Question
Using Java Product code: public class Product { private int itemNumber; private String name; private int qtyInStock; //quantity in stock private double price; private boolean
Using Java
Product code:
public class Product { private int itemNumber; private String name; private int qtyInStock; //quantity in stock private double price; private boolean active = true; public Product() { } public Product(int number, String name, int qty, double price) { this.itemNumber = number; this.name = name; this.qtyInStock = qty; this.price = price; } public void addToInventory(int quantity) { qtyInStock+=quantity; } public void deductFromInventory(int quantity) { qtyInStock-=quantity; } public int getItemNumber() { return itemNumber; } public void setItemNumber(int itemNumber) { this.itemNumber = itemNumber; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getQtyInStock() { return qtyInStock; } public void setQtyInStock(int qtyInStock) { this.qtyInStock = qtyInStock; } public double getPrice() { return price; } public void setPrice(double price) { this.price = price; } public boolean getActive() { return active; } public void setActive(boolean active) { this.active = active; } public double getInventoryValue() { return price * qtyInStock; } public String toString() { return " Item Number : " + this.itemNumber + " Name : " + this.name + " Quantity in stock: " + this.qtyInStock + " Price : " + this.price + " Stock Value : " + getInventoryValue() + " Product Status : " + (this.active?"Active":"Discontinued"); } }
Overview Create an inventory program that can be used for a range of different products (cds, dvds, software, etc.). Topic(s): Using loops and if statements Arrays of objects Instantiating Objects and Creating Classes Constructors 1. Open the inventory program that was created in Project 6: Creating an inventoryproject 2. You want to be able to mark your products as active or discontinued. If a product is discontinued it means that the remaining stock will be the last of it, and no more orders are to be made. a) Add a Boolean instance field to your Product class called active that has a default value of true. b) Create getter/setter methods for this new field. c) Add the value of this new field to the toString() method so that the output matches the following: Item Number : 3 Name : Marker Quantity in stock: 200 Price : 7.75 Product Status : Active 3. When you run the code, you get back a printed value for active as either true or false. This is not user friendly and would be better if the output stated either Active (true) or Discontinued (false). Add a ternary operator in your toString() method to achieve this. 4. Call the setter from the driver class and set the active value to false for the product6 object before you display the values to screen. Run and test your code. 5. Create a method getInventoryValue() in the Product class that will return the inventory value for each item. Use the product price multiplied by the quantity of stock to calculate the inventory value. Do not use any local variables in this method simply return the value in a single line of code. 6. Update the toString() method in the Product class to include a method call to the getInventoryValue() method that you have just created so that the output is as follows: Item Number : 3 Name : Marker Quantity in stock: 200 Price : 7.75 Stock Value : 1550.0 Product Status : Active CS 111B | Project #7 Spring 2021 _________________________________________________________________________________________________________ Page 2 of 5 7. Modify the ProductTester class. Ask the user to enter the number of products they wish to add. Accept a positive integer for the number of products and handle the value of zero. a) Create a variable named maxSize that can store integers. b) Create a prompt at the beginning of your main method that will instruct the user to enter the required value for the number of products they wish to store: Enter the number of products you would like to add Enter 0 (zero) if you do not wish to add products: c) Use a do while loop so that the program will not continue until a valid positive value is entered. If a value less than zero is entered an error message stating Incorrect Value entered! shouldbe displayed before the user is re-prompted to enter a new value. You should not leave the loop until a value of zero or greater is entered. 8. Modify the ProductTester class to handle multiple products using a single dimensional array if a value greater than zero is entered. a) Create an if statement that will display the message No products required! to the console if the value of maxSize is zero. b) Add an else statement to deal with any value other than zero. c) Create a single one-dimension array named products based on the Product class that will have the number of elements specified by the user. 9. You are now going to populate the array, getting the values from the user for each field in a product object. a) Inside the else statement under where you created the array write a for loop that will iterate through the array from zero to 1 less than maxSize. b) As the last input you received from the user was numeric you will need to add a statement that clears the input buffer as the first line in your for loop. c) Copy the code that you used to get input from the user for all a products fields into the for loop. This includes the name, quantity, price and item number. d) Add a new product object into the array using the index value for the position and the constructer that takes 4 parameters. e) Use a for each loop to display the information for each individual product in the products array. 10. Remove any unnecessary code thats not used in this project. 11. Save your project. CS 111B | Project #7 Spring 2021 _________________________________________________________________________________________________________ Page 3 of 5 Extra Credit (20 pts) You are now going to modify your code so that the main class will not do any processing but simply call static methods when required. a) Create a static method after the end of the main method called addToInventory. This method will not return any values and will accept the products array and the Scanner as parameters. b) Copy the code that adds the values to the array from the main method into the new addToInventory method. c) To resolve the errors that you have in your code move the local variables required (tempNumber, tempName, tempQty, tempPrice) from the main method into the top of the addToInventory method. d) Add a method call in main to the addToInventory() method where you removed the for loop from. e) Run and test your code. f) Create a static method after the end of the main method called displayInventory. This method will not return any values and will accept the products array as a parameter. Remember when you pass an array as a parameter you use the class name as the data type, a set of empty square brackets and then the array name (ClassName[]arrayName). g) Copy the code that displays the array from the main method into the new displayInventory method. h) Where you removed the display code from main, replace it with a method call to the displayInventory method. Remember to include the correct argument list to match the parameter list in the method you are calling. i) Run and test your code. CS 111B | Project #7 Spring 2021 _________________________________________________________________________________________________________ Page 4 of 5 Here is a sample run: Enter the number of products you would like to add. Enter 0 (zero) if you do not wish to add products: 4 Please enter the product name: Pencil Please enter the quantity of stock for this product: 25 Please enter the price for this product: 9.99 Please enter the item number: 1 Please enter the product name: Pen Please enter the quantity of stock for this product: 75 Please enter the price for this product: 8.99 Please enter the item number: 2 Please enter the product name: Marker Please enter the quantity of stock for this product: 200 Please enter the price for this product: 7.75 Please enter the item number: 3 Please enter the product name: Colored Pencil Please enter the quantity of stock for this product: 500 Please enter the price for this product: 9.5 Please enter the item number: 4 Item Number : 1 Name : Pencil Quantity in stock: 25 Price : 9.99 Stock Value : 249.75 Product Status : Active Item Number : 2 Name : Pen Quantity in stock: 75 Price : 8.99 Stock Value : 674.25 Product Status : Active Item Number : 3 Name : Marker Quantity in stock: 200 Price : 7.75 Stock Value : 1550.0 Product Status : Active Item Number : 4 Name : Colored Pencil Quantity in stock: 500 Price : 9.5 Stock Value : 4750.0 Product Status : Active CS 111B | Project #7 Spring 2021
Step by Step Solution
There are 3 Steps involved in it
Step: 1
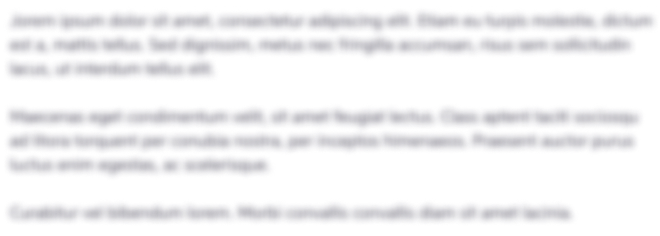
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started