Question
Using Python, estimate the return on investment over a period of time. Task 2: In order to implement this feature, you will have to update
Using Python, estimate the return on investment over a period of time.
Task 2:
In order to implement this feature, you will have to update the given investment calculator algorithm.
The base function to calculate the return on investment from Task 1 can be reutilized here to simplify our task. This feature will take into consideration a 12-month period by default.
To calculate the total amount earned over a period of time, you will have to loop through the n-months period, increase the amount for each period. The other rules from the previous task apply here as well.
For example: If you invest $3 million on the first month, and obtain a return of $93,000 dollars, then the amount to be invested in the second month is $3.093 million.
To summarize:
Loop over a period of 12 months to calculate the total for each period
Return the accumulated estimated value for a period of 12 months
Note that to calculate the accumulated value over a 12-month period do not just multiply the gain of every month by 12 (or n). In this case, the gain of every month is added to the original value, updating the amount to be invested in the subsequent month.
COMPLETED CODE FOR TASK 1:
# global variable: # amount that would trigger the increase on the gain margin in case the invested amount is greater than this value multiplier_amount = 1000000 def calculate_gains(amount_inv=0.0): # base amount gain margin gain_margin = 0.10 total_gains = 0 total_amount = 0 if amount_inv > 1000: # check whether the invested amount is greater than the multiplier amount if amount_inv > multiplier_amount: # +1% each time the amount surpasses increments of 1000000 multiplier_mod = (amount_inv / multiplier_amount) # update the `gain_margin` by the multiplier mod gain_margin = (gain_margin + multiplier_mod) / 100 # calculate the total amount of gains total_gains = round(amount_inv * gain_margin) # calculate the total amount plus the gain margin total_amount = amount_inv + total_gains # return the gains, the full amount and the gain margin return total_gains, total_amount, round(gain_margin, 3), print(calculate_gains(3000000))
OUTLINE FOR TASK 2: Please use this to complete the exercise!
from ReturnOnInvestment1 import calculate_gains def calculate_gains_over_time(amount_inv=0.0, period=12): # call the base `calculate_gains` function from Task 1 to estimate the gains for the first period # calculate the first period before entering the loop # loop through the specified period to calculate the gain of each month # 1 to period-1 because the first period gains is already calculated above # call the function to update the value based on the period inside the loop and the updated amount new_amount = total_amount # update the `new_amount` variable # return the final amount return new_amount print(calculate_gains_over_time(amount_inv=4000000, period=12))
Step by Step Solution
There are 3 Steps involved in it
Step: 1
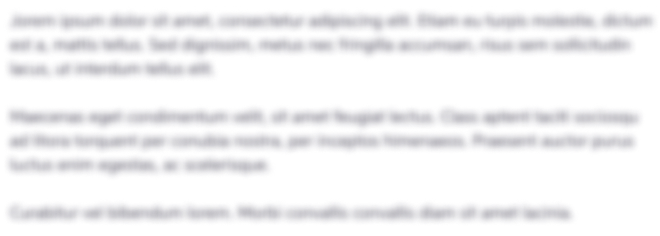
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started