
private int maxRounds;
public HigherCardGame(HigherCardPlayer [] players, introunds){
super(players);
for(HigherCardPlayer p : players){
for(int i = 0; i < 5; i++){
p.dealCard(deck.deal());
}
}
this.maxRounds = rounds;
}
public boolean isGameOver(){
return currentRounds > maxRounds;
}
public String getWinner(){
int maxScore = 0;
String winner = "";
for(CardPlayer c : players){
HigherCardPlayer hc = (HigherCardPlayer) c;
if(hc.getRoundsWon() > maxScore){
winner = hc.toString();
maxScore = hc.getRoundsWon();
}
}
return winner;
}
public void play(){
while(!isGameOver()){
Card highest = null;
HigherCardPlayer winning = null;
for(CardPlayer c : players){
HigherCardPlayer hc = (HigherCardPlayer) c;
hc.dealCard(deck.deal());
Card playerCard = hc.takeTurn();
System.out.println(c.toString() + " played " +playerCard.toString());
if(highest==null){
highest = playerCard;
}
if(playerCard.compareTo(highest) >= 0){
highest = playerCard;
winning = hc;
}
}
winning.updateRoundsWon();
System.out.println(winning.toString() + " won!");
currentRounds ++;
}
}
}
import java.util.*;
public class CrazyEightComputerPlayer extendsCrazyEightPlayer
{
public CrazyEightComputerPlayer(String name, int rounds){
super(name,rounds);
}
@Override
public void updateRoundsWon(){
rounds++;
}
@Override
public int getRoundsWon(){
return rounds;
}
@Override
public void dealCard(Card card){
hand.addCard(card);
}
@Override
public Card takeTurn(){
ArrayList cards = hand.getCards();
Random rng = new Random();
int index = rng.nextInt(cards.size());
return cards.remove(index);
}
}
import java.util.*;
public class CrazyEightHumanPlayer extendsCrazyEightPlayer
{
public CrazyEightHumanPlayer(String name, int rounds){
super(name,rounds);
}
@Override
public void updateRoundsWon(){
rounds++;
}
@Override
public int getRoundsWon(){
return rounds;
}
@Override
public void dealCard(Card card){
hand.addCard(card);
}
@Override
public Card takeTurn(){
Scanner in = new Scanner(System.in);
ArrayList cards = hand.getCards();
System.out.println(cards);
System.out.println("what element in the array list");
int element = in.nextInt();
return cards.get(element);
}
}
package cardgame;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Iterator;
public class Deck {
private ArrayList deck;
public Deck() {
this.deck = new ArrayList();
//adding Cards to deck
for (Rank card : Rank.values()) {
for (Suit suit : Suit.values()) {
deck.add(new Card(card.getCardValue(),suit));
}
}
shuffle();
}
//Returning first card index 0
public Card deal() {
return deck.remove(0);
}
// TOString implementation
@Override
public String toString() {
Iterator i = deck.iterator();
if (!i.hasNext())
return "...";
StringBuilder sb = new StringBuilder();
for (;;) {
Card e = i.next();
sb.append(e.toString());
if (!i.hasNext())
return sb.toString();
sb.append("");
}
}
// returns true if deck is empty
public boolean isDeckEmpty() {
return deck.isEmpty();
}
// shuffles cards in deck
public void shuffle() {
Collections.shuffle(deck);
}
//Sorting cards in deck
public void sort() {
Collections.sort(deck);
}
}
package cardgame;
public class DeckOrHandEmptyException extends CardException {
public DeckOrHandEmptyException(String message) {
super(message);
}
}
package cardgame;
public enum Rank
{
TWO(2),
THREE(3),
FOUR(4),
FIVE(5),
SIX(6),
SEVEN(7),
EIGHT(8),
NINE(9),
TEN(10),
JACK(11),
QUEEN(12),
KING(13),
ACE(14);
private int cardRank;
private Rank (int value)
{
this.cardRank = value;
}
public int getCardValue() {
return cardRank;
}
//Returns ENUM value for respective integer
public static Rank getRank(int rankint) {
for (Rank l : Rank.values()) {
if (l.cardRank == rankint) return l;
}
throw new IllegalArgumentException("error");
}
}
package cardgame;
public enum Suit
{
//Defined in order of rank
CLUBS,
DIAMONDS,
HEARTS,
SPADES
}
package cardgame;
import java.util.*;
public class Hand
{
private ArrayList cards;
public Hand(int m)
{
cards = new ArrayList(m);
}
public Card drawNext()
{
if(cards.isEmpty())
throw new DeckOrHandEmptyException("Hand is empty");
return cards.remove(0);
}
public Card draw(String s)
{
if(cards.isEmpty())
throw new DeckOrHandEmptyException("Hand is empty");
for(Card c : cards)
{
if(c.toString().equals(s))
{
cards.remove(c);
return c;
}
}
return null;
}
public void addCard(Card c) //adds card to hand
{
cards.add(c);
}
public ArrayList getCards() // gets the list of Cards inhand
{
return cards;
}
}
public class Card implements Comparable {
private Suit suit;
private int rank;
//constructor
public Card(int rank, Suit suit) {
this.rank = rank;
this.suit = suit;
}
// To String method
public String toString() {
return Rank.getRank(rank) + " of " + suit.name();
}
// Overriding compareTo method
@Override
public int compareTo(Card o) {
if (this.rank < o.rank) {
return -1;
} else if (this.rank > o.rank) {
return 1;
} else {
if (this.suit == o.suit) {
return 0;
} else
return this.suit.compareTo(o.suit);
}
}
// Getters
public int getRank() {
return rank;
}
public Suit getSuit() {
return suit;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
It seems that you have provided code snippets for several classes involved in a card game but they are not complete or consistent with the requirement...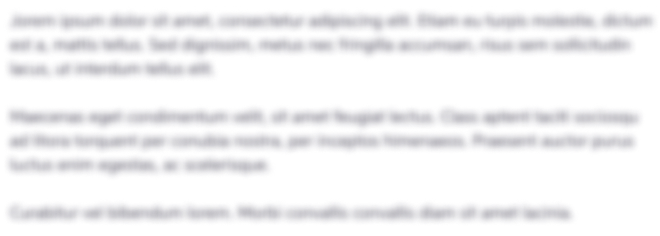
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started