Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Using Swift command line for macOS (open xcode, create a new xcode project, macOS, command line tool) or playground, practice the following exercises: Exercise: Swift
Using Swift command line for macOS (open xcode, create a new xcode project, macOS, command line tool) or playground, practice the following exercises:
Exercise: Swift Classes
- Create a class named Flight with 3 properties: airlineName, number of seats, number of customers with confirmed seats
- Create a custom initializer in the class to set its properties
- Create a method that returns the number of seats available after booking
- Create another class named Boeing as subclass of Flight and initialize it using the custom initializer in the flight class
- Calculate number of seats available in the Boeing flight and print its details
- Now, create one more class called Airbus as subclass of Flight with custom initializer. Override the calculate number of seats method to ignore 2 seats that are only available for cabin crew and rewrite it.
- Also, create a subclass of Airbus that again calculates number of seats using the overridden method above
Exercise: Enum
- Define an enum Month which consists of all the months in a year.
- Next, define a function NumberOfDays() which accepts the month and prints out Number of Days for that month. {Use switch case statement}
Exercise: Structures
- Define a structure, Cube, having 3 parameters length, breadth and height to encapsulate the data
- Create an area function inside struct that calculates the cubes area
- Instantiate cube1 with values of length, breadth and height.
- Assign cube1 to cube2
- Set length of cube2 to some other value
- Calculate and print area of both cubes
Exercise: Protocols
- Define a protocol MastersDegree, define following functions inside this protocol:
- printGPA() - This should print the GPA
- isSmartphonesCourseCompleted() - This should print if the student has completed smartphones course or not
- Now define a class Student which adopt MastersDegree protocol and provide the actual implementation
Exercise: Extensions
- Extending the datatype String add methods: stringLength() and concatenateString (Use another string as Smartphones)
- Next, create a constant named stringExtension of String initializing it to a string of your choice. Use the extended functionality in point 1 and print out the values in the console.
- Complete the code below:
class division {
var number: Int = 0
}
extension Division {
//Define a method to divide 2 numbers and print the result
}
let div = division()
div.dividedBy(number1: 1800, number2: 3)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
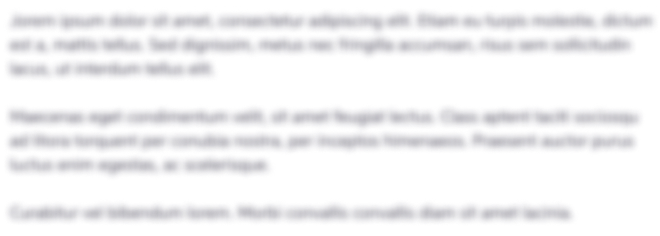
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started