Question
USING JAVA: - Open A7EmployeeChecker.java file and review the code. This is the file that creates some base employees and does some quick checks of
USING JAVA:
- Open A7EmployeeChecker.java file and review the code. This is the file that creates some base employees and does some quick checks of the methods on Employee. This will tell you if you coded the Employee file correct.
A7EmployeeChecker.java Below:
public class A7EmployeeChecker { public static void main(String[] args) { // setup employees Employee e1 = new Employee("Steve", "Rodgers", 3781, "Sales", "Manager", 28.50); Employee e2 = new Employee("Clint", "Barton", 6847, "Sales", "Customer Representative", 15.34); Employee e3 = new Employee("Tony", "Stark", 5749, "Service", "Lead Service Manager", 32.85); System.out.println("***** Assignment 7 Employee Checker ***** "); // print employees e1.printEmployee(); System.out.println(); e2.printEmployee(); System.out.println(); e3.printEmployee(); System.out.println(); System.out.println(); // add some hours e1.addHours(5); e3.addHours(); e2.addHours(12); e1.addHours(-20); // make sure you have negative check // print pay System.out.println("Employee Pay:"); System.out.printf("%s : $%.2f%n", e1.getFirstName(), (double)Math.round(e1.calculateWeeklyPay()*100)/100); System.out.printf("%s : $%.2f%n", e2.getFirstName(), (double)Math.round(e2.calculateWeeklyPay()*100)/100); System.out.printf("%s : $%.2f%n%n", e3.getFirstName(), (double)Math.round(e3.calculateWeeklyPay()*100)/100); // copy employee and modify Employee e4 = new Employee(e3); e4.setFirstName("Thor"); e4.setLastName("Odinson"); e4.setEmployeeNumber(8623); e4.setPayRate(24.36); e4.addHours(18); // print new employee info System.out.println(" New Employee:"); e4.printEmployee(); System.out.println(); // reset hours e1.resetHours(); e2.resetHours(); e3.resetHours(); e4.resetHours(); System.out.println(" Last employee print: "); // last print of employees e1.printEmployee(); System.out.println(); e2.printEmployee(); System.out.println(); e3.printEmployee(); System.out.println(); e4.printEmployee(); } } // ********************** DO NOT MODIFY ***************************
- Create a new class called Employee.java. This will be our class that holds the information about each employee. Review the UML diagram below as it will help you with the layout for the file. I have also given notes for specifics on what some of the methods require.
NOTES ON METHODS/VARIABLES TO FOLLOW:
- Static Variable:
See that currency is a NumberFormat static variable and should be setup with the getCurrencyInstance() at the variable declaration NOT in the constructors
-Constructors
Each constructor should setup hoursWorked to 0.0
The second constructor should set variables to blank if they are not sent, ie department, jobTitle, etc.
The copy constructor takes on and employee and should use the get methods from the send employee (e) and set up a new employee.
The default constructor should just set everything up to blank.
- Getters & Setters
These should be basic enough that they are setting and returning values. Note that I grouped them together for set/get of each variable. You can code them in any order. There also isn't a setter for hoursWorked variable as we have addHours() and resetHours() that will do the job for us.
- addHours() & addHours(double h)
These are overloaded methods. The addHours() should just add one (1) hour to the hoursWorked variable.
The addHours(double h) should add the variable h to hoursWorked variable. BUT we shouldn't add negative hours, so make sure you handle if the number is negative or not.
- calculateWeeklyPay()
This method should take the payRate and multiply it by the hoursWorked. Then return that value.
- resetHours()
This method sets the hoursWorked to 0
- printEmployee()
This should print the employee object out with headers and the variables. See the format below, where each variable is in square brackets. Note: You should use the currency format object on the payRate variable when printing.
Name: [firstName] [lastName] ID: [employeeNum] Department: [department] Title: [jobTitle] Pay: [payRate] Hours Worked: [hoursWorked]
OUTPUT OF EMPLOYEE.JAVA SHOULD BE LIKE THIS EXAMPLE:
Employee.java -firstName: String -lastName: String -employeeNum : int -department: String -jobTitle: String -hoursWorked: double -payRate: double -currency: NumberFormat > Employee(String fn, String In, int en, String dept, String job, double pr) constructor >> Employee(String fn, String In, int en) > Employee(Employee e) > Employee() +getFirstName() : String +setFirstName(String fn) : void +getLastName() : String +setLastName(String In) : void + getEmployeeNumber() : int +setEmployeeNumber(int en) : void + getDepartment(): String +setDepartment(String dept) : void + getJobTitle() : String +setJobTitle(String job) : void + getHoursWorked(): double +addHours() : void +addHours(double h) : void +resetHours() : void +getPayRate() : double +setPayRate(double pr): void +calculateWeeklyPay() : double +printEmployee() : void Name: Steve Rodgers ID: 3781 Department: Sales Title: Manager Pay: $28.50 Hours Worked: 0.0 Name: Clint Barton ID: 6847 Department: Sales Title: Customer Representative Pay: $15.34 Hours Worked: 0.0 Name: Tony Stark ID: 5749 Department: Service Title: Lead Service Manager Pay: $32.85 Hours Worked: 0.0 Employee Pay: Steve : $142.50 Clint : $184.08 Tony : $32.85 New Employee: Name: Thor Odinson ID: 8623 Department: Service Title: Lead Service Manager Pay: $24.36 Hours Worked: 18.0 Last employee print: Name: Steve Rodgers ID: 3781 Department: Sales Title: Manager Pay: $28.50 Hours Worked: 0.0 Name: Clint Barton ID: 6847 Department: Sales Title: Customer Representative Pay: $15.34 Hours Worked: 0.0 Name: Tony Stark ID: 5749 Department: Service Title: Lead Service Manager Pay: $32.85 Hours Worked: 0.0 Name: Thor Odinson ID: 8623 Department: Service Title: Lead Service Manager Pay: $24.36 Hours Worked: 0.0
Step by Step Solution
There are 3 Steps involved in it
Step: 1
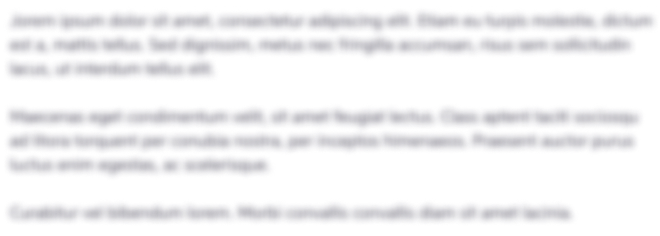
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started