Question
Using the code that FOLLOW the instructions. Implement all of the instruction into the program. It is supposed to convert the postfix expression given from
Using the code that FOLLOW the instructions. Implement all of the instruction into the program. It is supposed to convert the postfix expression given from the function infix_to_postfix and in postfix_to_assembly function it will convert it into assembly language like this example.
EX.:
This results in an assembly program that looks like:
Opcode | Operand | Comment |
LOAD | B | Load in B. |
MULR | C | B * C. |
STOR | TEMP1 | Save results of B * C. |
LOAD | A | Load A. |
ADDR | TEMP1 | Add A to B * C. |
STOR | TEMP2 | Save result. |
LOAD | D | Load D. |
SUBR | E | D - E. |
STOR | TEMP3 | Save result. |
LOAD | TEMP2 | Get A + B * C. |
DIVR | TEMP3 | Divide it by D - E. |
STOR | TEMP4 | Save result, also still in register. |
Your program output should be:
Infix Expression: ( ( AX + ( BY * C ) ) / ( D4 - E ) ) Postfix Expression: AX BY C * + D4 E - / LOAD BY MULR C STOR TEMP1 LOAD AX ADDR TEMP1 STOR TEMP2 LOAD D4 SUBR E STOR TEMP3 LOAD TEMP2 DIVR TEMP3 STOR TEMP4
INSTRUCTIONS: (things to implement)
1) Write a free function to convert the postfix expression into assembly. Put this in utilities.cpp/hpp.
2) You may use your split function (was used in code to show an example of how split function works) to get each token from the postfix expression.
3) Algorithm: Evaluated Postfix. Stack is empty, expr is a valid postfix expression. This algorithm needs to be augmented to solve the postfix to assembly problem.
While (not done with expr) do
t = next token in expr
if t is NOT an operator then
push(t)
else
right = pop stack
left = pop stack
push evaluate(left, t, right)
endif
end while
Top of stack has value
4) You will need to write a special evaluate function to produce the assembly and temporary memory locations. This function will need to output the assembly to an ostream.
5) create a seperate function for the temporary variables use the form TEMPn to save intermediate results. n is in the range of 1 to maxint.
6) Create a main file (modify the postfix.cpp) named assembler.cpp that reads in all the infix expressions in a file given on the command line and writes the expression, the postfix, and the assembly code to a file (optional name on command line otherwise to standard output).
Output format: Sequence of assembly instructions that evaluate the expression. The instruction must start in column 4 and the operand starts in column 10. See the program output example above.
CODE:
You will need to add the functions to utilities.cpp/hpp and implement them in postfix.cpp
---------------------------
utilities.hpp
---------------------------
#ifndef UTILITIES_HPP_ #define UTILITIES_HPP_ #include "stack.hpp" #include #include "../string/string.hpp" String infix_to_postfix(String& infix); #endif
----------------------------------
utilities.cpp
----------------------------------
#include #include #include "utilities.hpp" #include "stack.hpp" String infix_to_postfix(String& infix) { std::vector strings = infix.split(' '); stack stackOfTokens; for(size_t i = 0; i < strings.size(); ++i){ if(strings[i] == ";") break; if(strings[i] == ")"){ String right = stackOfTokens.pop(); String oper = stackOfTokens.pop(); String left = stackOfTokens.pop(); stackOfTokens.push(left + " " + right + " " + oper); } else { if(strings[i]!= "("){ stackOfTokens.push(strings[i]); } } } String result = stackOfTokens.top() + " "; return result; }
------------------------------------------------
postfix.cpp
------------------------------------------------
#include #include #include "utilities.hpp" #include "../string/string.hpp" #include int main(int argc, char* argv[]) { std::ifstream in(argv[1]); std::ofstream out(argv[2]); if(argc < 2 || argc > 4){ std::cout << "Invalid Arguments!" << ' '; } if(!in){ std::cout << "Could not open file: " << argv[1] << ' '; return -1; } char c = '\0'; String s; while(!in.eof()){ while(c != ' ' && c != ' ' && !in.eof()){ s += c; in.get(c); } String output = infix_to_postfix(s); int pos = s.find(";", 0); String strpos = s.substr(0, pos); if(argc == 2){ std::cout << "Infix: " << strpos << ' '; std::cout << "Postfix: " << output << ' '; } else{ out << "Infix: " << strpos << ' '; out << "Postfix: " << output << ' '; } in >> c; s = ""; } out.close(); in.close(); return 0; }
Thank you! The code should be in C++ for it to work.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
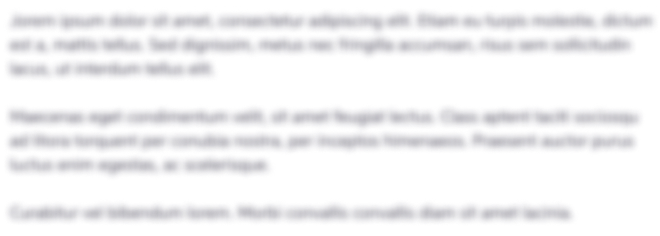
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started