Question
Using java socket programming rewrite the following program to handle multiple clients simultaneously (multi threaded programming) import java.io.*; import java.net.*; public class WelcomeClient { public
Using java socket programming rewrite the following program to handle multiple clients simultaneously (multi threaded programming)
import java.io.*; import java.net.*;
public class WelcomeClient { public static void main(String[] args) throws IOException { if (args.length != 2) { System.err.println( "Usage: java EchoClient
String hostName = args[0]; int portNumber = Integer.parseInt(args[1]);
try ( Socket kkSocket = new Socket(hostName, portNumber); PrintWriter out = new PrintWriter(kkSocket.getOutputStream(), true); BufferedReader in = new BufferedReader( new InputStreamReader(kkSocket.getInputStream())); ) { BufferedReader stdIn = new BufferedReader(new InputStreamReader(System.in)); String fromServer; String fromUser;
while ((fromServer = in.readLine()) != null) { System.out.println("Server: " + fromServer); if (fromServer.equals("Bye.")) break; fromUser = stdIn.readLine(); if (fromUser != null) { System.out.println("Client: " + fromUser); out.println(fromUser); } } } catch (UnknownHostException e) { System.err.println("Don't know about host " + hostName); System.exit(1); } catch (IOException e) { System.err.println("Couldn't get I/O for the connection to " + hostName); System.exit(1); } } }
import java.net.*; import java.io.*;
public class WelcomeServer { public static void main(String[] args) throws IOException { if (args.length != 1) { System.err.println("Usage: java WelcomeServer
int portNumber = Integer.parseInt(args[0]);
try ( ServerSocket serverSocket = new ServerSocket(portNumber); Socket clientSocket = serverSocket.accept(); PrintWriter out = new PrintWriter(clientSocket.getOutputStream(), true); BufferedReader in = new BufferedReader( new InputStreamReader(clientSocket.getInputStream())); ) { String inputLine, outputLine; // Initiate conversation with client WelcomeProtocol wp = new WelcomeProtocol(); outputLine = wp.processInput(null); out.println(outputLine);
while ((inputLine = in.readLine()) != null) { outputLine = wp.processInput(inputLine); out.println(outputLine); if (outputLine.equals("Bye.")) break; } } catch (IOException e) { System.out.println("Exception caught when trying to listen on port " + portNumber + " or listening for a connection"); System.out.println(e.getMessage()); } } }
import java.net.*; import java.io.*;
public class WelcomeProtocol { private static final int WAITING = 0; private static final int SENTWELCOME = 1; private String str; private int state = WAITING; private int currentJoke = 0;
public String processInput(String theInput) { String theOutput = null;
if (state == WAITING) { theOutput = "Welcome!"; state = SENTWELCOME; } else if (state == SENTWELCOME) { if (theInput.equalsIgnoreCase("bye")) { System.exit(1); } else { str = theInput; String str2 = str.replaceAll("\\s",""); int length = str2.length(); String len = Integer.toString(length); theOutput = len; state = SENTWELCOME; } } return theOutput; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
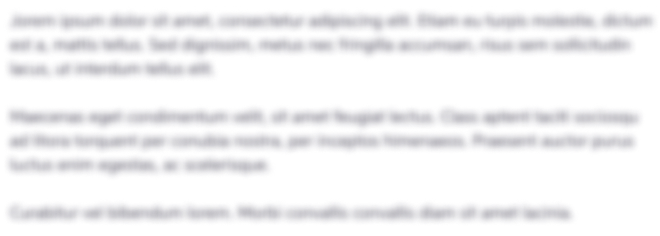
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started